- 1. Micro-Worlds
- 2. Light-Bot in Java
- 3. Jeroos of Santong Island
- 4. Problem Solving and Algorithms
- 5. Creating Jeroo Methods
- 6. Conditionally Executing Actions
- 7. Repeating Actions
- 8. Handling Touch Events
- 9. Adding Text to the Screen

Problem Solving and Algorithms
Learn a basic process for developing a solution to a problem. Nothing in this chapter is unique to using a computer to solve a problem. This process can be used to solve a wide variety of problems, including ones that have nothing to do with computers.
Problems, Solutions, and Tools
I have a problem! I need to thank Aunt Kay for the birthday present she sent me. I could send a thank you note through the mail. I could call her on the telephone. I could send her an email message. I could drive to her house and thank her in person. In fact, there are many ways I could thank her, but that's not the point. The point is that I must decide how I want to solve the problem, and use the appropriate tool to implement (carry out) my plan. The postal service, the telephone, the internet, and my automobile are tools that I can use, but none of these actually solves my problem. In a similar way, a computer does not solve problems, it's just a tool that I can use to implement my plan for solving the problem.
Knowing that Aunt Kay appreciates creative and unusual things, I have decided to hire a singing messenger to deliver my thanks. In this context, the messenger is a tool, but one that needs instructions from me. I have to tell the messenger where Aunt Kay lives, what time I would like the message to be delivered, and what lyrics I want sung. A computer program is similar to my instructions to the messenger.
The story of Aunt Kay uses a familiar context to set the stage for a useful point of view concerning computers and computer programs. The following list summarizes the key aspects of this point of view.
A computer is a tool that can be used to implement a plan for solving a problem.
A computer program is a set of instructions for a computer. These instructions describe the steps that the computer must follow to implement a plan.
An algorithm is a plan for solving a problem.
A person must design an algorithm.
A person must translate an algorithm into a computer program.
This point of view sets the stage for a process that we will use to develop solutions to Jeroo problems. The basic process is important because it can be used to solve a wide variety of problems, including ones where the solution will be written in some other programming language.
An Algorithm Development Process
Every problem solution starts with a plan. That plan is called an algorithm.
There are many ways to write an algorithm. Some are very informal, some are quite formal and mathematical in nature, and some are quite graphical. The instructions for connecting a DVD player to a television are an algorithm. A mathematical formula such as πR 2 is a special case of an algorithm. The form is not particularly important as long as it provides a good way to describe and check the logic of the plan.
The development of an algorithm (a plan) is a key step in solving a problem. Once we have an algorithm, we can translate it into a computer program in some programming language. Our algorithm development process consists of five major steps.
Step 1: Obtain a description of the problem.
Step 2: analyze the problem., step 3: develop a high-level algorithm., step 4: refine the algorithm by adding more detail., step 5: review the algorithm..
This step is much more difficult than it appears. In the following discussion, the word client refers to someone who wants to find a solution to a problem, and the word developer refers to someone who finds a way to solve the problem. The developer must create an algorithm that will solve the client's problem.
The client is responsible for creating a description of the problem, but this is often the weakest part of the process. It's quite common for a problem description to suffer from one or more of the following types of defects: (1) the description relies on unstated assumptions, (2) the description is ambiguous, (3) the description is incomplete, or (4) the description has internal contradictions. These defects are seldom due to carelessness by the client. Instead, they are due to the fact that natural languages (English, French, Korean, etc.) are rather imprecise. Part of the developer's responsibility is to identify defects in the description of a problem, and to work with the client to remedy those defects.
The purpose of this step is to determine both the starting and ending points for solving the problem. This process is analogous to a mathematician determining what is given and what must be proven. A good problem description makes it easier to perform this step.
When determining the starting point, we should start by seeking answers to the following questions:
What data are available?
Where is that data?
What formulas pertain to the problem?
What rules exist for working with the data?
What relationships exist among the data values?
When determining the ending point, we need to describe the characteristics of a solution. In other words, how will we know when we're done? Asking the following questions often helps to determine the ending point.
What new facts will we have?
What items will have changed?
What changes will have been made to those items?
What things will no longer exist?
An algorithm is a plan for solving a problem, but plans come in several levels of detail. It's usually better to start with a high-level algorithm that includes the major part of a solution, but leaves the details until later. We can use an everyday example to demonstrate a high-level algorithm.
Problem: I need a send a birthday card to my brother, Mark.
Analysis: I don't have a card. I prefer to buy a card rather than make one myself.
High-level algorithm:
Go to a store that sells greeting cards Select a card Purchase a card Mail the card
This algorithm is satisfactory for daily use, but it lacks details that would have to be added were a computer to carry out the solution. These details include answers to questions such as the following.
"Which store will I visit?"
"How will I get there: walk, drive, ride my bicycle, take the bus?"
"What kind of card does Mark like: humorous, sentimental, risqué?"
These kinds of details are considered in the next step of our process.
A high-level algorithm shows the major steps that need to be followed to solve a problem. Now we need to add details to these steps, but how much detail should we add? Unfortunately, the answer to this question depends on the situation. We have to consider who (or what) is going to implement the algorithm and how much that person (or thing) already knows how to do. If someone is going to purchase Mark's birthday card on my behalf, my instructions have to be adapted to whether or not that person is familiar with the stores in the community and how well the purchaser known my brother's taste in greeting cards.
When our goal is to develop algorithms that will lead to computer programs, we need to consider the capabilities of the computer and provide enough detail so that someone else could use our algorithm to write a computer program that follows the steps in our algorithm. As with the birthday card problem, we need to adjust the level of detail to match the ability of the programmer. When in doubt, or when you are learning, it is better to have too much detail than to have too little.
Most of our examples will move from a high-level to a detailed algorithm in a single step, but this is not always reasonable. For larger, more complex problems, it is common to go through this process several times, developing intermediate level algorithms as we go. Each time, we add more detail to the previous algorithm, stopping when we see no benefit to further refinement. This technique of gradually working from a high-level to a detailed algorithm is often called stepwise refinement .
The final step is to review the algorithm. What are we looking for? First, we need to work through the algorithm step by step to determine whether or not it will solve the original problem. Once we are satisfied that the algorithm does provide a solution to the problem, we start to look for other things. The following questions are typical of ones that should be asked whenever we review an algorithm. Asking these questions and seeking their answers is a good way to develop skills that can be applied to the next problem.
Does this algorithm solve a very specific problem or does it solve a more general problem ? If it solves a very specific problem, should it be generalized?
For example, an algorithm that computes the area of a circle having radius 5.2 meters (formula π*5.2 2 ) solves a very specific problem, but an algorithm that computes the area of any circle (formula π*R 2 ) solves a more general problem.
Can this algorithm be simplified ?
One formula for computing the perimeter of a rectangle is:
length + width + length + width
A simpler formula would be:
2.0 * ( length + width )
Is this solution similar to the solution to another problem? How are they alike? How are they different?
For example, consider the following two formulae:
Rectangle area = length * width Triangle area = 0.5 * base * height
Similarities: Each computes an area. Each multiplies two measurements.
Differences: Different measurements are used. The triangle formula contains 0.5.
Hypothesis: Perhaps every area formula involves multiplying two measurements.
Example 4.1: Pick and Plant
This section contains an extended example that demonstrates the algorithm development process. To complete the algorithm, we need to know that every Jeroo can hop forward, turn left and right, pick a flower from its current location, and plant a flower at its current location.
Problem Statement (Step 1)
A Jeroo starts at (0, 0) facing East with no flowers in its pouch. There is a flower at location (3, 0). Write a program that directs the Jeroo to pick the flower and plant it at location (3, 2). After planting the flower, the Jeroo should hop one space East and stop. There are no other nets, flowers, or Jeroos on the island.
Analysis of the Problem (Step 2)
The flower is exactly three spaces ahead of the jeroo.
The flower is to be planted exactly two spaces South of its current location.
The Jeroo is to finish facing East one space East of the planted flower.
There are no nets to worry about.
High-level Algorithm (Step 3)
Let's name the Jeroo Bobby. Bobby should do the following:
Get the flower Put the flower Hop East
Detailed Algorithm (Step 4)
Get the flower Hop 3 times Pick the flower Put the flower Turn right Hop 2 times Plant a flower Hop East Turn left Hop once
Review the Algorithm (Step 5)
The high-level algorithm partitioned the problem into three rather easy subproblems. This seems like a good technique.
This algorithm solves a very specific problem because the Jeroo and the flower are in very specific locations.
This algorithm is actually a solution to a slightly more general problem in which the Jeroo starts anywhere, and the flower is 3 spaces directly ahead of the Jeroo.
Java Code for "Pick and Plant"
A good programmer doesn't write a program all at once. Instead, the programmer will write and test the program in a series of builds. Each build adds to the previous one. The high-level algorithm will guide us in this process.
FIRST BUILD
To see this solution in action, create a new Greenfoot4Sofia scenario and use the Edit Palettes Jeroo menu command to make the Jeroo classes visible. Right-click on the Island class and create a new subclass with the name of your choice. This subclass will hold your new code.
The recommended first build contains three things:
The main method (here myProgram() in your island subclass).
Declaration and instantiation of every Jeroo that will be used.
The high-level algorithm in the form of comments.
The instantiation at the beginning of myProgram() places bobby at (0, 0), facing East, with no flowers.
Once the first build is working correctly, we can proceed to the others. In this case, each build will correspond to one step in the high-level algorithm. It may seem like a lot of work to use four builds for such a simple program, but doing so helps establish habits that will become invaluable as the programs become more complex.
SECOND BUILD
This build adds the logic to "get the flower", which in the detailed algorithm (step 4 above) consists of hopping 3 times and then picking the flower. The new code is indicated by comments that wouldn't appear in the original (they are just here to call attention to the additions). The blank lines help show the organization of the logic.
By taking a moment to run the work so far, you can confirm whether or not this step in the planned algorithm works as expected.
THIRD BUILD
This build adds the logic to "put the flower". New code is indicated by the comments that are provided here to mark the additions.
FOURTH BUILD (final)
Example 4.2: replace net with flower.
This section contains a second example that demonstrates the algorithm development process.
There are two Jeroos. One Jeroo starts at (0, 0) facing North with one flower in its pouch. The second starts at (0, 2) facing East with one flower in its pouch. There is a net at location (3, 2). Write a program that directs the first Jeroo to give its flower to the second one. After receiving the flower, the second Jeroo must disable the net, and plant a flower in its place. After planting the flower, the Jeroo must turn and face South. There are no other nets, flowers, or Jeroos on the island.
Jeroo_2 is exactly two spaces behind Jeroo_1.
The only net is exactly three spaces ahead of Jeroo_2.
Each Jeroo has exactly one flower.
Jeroo_2 will have two flowers after receiving one from Jeroo_1. One flower must be used to disable the net. The other flower must be planted at the location of the net, i.e. (3, 2).
Jeroo_1 will finish at (0, 1) facing South.
Jeroo_2 is to finish at (3, 2) facing South.
Each Jeroo will finish with 0 flowers in its pouch. One flower was used to disable the net, and the other was planted.
Let's name the first Jeroo Ann and the second one Andy.
Ann should do the following: Find Andy (but don't collide with him) Give a flower to Andy (he will be straight ahead) After receiving the flower, Andy should do the following: Find the net (but don't hop onto it) Disable the net Plant a flower at the location of the net Face South
Ann should do the following: Find Andy Turn around (either left or right twice) Hop (to location (0, 1)) Give a flower to Andy Give ahead Now Andy should do the following: Find the net Hop twice (to location (2, 2)) Disable the net Toss Plant a flower at the location of the net Hop (to location (3, 2)) Plant a flower Face South Turn right
The high-level algorithm helps manage the details.
This algorithm solves a very specific problem, but the specific locations are not important. The only thing that is important is the starting location of the Jeroos relative to one another and the location of the net relative to the second Jeroo's location and direction.
Java Code for "Replace Net with Flower"
As before, the code should be written incrementally as a series of builds. Four builds will be suitable for this problem. As usual, the first build will contain the main method, the declaration and instantiation of the Jeroo objects, and the high-level algorithm in the form of comments. The second build will have Ann give her flower to Andy. The third build will have Andy locate and disable the net. In the final build, Andy will place the flower and turn East.
This build creates the main method, instantiates the Jeroos, and outlines the high-level algorithm. In this example, the main method would be myProgram() contained within a subclass of Island .
This build adds the logic for Ann to locate Andy and give him a flower.
This build adds the logic for Andy to locate and disable the net.
This build adds the logic for Andy to place a flower at (3, 2) and turn South.
Read the new OECD publication on supporting teachers to use digital tools for developing and assessing 21st century competences.
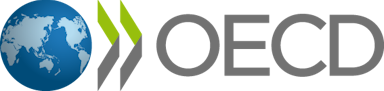
- Applications
- Karel the Turtle
- Betty's Brain
- Game Creator by Cand.li
- Competences
- PILA for Research
Competency framework
Conceptual framework of the PILA Computational Problem Solving module
What is computational problem solving.
‘Computational problem solving’ is the iterative process of developing computational solutions to problems. Computational solutions are expressed as logical sequences of steps (i.e. algorithms), where each step is precisely defined so that it can be expressed in a form that can be executed by a computer. Much of the process of computational problem solving is thus oriented towards finding ways to use the power of computers to design new solutions or execute existing solutions more efficiently.
Using computation to solve problems requires the ability to think in a certain way, which is often referred to as ‘computational thinking’. The term originally referred to the capacity to formulate problems as a defined set of inputs (or rules) producing a defined set of outputs. Today, computational thinking has been expanded to include thinking with many levels of abstractions (e.g. reducing complexity by removing unnecessary information), simplifying problems by decomposing them into parts and identifying repeated patterns, and examining how well a solution scales across problems.
Why is computational problem solving important and useful?
Computers and the technologies they enable play an increasingly central role in jobs and everyday life. Being able to use computers to solve problems is thus an important competence for students to develop in order to thrive in today’s digital world. Even people who do not plan a career in computing can benefit from developing computational problem solving skills because these skills enhance how people understand and solve a wide range of problems beyond computer science.
This skillset can be connected to multiple domains of education, and particularly to subjects like science, technology, engineering or mathematics (STEM) and the social sciences. Computing has revolutionised the practices of science, and the ability to use computational tools to carry out scientific inquiry is quickly becoming a required skillset in the modern scientific landscape. As a consequence, teachers who are tasked with preparing students for careers in these fields must understand how this competence develops and can be nurtured. At school, developing computational problem solving skills should be an interdisciplinary activity that involves creating media and other digital artefacts to design, execute, and communicate solutions, as well as to learn about the social and natural world through the exploration, development and use of computational models.
Is computational problem solving the same as knowing a programming language?
A programming language is an artificial language used to write instructions (i.e. code) that can be executed by a computer. However, writing computer code requires many skills beyond knowing the syntax of a specific programming language. Effective programmers must be able to apply the general practices and concepts involved in computational thinking and problem solving. For example, programmers have to understand the problem at hand, explore how it can be simplified, and identify how it relates to other problems they have already solved. Thus, computational problem solving is a skillset that can be employed in different human endeavours, including programming. When employed in the context of programming, computational problem solving ensures that programmers can use their knowledge of a programming language to solve problems effectively and efficiently.
Students can develop computational problem solving skills without the use of a technical programming language (e.g. JavaScript, Python). In the PILA module, the focus is not on whether students can read or use a certain programming language, but rather on how well students can use computational problem solving skills and practices to solve problems (i.e. to “think” like a computer scientist).
How is computational problem solving assessed in PILA?
Computational problem solving is assessed in PILA by asking students to work through dynamic problems in open-ended digital environments where they have to interpret, design, or debug computer programs (i.e. sequences of code in a visual format). PILA provides ‘learning assessments’, which are assessment experiences that include resources and structured support (i.e. scaffolds) for learning. During these experiences, students iteratively develop programs using various forms of support, such as tutorials, automated feedback, hints and worked examples. The assessments are cumulative, asking students to use what they practiced in earlier tasks when completing successive, more complex tasks.
To ensure that the PILA module focuses on foundational computational problem solving skills and that the material is accessible to all secondary school students no matter their knowledge of programming languages, the module includes an assessment application, ‘Karel World’, that employs an accessible block-based visual programming language. Block-based environments prevent syntax errors while still retaining the concepts and practices that are foundational to programming. These environments work well to introduce novices to programming and help develop their computational problem solving skills, and can be used to generate a wide spectrum of problems from very easy to very hard.
What is assessed in the PILA module on computational problem solving?
Computational problem solving skills.
The module assesses the following set of complementary problem solving skills, which are distinct yet are often used together in order to create effective and efficient solutions to complex problems:
• Decompose problems
Decomposition is the act of breaking down a problem goal into a set of smaller, more manageable sub-goals that can be addressed individually. The sub-goals can be further broken down into more fine-grained sub-goals to reach the granularity necessary for solving the entire problem.
• Recognise and address patterns
Pattern recognition refers to the ability to identify elements that repeat within a problem and can thus be solved through the same operations. Adressing repeating patterns means instructing a computer to iterate given operations until the desired result is achieved. This requires identifying the repeating instructions and defining the conditions governing the duration of the repetition.
• Generalise solutions
Generalisation is the thinking process that results in identifying similarities or common differences across problems to define problem categories. Generalising solution results in producing programs that work across similar problems through the use of ‘abstractions’, such as blocks of organised, reusable sequence(s) of instructions.
• Systematically test and debug
Solving a complex computational problem is an adaptive process that follows iterative cycles of ideation, testing, debugging, and further development. Computational problem solving involves systematically evaluating the state of one’s own work, identifying when and how a given operation requires fixing, and implementing the needed corrections.
Programming concepts
In order to apply these skills to the programming tasks presented in the module, students have to master the below set of programming concepts. These concepts can be isolated but are more often used in concert to solve computational problems:
• Sequences
Sequences are lists of step-by-step instructions that are carried out consecutively and specify the behavior or action that should be produced. In Karel World, for example, students learn to build a sequence of block commands to instruct a turtle to move around the world, avoiding barriers (e.g. walls) and performing certain actions (e.g. pick up or place stones).
• Conditionals
Conditional statements allow a specific set of commands to be carried out only if certain criteria are met. For example, in Karel World, the turtle can be instructed to pick up stones ‘if stones are present’.
To create more concise and efficient instructions, loops can communicate an action or set of actions that are repeated under a certain condition. The repeat command indicates that a given action (i.e. place stone) should be repeated through a real value (i.e. 9 times). A loop could also include a set of commands that repeat as long as a Boolean condition is true, such as ‘while stones are present’.
• Functions
Creating a function helps organise a program by abstracting longer, more complex pieces of code into one single step. By removing repetitive areas of code and assigning higher-level steps, functions make it easier to understand and reason about the various steps of the program, as well as facilitate its use by others. A simple example in Karel World is the function that instructs the turtle to ‘turn around’, which consists of turning left twice.
How is student performance evaluated in the PILA module?
Student performance in the module is evaluated through rubrics. The rubrics are structured in levels, that succinctly describe how students progress in their mastery of the computational problem solving skills and associated concepts. The levels in the rubric (see Table 1) are defined by the complexity of the problems that are presented to the students (simple, relatively complex or complex) and by the behaviours students are expected to exhibit while solving the problem (e.g., using functions, conducting tests). Each problem in the module is mapped to one or more skills (the rows in the rubric) and classified according to its complexity (the columns in the rubric). Solving a problem in the module and performing a set of expected programming operations thus provide evidence that supports the claims about the student presented in the rubric. The more problems at a given cell of the rubric the student solves, the more conclusive is the evidence that the student has reached the level corresponding to that cell.
Please note: the rubric is updated as feedback is received from teachers on the clarity and usefulness of the descriptions.
Table 1 . Rubric for computational problem solving skills
Learning management skills
The performance of students on the PILA module depends not just on their mastery of computational problem solving skills and concepts, but also on their capacity to effectively manage their work in the digital learning environment. The complex tasks included in the module invite students to monitor, adapt and reflect on their understanding and progress. The assessment will capture data on students’ ability to regulate these aspects of their own work and will communicate to teachers the extent to which their students can:
• Use resources
PILA tasks provide resources such as worked examples that students can refer to as they build their own solution. Students use resources effectively when they recognise that they have a knowledge gap or need help after repeated failures and proceed to accessing a learning resource.
• Adapt to feedback
As students work through a PILA assessment, they receive different types of automated feedback (e.g.: ‘not there yet’, ‘error: front is blocked’, ‘try using fewer blocks’). Students who can successfully adapt are able to perform actions that are consistent with the feedback, for example inserting a repetition block in their program after the feedback ‘try using fewer blocks’.
• Evaluate own performance
In the assessment experiences designed by experts in PILA, the final task is a complex, open challenge. Upon completion of this task, students are asked to evaluate their own performance and this self-assessment is compared with their actual performance on the task.
• Stay engaged
The assessment will also collect information on the extent to which students are engaged throughout the assessment experience. Evidence on engagement is collected through questions that are included in a survey at the end of the assessment, and through information on students’ use of time and number of attempts.
Learn about computational problem solving-related learning trajectories:
- Rich, K. M., Strickland, C., Binkowski, T. A., Moran, C., & Franklin, D. (2017). K-8 Learning Trajectories Derived from Research Literature: Sequence, Repetition, Conditionals. Proceedings of the 2017 ACM Conference on International Computing Education Research, 182–190.
- Rich, K. M., Strickland, C., Binkowski, T. A., & Franklin, D. (2019). A K-8 Debugging Learning Trajectory Derived from Research Literature. Proceedings of the 50th ACM Technical Symposium on Computer Science Education, 745–751. https://doi.org/10.1145/3287324.3287396
- Rich, K. M., Binkowski, T. A., Strickland, C., & Franklin, D. (2018). Decomposition: A K-8 Computational Thinking Learning Trajectory. Proceedings of the 2018 ACM Conference on International Computing Education Research - ICER ’18, 124–132. https://doi.org/10.1145/3230977.3230979
Learn about the connection between computational thinking and STEM education:
- Weintrop, D., Beheshti, E., Horn, M., Orton, K., Jona, K., Trouille, L., & Wilensky, U. (2015). Defining Computational Thinking for Mathematics and Science Classrooms. Journal of Science Education and Technology, 25(1), 127–147. doi:10.1007/s10956-015-9581-5
Learn how students apply computational problem solving to Scratch:
- Brennan, K., & Resnick, M. (2012). Using artifact-based interviews to study the development of computational thinking in interactive media design. Paper presented at annual American Educational Research Association meeting, Vancouver, BC, Canada.
Associated Content
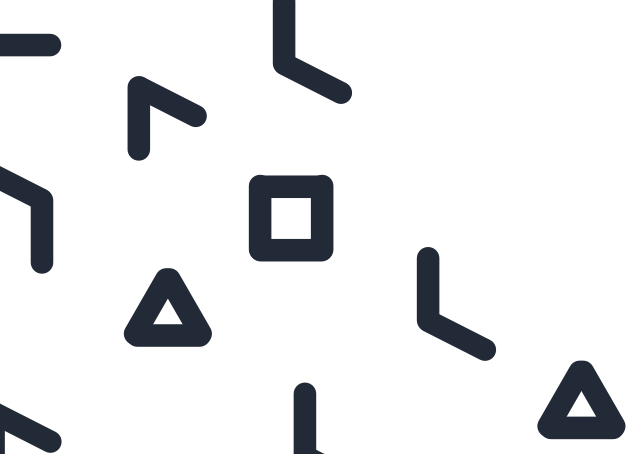
Take a step further
© Organisation for Economic Co-operation and Development
ChatableApps
Mastering the Six Step Problem Solving Model – A Comprehensive Guide for Effective Solutions
Introduction.
Problem-solving skills are essential in both personal and professional lives. Whether you are facing a small issue or a complex challenge, having a structured approach can help you navigate through the problem, analyze it thoroughly, and find effective solutions. One popular and widely-used problem-solving model is the Six Step Problem Solving Model. In this blog post, we will explore the six steps of this model in detail, discussing how each step contributes to solving problems successfully.
Understanding the Six Step Problem Solving Model
The Six Step Problem Solving Model provides a systematic framework for approaching problems. Each step plays a crucial role in understanding, analyzing, and resolving the problem at hand. Let’s delve into each step:
Step 1: Define the problem
The first step is to clearly define the problem. This involves identifying the issue you are facing and understanding its importance. You must have a clear understanding of what needs to be solved before you can move forward. A well-defined problem statement sets the foundation for effective problem-solving.
Step 2: Analyze the problem
Once the problem is defined, it’s time to analyze it. This step involves gathering relevant information and identifying the root causes of the problem. By thoroughly understanding the underlying factors contributing to the problem, you can develop targeted strategies to address them.
Step 3: Generate potential solutions
After analyzing the problem, it’s time to brainstorm potential solutions. This step encourages creative thinking and exploration of different possibilities. Utilizing various brainstorming techniques can help generate a wide range of ideas. Once potential solutions are identified, it’s crucial to evaluate them based on their feasibility and potential impact.
Step 4: Choose the best solution
With a list of potential solutions in hand, it’s important to choose the best one. This step involves utilizing decision-making tools to evaluate each solution’s strengths and weaknesses. Factors such as feasibility, cost, and potential impact should be considered during the decision-making process. By selecting the most effective solution, you increase the likelihood of achieving a successful outcome.
Step 5: Implement the solution
Once a solution has been chosen, it’s time to put it into action. This step requires developing a detailed action plan that outlines the necessary steps to implement the solution effectively. Additionally, assigning responsibilities ensures that everyone involved understands their role in the implementation process. By having a well-structured plan, you can streamline the implementation process and minimize potential setbacks.
Step 6: Evaluate and follow-up
The final step of the problem-solving model is to evaluate the effectiveness of the solution implementation and make necessary adjustments if needed. This step involves assessing whether the solution has produced the desired outcome or if further modifications are required. Regular follow-ups are essential to ensure continuous improvement and address any new challenges that arise.
Applying the Six Step Problem Solving Model in Real-life Scenarios
The Six Step Problem Solving Model can be applied to various real-life situations, both personal and professional. Let’s explore some examples:
Personal problem-solving
When faced with a personal problem, such as managing time effectively or improving relationships, the Six Step Problem Solving Model can be a valuable tool. By defining the problem, analyzing its causes, generating potential solutions, choosing the best one, implementing it, and evaluating the results, individuals can overcome personal challenges and improve their well-being.
Professional problem-solving
In a professional setting, problem-solving skills are vital for success. From addressing customer complaints to optimizing business processes, the Six Step Problem Solving Model provides a structured approach. Applying the model allows for a thorough understanding of the problem, consideration of multiple solutions, informed decision-making, effective implementation, and continuous evaluation for improvement.
Case studies highlighting successful application of the model
Let’s take a look at a few case studies that demonstrate the successful application of the Six Step Problem Solving Model:
- Case Study 1: Resolving Customer Complaints: A customer service team at a retail store implemented the Six Step Problem Solving Model to address a high volume of customer complaints. By defining the problem (long wait times and inadequate product knowledge), analyzing the root causes (staffing issues and lack of training), generating potential solutions (hiring additional staff, providing comprehensive training), choosing the best solution (opting for both solutions), implementing the changes, and evaluating the results, the team successfully reduced customer complaints and improved overall customer satisfaction.
- Case Study 2: Streamlining Manufacturing Processes: A manufacturing company faced inefficiencies in its production line, resulting in increased costs and delays in product delivery. Utilizing the Six Step Problem Solving Model, the team defined the problem (inefficient workflows and bottlenecks), analyzed the root causes (ineffective equipment maintenance and suboptimal process design), generated potential solutions (implementing regular maintenance schedules, reconfiguring layouts), chose the best solution (combination of both solutions), implemented the changes, and continuously evaluated and adjusted strategies. As a result, the company improved productivity, reduced costs, and enhanced customer satisfaction.
Tips and Best Practices for Mastering the Six Step Problem Solving Model
Mastering the Six Step Problem Solving Model requires a combination of critical thinking, effective communication, and appropriate utilization of problem-solving tools and techniques. Here are some tips to enhance your proficiency in using this model:
Developing critical thinking skills
Critical thinking is essential for problem-solving. Sharpening your critical thinking skills allows you to objectively analyze situations, identify patterns, and generate creative and effective solutions. Engage in activities that promote critical thinking, such as puzzles or mind mapping exercises, to enhance this skill.
Enhancing communication and collaboration
Effective communication and collaboration are key to successful problem-solving. Encourage open and constructive dialogue within teams, actively listen to others’ perspectives, and promote idea sharing. By fostering a collaborative environment, you can tap into the collective knowledge and insights of your team, leading to more comprehensive and innovative solutions.
Utilizing problem-solving tools and techniques
There are various problem-solving tools and techniques available that can complement the Six Step Problem Solving Model. Examples include SWOT analysis, Fishbone diagrams, and decision matrices. Familiarize yourself with these tools, and utilize them as appropriate to enhance your problem-solving capabilities.
Advantages and Limitations of the Six Step Problem Solving Model
While the Six Step Problem Solving Model provides a structured approach to problem-solving, it is important to consider its advantages and limitations:
Advantages of using a structured approach
Using a structured approach, such as the Six Step Problem Solving Model, offers several benefits. It provides a clear framework that guides problem-solving activities, ensures thorough analysis of the problem, and encourages systematic decision-making. Additionally, this model allows for continuous evaluation and improvement, enabling individuals and teams to continuously refine their problem-solving skills.
Potential challenges and drawbacks
There are a few potential challenges and drawbacks to be aware of when using the Six Step Problem Solving Model. It may require significant time and effort to complete all six steps, especially for complex problems. Additionally, this model assumes a linear problem-solving process, which may not always align with the dynamic and iterative nature of certain challenges. It is important to adapt the model as needed to accommodate different problem-solving contexts.
The Six Step Problem Solving Model provides individuals and teams with an effective framework for approaching and resolving problems. By defining the problem, analyzing it thoroughly, generating potential solutions, choosing the most suitable option, implementing it effectively, and continuously evaluating and adjusting strategies, you can overcome obstacles and achieve successful outcomes. Mastering this model requires critical thinking, effective communication, and a willingness to learn and improve. Apply the Six Step Problem Solving Model in your personal and professional life and witness the positive impact it can have on problem-solving processes.
Related articles:
- Mastering the Six Step Problem Solving Model – A Step-by-Step Guide for Effective Solutions
- Understanding AI Model Drift – Causes, Challenges, and Solutions
- Mastering the Art of Critical Thinking and Problem Solving – A Comprehensive Definition and Guide
- Mastering the Six-Step Problem Solving Model – A Comprehensive Guide for Success
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.

How it works
For Business
Join Mind Tools
Article • 4 min read
The Problem-Solving Process
Looking at the basic problem-solving process to help keep you on the right track.
By the Mind Tools Content Team
Problem-solving is an important part of planning and decision-making. The process has much in common with the decision-making process, and in the case of complex decisions, can form part of the process itself.
We face and solve problems every day, in a variety of guises and of differing complexity. Some, such as the resolution of a serious complaint, require a significant amount of time, thought and investigation. Others, such as a printer running out of paper, are so quickly resolved they barely register as a problem at all.
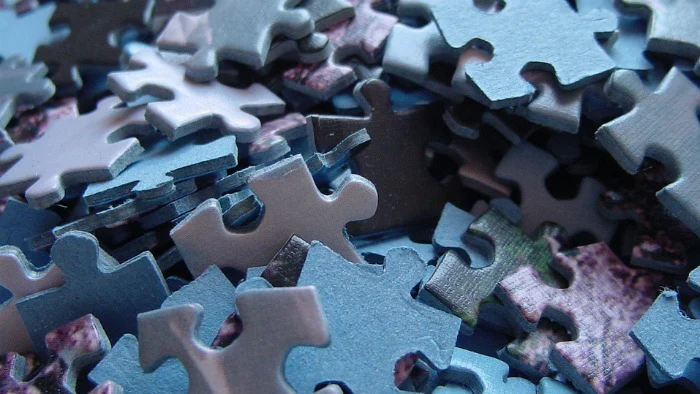
Despite the everyday occurrence of problems, many people lack confidence when it comes to solving them, and as a result may chose to stay with the status quo rather than tackle the issue. Broken down into steps, however, the problem-solving process is very simple. While there are many tools and techniques available to help us solve problems, the outline process remains the same.
The main stages of problem-solving are outlined below, though not all are required for every problem that needs to be solved.
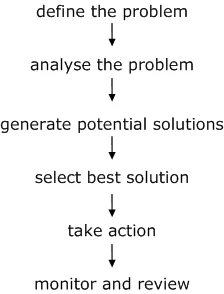
1. Define the Problem
Clarify the problem before trying to solve it. A common mistake with problem-solving is to react to what the problem appears to be, rather than what it actually is. Write down a simple statement of the problem, and then underline the key words. Be certain there are no hidden assumptions in the key words you have underlined. One way of doing this is to use a synonym to replace the key words. For example, ‘We need to encourage higher productivity ’ might become ‘We need to promote superior output ’ which has a different meaning.
2. Analyze the Problem
Ask yourself, and others, the following questions.
- Where is the problem occurring?
- When is it occurring?
- Why is it happening?
Be careful not to jump to ‘who is causing the problem?’. When stressed and faced with a problem it is all too easy to assign blame. This, however, can cause negative feeling and does not help to solve the problem. As an example, if an employee is underperforming, the root of the problem might lie in a number of areas, such as lack of training, workplace bullying or management style. To assign immediate blame to the employee would not therefore resolve the underlying issue.
Once the answers to the where, when and why have been determined, the following questions should also be asked:
- Where can further information be found?
- Is this information correct, up-to-date and unbiased?
- What does this information mean in terms of the available options?
3. Generate Potential Solutions
When generating potential solutions it can be a good idea to have a mixture of ‘right brain’ and ‘left brain’ thinkers. In other words, some people who think laterally and some who think logically. This provides a balance in terms of generating the widest possible variety of solutions while also being realistic about what can be achieved. There are many tools and techniques which can help produce solutions, including thinking about the problem from a number of different perspectives, and brainstorming, where a team or individual write as many possibilities as they can think of to encourage lateral thinking and generate a broad range of potential solutions.
4. Select Best Solution
When selecting the best solution, consider:
- Is this a long-term solution, or a ‘quick fix’?
- Is the solution achievable in terms of available resources and time?
- Are there any risks associated with the chosen solution?
- Could the solution, in itself, lead to other problems?
This stage in particular demonstrates why problem-solving and decision-making are so closely related.
5. Take Action
In order to implement the chosen solution effectively, consider the following:
- What will the situation look like when the problem is resolved?
- What needs to be done to implement the solution? Are there systems or processes that need to be adjusted?
- What will be the success indicators?
- What are the timescales for the implementation? Does the scale of the problem/implementation require a project plan?
- Who is responsible?
Once the answers to all the above questions are written down, they can form the basis of an action plan.
6. Monitor and Review
One of the most important factors in successful problem-solving is continual observation and feedback. Use the success indicators in the action plan to monitor progress on a regular basis. Is everything as expected? Is everything on schedule? Keep an eye on priorities and timelines to prevent them from slipping.
If the indicators are not being met, or if timescales are slipping, consider what can be done. Was the plan realistic? If so, are sufficient resources being made available? Are these resources targeting the correct part of the plan? Or does the plan need to be amended? Regular review and discussion of the action plan is important so small adjustments can be made on a regular basis to help keep everything on track.
Once all the indicators have been met and the problem has been resolved, consider what steps can now be taken to prevent this type of problem recurring? It may be that the chosen solution already prevents a recurrence, however if an interim or partial solution has been chosen it is important not to lose momentum.
Problems, by their very nature, will not always fit neatly into a structured problem-solving process. This process, therefore, is designed as a framework which can be adapted to individual needs and nature.
Join Mind Tools and get access to exclusive content.
This resource is only available to Mind Tools members.
Already a member? Please Login here
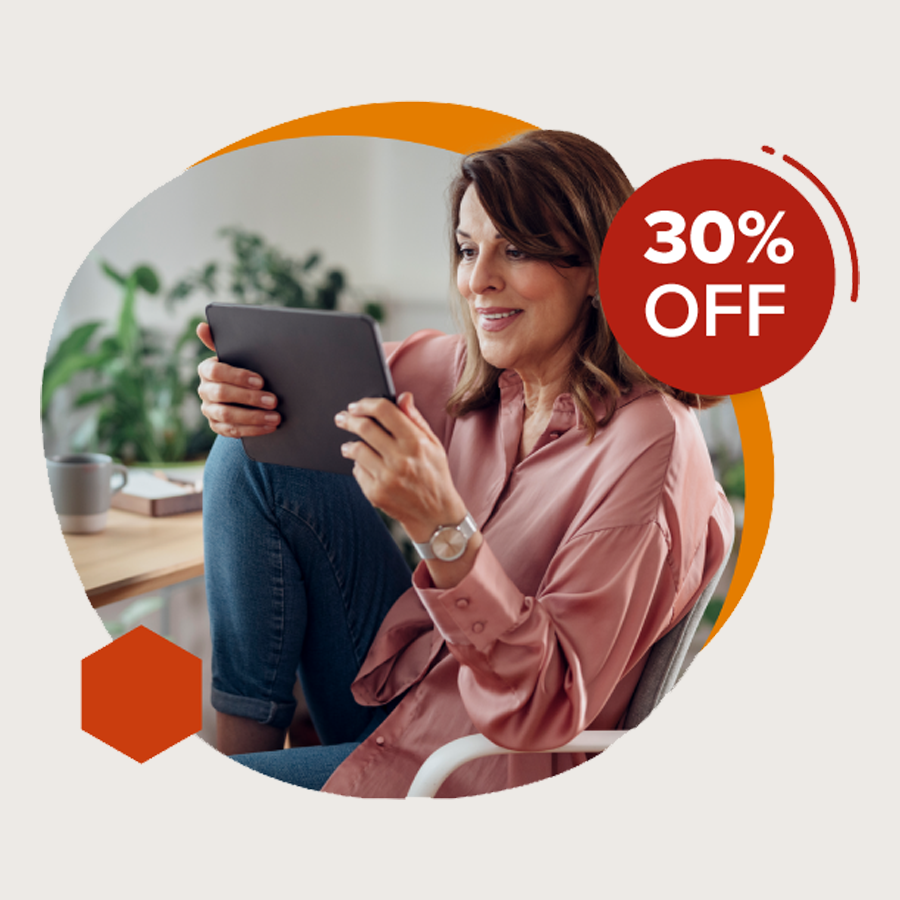
Get 30% off your first year of Mind Tools
Great teams begin with empowered leaders. Our tools and resources offer the support to let you flourish into leadership. Join today!
Sign-up to our newsletter
Subscribing to the Mind Tools newsletter will keep you up-to-date with our latest updates and newest resources.
Subscribe now
Business Skills
Personal Development
Leadership and Management
Member Extras
Most Popular
Newest Releases
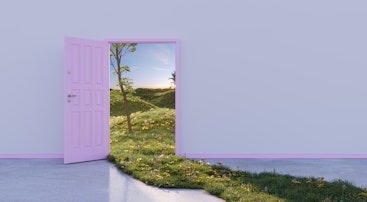
7 Reasons Why Change Fails
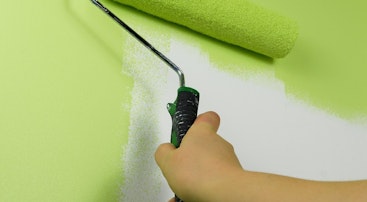
Why Change Can Fail
Mind Tools Store
About Mind Tools Content
Discover something new today
Top tips for tackling problem behavior.
Tips to tackle instances of problem behavior effectively
Defeat Procrastination for Good
Saying "goodbye" to procrastination

How Emotionally Intelligent Are You?
Boosting Your People Skills
Self-Assessment
What's Your Leadership Style?
Learn About the Strengths and Weaknesses of the Way You Like to Lead
Recommended for you
How to manage defensive people.
Lowering Defenses by Building Trust
Business Operations and Process Management
Strategy Tools
Customer Service
Business Ethics and Values
Handling Information and Data
Project Management
Knowledge Management
Self-Development and Goal Setting
Time Management
Presentation Skills
Learning Skills
Career Skills
Communication Skills
Negotiation, Persuasion and Influence
Working With Others
Difficult Conversations
Creativity Tools
Self-Management
Work-Life Balance
Stress Management and Wellbeing
Coaching and Mentoring
Change Management
Team Management
Managing Conflict
Delegation and Empowerment
Performance Management
Leadership Skills
Developing Your Team
Talent Management
Problem Solving
Decision Making
Member Podcast
- Spring 2024
- Spring 2023
- Spring 2022
- Spring 2021
- Spring 2020
- Summer 2019
- Spring 2019
- Summer 2018
- Spring 2018
- Spring 2017
- Spring 2016
- Staff and Contact
- Julia Robinson Math Festival
- Teachers’ Circle
- The Three Stages of the Problem-Solving Cycle
Essentially every problem-solving heuristic in mathematics goes back to George Polya’s How to Solve It ; my approach is no exception. However, this cyclic description might help to keep the process cognitively present.
A few months ago, I produced a video describing this the three stages of the problem-solving cycle: Understand, Strategize, and Implement. That is, we must first understand the problem, then we think of strategies that might help solve the problem, and finally we implement those strategies and see where they lead us. During two decades of observing myself and others in the teaching and learning process, I’ve noticed that the most neglected phase is often the first one—understanding the problem.

The Three Stages Explained
- What am I looking for?
- What is the unknown?
- Do I understand every word and concept in the problem?
- Am I familiar with the units in which measurements are given?
- Is there information that seems missing?
- Is there information that seems superfluous?
- Is the source of information bona fide? (Think about those instances when a friend gives you a puzzle to solve and you suspect there’s something wrong with the way the puzzle is posed.)
- Logical reasoning
- Pattern recognition
- Working backwards
- Adopting a different point of view
- Considering extreme cases
- Solving a simpler analogous problem
- Organizing data
- Making a visual representation
- Accounting for all possibilities
- Intelligent guessing and testing
I have produced videos explaining each one of these strategies individually using problems we have solved at the Chapel Hill Math Circle.
- Implementing : We now implement our strategy or set of strategies. As we progress, we check our reasoning and computations (if any). Many novice problem-solvers make the mistake of “doing something” before understanding (or at least thinking they understand) the problem. For instance, if you ask them “What are you looking for?”, they might not be able to answer. Certainly, it is possible to have an incorrect understanding of the problem, but that is different from not even realizing that we have to understand the problem before we attempt to solve it!
As we implement our strategies, we might not be able to solve the problem, but we might refine our understanding of the problem. As we refine our understanding of the problem, we can refine our strategy. As we refine our strategy and implement a new approach, we get closer to solving the problem, and so on. Of course, even after several iterations of this cycle spanning across hours, days, or even years, one may still not be able to solve a particular problem. That’s part of the enchanting beauty of mathematics.
I invite you to observe your own thinking—and that of your students—as you move along the problem-solving cycle!
[1] Problem-Solving Strategies in Mathematics , Posamentier and Krulik, 2015.
About the author: You may contact Hector Rosario at [email protected].
Share this:
1 response to the three stages of the problem-solving cycle.
Pingback: When (Not) to Give a Hint | Chapel Hill Math Circle
Leave a comment Cancel reply
- Search for:
Recent Posts
- When (Not) to Give a Hint
- Making “Maths” Make Sense
- Problem-Solving Pedagogy
Recent Comments
- September 2016
- Uncategorized
- Entries feed
- Comments feed
- WordPress.com

- Already have a WordPress.com account? Log in now.
- Subscribe Subscribed
- Copy shortlink
- Report this content
- View post in Reader
- Manage subscriptions
- Collapse this bar
- 281.412.7766
- [email protected]
- Learner Dashboard
- GET YOUR CAUSE MAPPING TEMPLATE

- About Cause Mapping®
- What is Root Cause Analysis?
- Cause Mapping® Method
- Cause Mapping® FAQs
- Why ThinkReliability?
- Online Workshops
- On-Demand Training Catalog
- On-Demand Training Subscription
- Company Case Study
- Upcoming Webinars
- Webinar Archives
- Public Workshops
- Private Workshops
- Cause Mapping Certified Facilitator Program
- Our Services
- Facilitation, Consulting, and Coaching
- Root Cause Analysis Program Development
- Work Process Reliability™
- Cause Mapping® Template
- Root Cause Analysis Examples
- Video Library
- Articles and Downloads
- About ThinkReliability
- Client List
- Testimonials
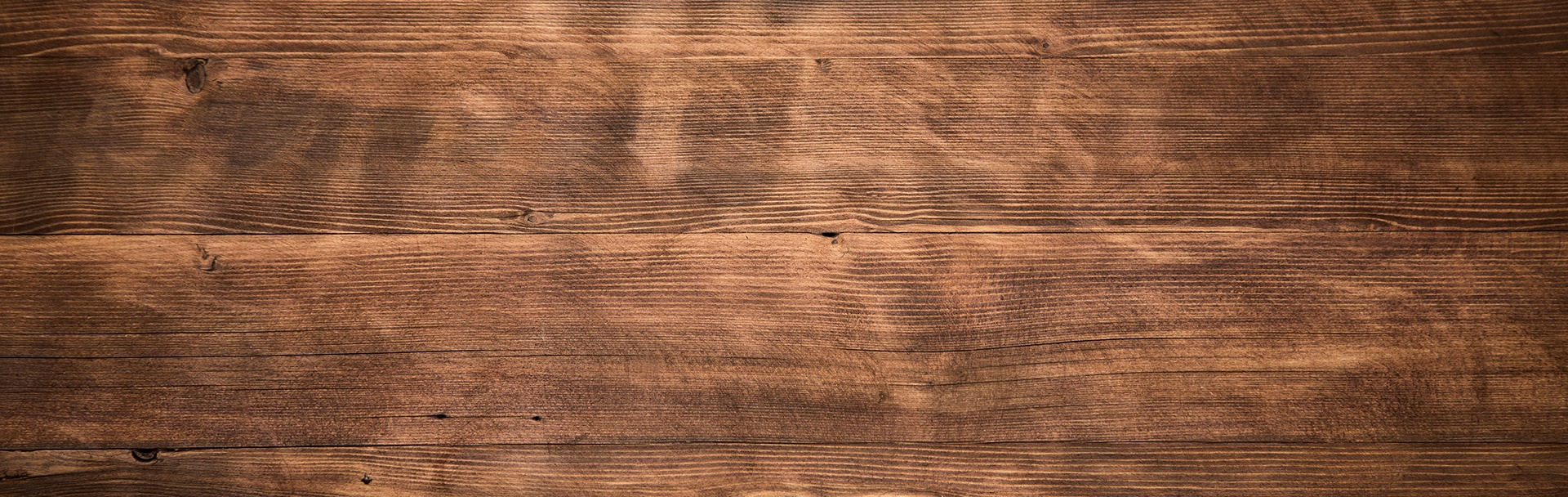
Problem Solving - 3 Basic Steps
Don't complicate it.
Problems can be confusing. Your problem-solving process shouldn’t make them more confusing. With a variety of different tools available, it’s common for people in the same company to use different approaches and different terminology. This makes problem solving problematic. It shouldn’t be.
Some companies use 5Whys , some use fishbone diagrams , and some categorize incidents into generic buckets like " human error " and " procedure not followed ." Some problem-solving methods have six steps, some have eight steps and some have 14 steps. It’s easy to understand how employees get confused.
6-sigma is another widely recognized problem-solving tool. It has five steps with its own acronym, DMAIC: define, measure, analyze, improve and control. The first two steps are for defining and measuring the problem . The third step is the analysis . And the fourth and fifth steps are improve and control, and address solutions .
3 Basic Steps of Problem Solving
As the name suggests, problem solving starts with a problem and ends with solutions. The step in the middle is the analysis. The level of detail within a problem changes based on the magnitude of an issue, but the basic steps of problem solving remain the same regardless of the type of problem:
Step 1. Problem
Step 2. analysis, step 3. solutions.
But these steps are not necessarily what everyone does. Some groups jump directly to solutions after a hasty problem definition. The analysis step is regularly neglected. Individuals and organizations don’t dig into the details that are essential to understand the issue. In the Cause Mapping® method, the point of root cause analysis is to reveal what happened within an incident—to do that digging.
Step 1. Problem
A complete problem definition consists of several different questions:
- What is the problem?
- When did it happen?
- Where did it happen?
- What was the total impact to each of the organization’s overall goals?
These four questions capture what individuals see as a problem, along with the specifics about the setting of the issue (the time and place), and, importantly, the overall consequences to the organization. The traditional approach of writing a problem description as a few sentences doesn’t necessarily capture the information needed for a complete definition. Some organizations see their problem as a single effect, but that doesn’t reflect the nature of an actual issue since different negative outcomes can occur within the same incident. Specific pieces of information are captured within each of the four questions to provide a thorough definition of the problem.
The analysis step provides a clear explanation of an issue by breaking it down into parts. A simple way to organize the details of an incident is to make a timeline . Each piece of the incident in placed in chronological order. A timeline is an effective way to understand what happened and when for an issue.
Ultimately, the objective of problem solving is to turn the negative outcomes defined in step 1 into positive results. To do so, the causes that produced the unwanted outcomes must be identified. These causes provide both the explanation of the issue as well as control points for different solution options. This cause-and-effect approach is the basis of explaining and preventing a problem solving. It’s why cause-and-effect thinking is fundamental for troubleshooting, critical thinking and effective root cause analysis.
Many organizations are under-analyzing their problems because they stop at generic categories like procedure not followed, training less than adequate or management systems . This is a mistake. Learning how to dig a littler further, by asking more Why questions, can reveal significant insight about those chronic problems that people have come to accept as normal operations.
A Cause Map™ diagram provides a way for frontline personnel, technical leads and managers to communicate the details of an issue objectively, accurately and thoroughly. A cause-and-effect analysis can begin as a single, linear path that can be expanded into as much detail as needed to fully understand the issue.
Solutions are specific actions that control specific causes to produce specific outcomes. Both short-term and long-term solutions can be identified from a clear and accurate analysis. It is also important for people to understand that every cause doesn’t need to be solved. Most people believe that 15 causes require 15 solutions. That is not true. Changing just one cause along a causal path breaks that chain of events. Providing solutions on more than one causal path provides additional layers of protection to further reduce the risk of a similar issue occurring in the future.
The Basics of Problem Solving Don't Change
These three steps of problem solving can be applied consistently across an organization from frontline troubleshooters to the executives. First principles should be the foundation of a company’s problem-solving culture. Overlooking these basics erodes critical thinking. Even though the fundamentals of cause-and-effect don’t change, organizations and individuals continue to find special adjectives, algorithms and jargon appealing. Teaching too many tools and using contrived terms such as “true root causal factors” is a symptom of ignoring lean principles. Don’t do that which is unnecessary.
Your problems may be complex, but your problem-solving process should be clear and simple. A scientific approach that objectively explains what happened and why (cause and effect) is sound. It’s the basis for understanding and solving a problem – any problem. It works on the farm, in the power plant, at the manufacturing company and at an airline. It works for the cancer researcher and for the auto mechanic. It also works the same way for safety incidents, production losses and equipment failures. Cause and effect doesn’t change. Just test it.
If you’re interested in seeing one of your problems dissected as a Cause Map diagram, send us an email or call the ThinkReliability office. We’ll arrange a call to step through your issue. You can also learn more about improving the way your organization investigates and prevents problems through one of our upcoming online webinars, short courses or workshops .
Want to learn more? Watch our 28-minute video on problem-solving basics.
Share This Post With A Friend

Similar Posts
Other resources.
- Root Cause Analysis blog
- Patient Safety blog
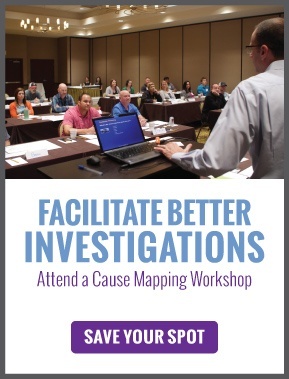
READ BY - - - - - - - - - -

Other Resources - - - - - - - - - -

Sign Up For Our eNewsletter

Snapsolve any problem by taking a picture. Try it in the Numerade app?
A descriptive phase model of problem-solving processes
- Original Paper
- Open access
- Published: 09 March 2021
- Volume 53 , pages 737–752, ( 2021 )
Cite this article
You have full access to this open access article
- Benjamin Rott ORCID: orcid.org/0000-0002-8113-1584 1 ,
- Birte Specht 2 &
- Christine Knipping 3
7414 Accesses
20 Citations
Explore all metrics
This article has been updated
Complementary to existing normative models, in this paper we suggest a descriptive phase model of problem solving. Real, not ideal, problem-solving processes contain errors, detours, and cycles, and they do not follow a predetermined sequence, as is presumed in normative models. To represent and emphasize the non-linearity of empirical processes, a descriptive model seemed essential. The juxtaposition of models from the literature and our empirical analyses enabled us to generate such a descriptive model of problem-solving processes. For the generation of our model, we reflected on the following questions: (1) Which elements of existing models for problem-solving processes can be used for a descriptive model? (2) Can the model be used to describe and discriminate different types of processes? Our descriptive model allows one not only to capture the idiosyncratic sequencing of real problem-solving processes, but simultaneously to compare different processes, by means of accumulation. In particular, our model allows discrimination between problem-solving and routine processes. Also, successful and unsuccessful problem-solving processes as well as processes in paper-and-pencil versus dynamic-geometry environments can be characterised and compared with our model.
Similar content being viewed by others
Thematic Analysis
Qualitative Content Analysis: Theoretical Background and Procedures
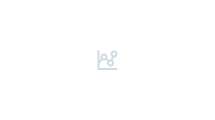
Decision Making: a Theoretical Review
Avoid common mistakes on your manuscript.
1 Introduction
Problem solving (PS)—in the sense of working on non-routine tasks for which the solver knows no previously learned scheme or algorithm designed to solve them (cf. Schoenfeld, 1985 , 1992b )—is an important aspect of doing mathematics (Halmos, 1980 ) as well as learning and teaching mathematics (Liljedahl et al. 2016 ). As one of several reasons, PS is used as a means to help students learn how to think mathematically (Schoenfeld, 1992b ). Hence, PS is part of mathematics curricula in almost all countries (e.g., KMK, 2004 ; NCTM, 2000 , 2014 ). Accordingly, PS has been a focus of interest of researchers for several decades, Pólya ( 1945 ) being one of the most prominent scholars interested in this activity.
Problem-solving processes (PS processes) can be characterised by their inner or their outer structure (Philipp, 2013 , pp. 39–40). The inner structure refers to (meta)cognitive processes such as heuristics, checks, or beliefs, whereas the outer structure refers to observable actions that can be characterised in phases like ‘understanding the problem’ or ‘devising a plan’, as well as the chronological sequence of such phases in a PS process. Our focus in this paper is on the outer structure, as it is directly accessible to teachers and researchers via observation.
In the research literature, there are various characterisations of PS processes. However, almost all of the existing models are normative , which means they represent idealised processes. They characterise PS processes according to distinct phases, in a predetermined sequence, which is why they are sometimes called ‘prescriptive’ instead of normative. These phases and their sequencing have been formulated as a norm for PS processes. Normative models are generally used as a pedagogical tool to guide students’ PS processes and to help them to become better problem solvers. The normative models in current research have mostly been derived from theoretical considerations. Nevertheless, real PS processes look different; they contain errors, detours, and cycles, and they do not follow a predetermined sequence. Actual processes like these are not considered in normative models. Accordingly, there are almost no models that guide teachers and researchers in observing, understanding, and analysing PS processes in their ‘non-smooth’ occurrences (cf. Fernandez et al. 1994 ; Rott, 2014 ). Our aim in this paper, therefore, is to address this research gap by suggesting a descriptive model.
A descriptive model enables not only the representation of real PS processes, but also reveals additional potential for analyses. Our model allows one systematically to compare several PS processes simultaneously by means of accumulation, which is an approach that to our knowledge has not been proposed before in the mathematics education community. In Sect. 6 , we show how this approach can be used to reveal ‘bumps and bruises’ of real students’ PS processes to illustrate the practical value of our descriptive model (Sect. 5.3 , Fig. 5 ). We show how our model allows one to discriminate problem-solving processes from routine processes when students work on tasks. We illustrate how differences between successful and unsuccessful processes can be identified using our model. We also reveal how students’ PS processes, working in a paper-and-pencil environment compared to working in a digital (dynamic geometry) environment , can be characterised and compared by means of our model.
Our descriptive model is based on intertwining theoretical considerations, in the form of a review of existing models, as well as on a video-study researching the processes shown by mathematics pre-service teachers working on geometrical problems.
2 Theoretical background
In this section, we first describe and compare aspects of existing models of PS processes (which are mostly normative) to characterize their potential and their limitations for analysing students’ PS processes (2.1). We then discuss why looking specifically at students’ PS processes in geometry and in dynamic geometry contexts is of particular value for developing a descriptive model of PS processes (2.2).
2.1 Models of problem-solving processes
Looking at models from mathematics, mathematics education, and psychology that describe the progression of PS processes, we find phase models, evolved by authors observing their own PS processes or those of people with whom the authors are familiar. So, the vast majority of existing PS process models are not based on ‘uninvolved’ empirical data (e.g., videotaped PS processes of students); they were actually not designed for the analysis of empirical data or to describe externally observed processes, which emphasises the need for a descriptive model.
2.1.1 Classic models of problem-solving processes
Two ‘basic types’ of phase models for PS processes have evolved in psychology and mathematics education. Any further models can be assigned to one or the other of these basic types: (1) the intuitive or creative type and (2) the logical type (Neuhaus, 2002 ).
Intuitive or creative models of PS processes originate in Poincaré’s ( 1908 ) introspective reflection on his own PS processes. Building on his thoughts, the mathematician Hadamard ( 1945 ) and the psychologist Wallas ( 1926 ) described PS processes with a particular focus on subconscious activities. Their ideas are most often summarised in a four-phase model: (i) After working on a difficult problem for some time and not finding a solution ( preparation ), (ii) the problem solver does and thinks of different things ( incubation ). (iii) After some more time—hours, or even weeks—suddenly, a genius idea appears ( illumination ), providing a solution or at least a significant step towards a solution of the problem; (iv) this idea has to be checked for correctness ( verification ).
So-called logical models of PS processes were introduced by Dewey ( 1910 ), describing five phases: (i) encountering a problem ( suggestions ), (ii) specifying the nature of the problem ( intellectualization ), (iii) approaching possible solutions ( the guiding idea and hypothesis ), (iv) developing logical consequences of the approach ( reasoning (in the narrower sense) ), and (v) accepting or rejecting the idea by experiments ( testing the hypothesis by action ). Unlike in Wallas’ model, there are no subconscious activities described in Dewey’s model. Pólya’s ( 1945 ) famous four-phase model—(i) understanding the problem , (ii) devising a plan , (iii) carrying out the plan , and (iv) looking back —manifests, according to Neuhaus ( 2002 ), references to Dewey’s work.
Research in mathematics education mainly focuses on logical models for describing PS processes, following Pólya or more recent variants of his model (see below). This is due to the fact that PS processes of the intuitive or creative type might take hours, days, or even weeks to allow for genuine incubation phases, and PS activities in the context of schooling and university teaching are mostly shorter and more contained. Therefore, we focus on logical models. In the following, we compare prominent PS process phase models that emerged in the last decades (see Fig. 1 ).
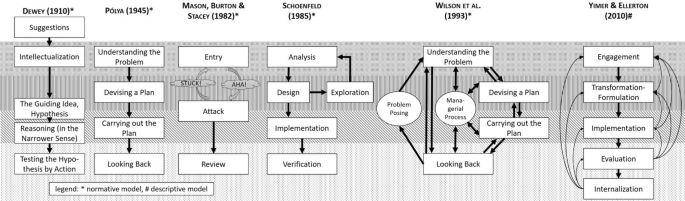
Different phase models of problem-solving processes
2.1.2 Recent models of problem-solving processes
In Fig. 1 , different models are presented (for more details see the appendix). These build on and alter distinct aspects of Pólya’s model, especially envisioned phases and possible transitions between these phases. They mark this distinction by using different terminology for these nuanced differences in the phases. The models by Mason et al. ( 1982 ), Schoenfeld ( 1985 , Chapter 4), and Wilson et al. ( 1993 ; Fernandez et al. 1994 ) are normative; they are mostly used for teaching purposes, that is, to instruct students in becoming better problem solvers. Compared to actual PS processes, these models comprise simplifications; looking at and analysing students’ PS processes requires models which are suited to portray these uneven and cragged processes.
In several studies, actual PS processes are analysed; however, only a few of these studies use any of these normative models that describe the outer structure of PS processes. Even fewer studies present a descriptive model as part of their results. Some of the rare studies that attempt to derive such a model are presented in more detail in the appendix; their essential ideas are presented below (Artzt & Armour-Thomas, 1992 ; Jacinto & Carreira, 2017 ; Yimer & Ellerton, 2010 ).
2.1.3 Comparing models of problem-solving processes
In this section, we compare the previously mentioned as well as additional phase models with foci on (a) the different types of phases and (b) linearity or non-linearity of the portrayed PS processes. Figure 1 illustrates similarities and differences in these models, starting with those of Dewey ( 1910 ) and Pólya ( 1945 ) as these authors were the first to suggest such models. Schoenfeld ( 1985 ) and Mason et al. ( 1982 ) introduced this discussion to the mathematics education community, referring back to ideas of Pólya. Then, we discuss those of Wilson et al. ( 1993 ), and Yimer and Ellerton ( 2010 ), as examples of more recent models in mathematics education.
Different types of phases
The presented models comprise three, four, or more different phases. However, we do not think that this number is important per se; instead, it is interesting to see which activities are encompassed in these phases of the different models and in the extent and manner in which they follow Pólya’s formulation, adopt it, or go beyond his ideas. In Fig. 1 , we indicated Pólya’s phases with differently patterned layers in the background.
Dewey’s ( 1910 ) model starts with a phase (named “suggestions”) in which the problem solvers come into contact with a problem without already analysing or working on it. Such a phase is seldom found in phase models in the context of mathematics education. In mathematics though this phase at the beginning is typical and important, as Dewey already pointed out. In the context of teaching, on the other hand, PS mostly starts with a task handed to the students by their teachers. Analysing and working on the problem is expected right from the beginning; this is part of the nature of the provided task. So, in educational research the phase of “suggestions” is rarely mentioned, as it normally does not occur in students’ PS processes.
“Understanding the problem”, Pólya’s ( 1945 ) first phase, is comparable to the second phase (“intellectualization”) of Dewey’s model. In this phase, problem solvers are meant to make sense of the given problem and its conditions. Such a phase is used in all models, though often labelled slightly differently (see Fig. 1 for a juxtaposition). Artzt and Armour-Thomas, ( 1992 ) facing the empirical data of their study, differentiated this phase of “understanding the problem” into a first step, where students are meant to apprehend the task (“understanding”), and a second step, where students are actually expected to comprehend the problem (“analysing”); a similar differentiation is presented by Jacinto and Carreira ( 2017 ) into “grasping, noticing” and “interpreting” a problem.
The next two phases incorporate the actual work on the problem. Pólya describes these phases as “devising” and “carrying out a plan”. Especially the planning phase encompasses many different activities, such as looking for similar problems or generalizations. These two phases are also integral parts of the models by Wilson et al. ( 1993 ), and Yimer and Ellerton ( 2010 ) (see Fig. 1 ), or Jacinto and Carreira ( 2017 , there called “plan” and “create”). Mason et al. ( 1982 ) chose to combine both phases, calling this combined phase “attack”. According to their educational and research experience, they noted that both phases cannot be distinguished in most cases; therefore, a differentiation would not be helpful for learning PS and describing PS processes. Schoenfeld ( 1985 ), on the contrary, further differentiated those phases by splitting Pólya’s second phase into a structured “planning” (or “design”) phase and an unstructured “exploration” phase. When “planning”, one might adopt a known procedure or try a combination of known procedures in a new problem context. However, when known procedures do not help, working heuristically (e.g., looking at examples, counter-examples, or extreme cases) might be a way to approach the given problem in “exploration” (Schoenfeld, 1985 , p. 106). According to Schoenfeld, exploration is the “heuristic heart” of PS processes.
The last phase in Pólya’s model is “looking back”, the moment when a solution should be checked, other approaches should be explored, and methods used should be reflected upon. This phase is also present in other models (see Fig. 1 ). In their empirical approach, Yimer and Ellerton ( 2010 ), for example, differentiated this phase into two steps, namely, “evaluation” (i.e., checking the results), which refers to looking back on the recently solved problem, and “internalization” (i.e., reflecting the solution and the methods used), which focuses on what has been learnt by solving this problem and looks forward to using this recent experience for solving future problems. Jacinto and Carreira ( 2017 ) used the same “verifying” phase as Pólya, but added a “disseminating” phase for presenting solutions, as their final phase.
Other researchers (see the appendix) came to insights similar to those of these researchers, using slightly different terminologies when describing these phases or combinations of these phases.
Sequence of phases: linear or non-linear problem-solving processes
Other important aspects are transitions from one phase to another, and how such transitions occur. The graphical representations of different models in Fig. 1 not only indicate slightly different phases (and distinct labels for these phases), but also illustrate different understandings of how these phases are related and sequenced.
There are strictly linear models like Pólya’s ( 1945 ), which outline four phases that should be passed through when solving a problem, in the given order. Of course, Pólya as a mathematician knew that PS processes are not always linear; in his normative model, however, he proposed such a stepwise procedure, which has often been criticised (cf. Wilson et al. 1993 ). Mason et al. ( 1982 ) and Schoenfeld ( 1985 ) discarded this strict linearity, including forward and backward steps between analysing, planning, and exploring (or attacking, respectively) a problem. Thereafter, PS processes linearly proceed towards the looking back equivalents of their models. Wilson et al. ( 1993 ) presented a fully “dynamic, cyclic interpretation of Polya’s stages” (p. 60) and included forward and backward steps between all phases, even after “looking back”. The same is true for Yimer and Ellerton ( 2010 ), who included transitions between all phases in their model.
As we illustrate later, transitions from one phase to another reflect also characteristic features of routine and non-routine processes in general, and can be also distinctive for students’ PS processes in traditional paper-and-pencil environments compared to Dynamic Geometry Software (DGS) contexts. Our descriptive model of PS processes, which we present in Sect. 5 , also evolved by comparative analyses of students’ PS processes in both learning contexts. Thus, we comment briefly in 2.2 on what existing research has found in this respect so far.
2.2 Problem solving in geometry and dynamic geometry software
Overall, geometry is especially suited for learning mathematical PS in general and PS strategies or heuristics in particular (see Schoenfeld, 1985 ). Notably, many geometric problems can be illustrated in models, sketches, and drawings, or can be solved looking at special cases or working backwards (ibid.). Additionally, the objects of action (at least in Euclidean geometry) and the permitted actions (e.g., constructions with compasses and ruler) are easy to understand. Therefore, in our empirical study (see Sect. 4 ), we opted for PS processes in geometry contexts, knowing that other contexts could be equally fruitful.
One particular tool to support learning and working in the context of geometry, since the 1980s, is DGS, which is characterised by three features, namely, dragmode, macro-constructions, and locus of points (Sträßer, 2002 ). With these features, DGS can be used not only for verification purposes, but also for guided discoveries as well as working heuristically (e.g., Jacinto & Carreira, 2017 ). However, as Gawlick ( 2002 ) pointed out, to profit from such an environment, students—especially low achievers—need some time to get accustomed to handling the software. Comparing DGS and paper-and-pencil environments, Koyuncu et al. ( 2015 ) observed that in a study with two pre-service teachers, “[b]oth participants had a tendency toward using algebraic solutions in the [paper-and-pencil based] environment, whereas they used geometric solutions in the [DGS based] environment.” (p. 857 f.). These potential differences between PS processes in paper-and-pencil versus DGS environments are interesting for research and practice. Therefore, we compared students’ PS processes in these two environments in our empirical study.
3 Research questions
With regard to research on PS processes, it is striking that there is only a small number of studies, often with a low number of participants, that present and apply a descriptive model of PS processes. Further, the identified models are not suited for comparing PS processes across groups of students, but can only describe cases. Last but not least, in most empirical studies, the selection of phases that are included, and the assumption of (non-)linearity, are not discussed and/or justified. In all these respects, we see a research gap. Contributing to filling this gap was one of the motivations for the study presented here. Based on the existing research literature, we formulated two main research questions:
What elements of the already discussed PS process models can be used for a descriptive model? In particular, what is necessary so that such a descriptive model enables
a recognition of types of phases and an identification of phases in actual PS processes as well as
an identification of the sequence (i.e., the order, linear or non-linear) of phases and transitions between phases?
Can the model be used to describe and discriminate among different types of PS processes, for example
routine and non-routine processes,
successful and not successful processes, or
paper-and-pencil vs. DGS processes?
These questions guided our study and the motivation for developing a descriptive model of PS processes. Next, we present the methodology, before we discuss results of our empirical study and present our model.
4 Methodology
In a previous empirical study, we looked at PS processes of pre-service teacher students in geometry contexts. The data in this study were enormously rich and challenged us in their analyses in many ways. Existing PS models did not allow us to harvest fully this rich data corpus and we realised that with respect to our empirical data, we needed a descriptive model. So we formulated the research questions listed above in order to explore the potential and necessary extensions of the existing normative PS process models. We changed our perspective and focused on the development of an empirically grounded theoretical model. We required an approach that would allow us to mine the data of our empirical study and to provide a conceptualisation that could be helpful for further research on students’ problem-solving processes. The methodological approach we used is described in the following.
4.1 Our empirical study
About 250 pre-service teacher students attended a course on Elementary Geometry , which was conceived and conducted by the third author at a university in Northern Germany. The course lasted for one semester (14 weeks); each week, a two-hour lecture for all students as well as eight 2-h tutorials for up to 30 students each, supervised by tutors (advanced students), took place. Four tutorials (U1, Ulap2, U3, and Ulap4) were involved in this study: in U1 and U3 the students worked in a paper-and-pencil environment, in Ulap2 and Ulap4 the students used laptop computers to work in a DGS environment. (The abbreviations consist of U, the first letter ‘Uebung’, German for tutorial, with an added ‘lap’ for groups which used laptop computers as well as an individual number.) Students worked on weekly exercises, which were discussed in the tutorials. In addition, over the course of the semester, in groups of three or four, the students worked on five geometric problems in the tutorials (approx. 45 min for each problem), accompanied by as little tutor help as possible. In this paper, we focus on these five problems. See the appendix for additional information regarding the organisation of our study.
The five problems were chosen so that students had the opportunity to solve a variety of non-routine tasks, which at the same time did not require too much advanced knowledge that students might not have.
For each of the five problems, two groups from each of the four tutorials were observed. Each problem was therefore worked on by four groups with and four groups without DGS (minus some data loss because of students missing tutorials or technical difficulties). The collected data were videos of the groups working on these problems ( processes ), notes by the students ( products ), as well as observers’ notes. Overall, 33 processes (15 from paper-and-pencil as well as 18 from DGS groups) from all five problems, with a combined duration of 25 h, were analysed. For space reasons, we cannot discuss all five problems in detail here. Instead, we present three of the five problems here; the other two can be found in the appendix.
4.1.1 The problems
Regarding the ‘Shortest Detour’ (Fig. 2 , top), as long as A and B are on different sides of the straight line, a line segment from A to B is the shortest way. When A and B are on the same side of g , an easy (not the only) way to solve this problem is by reflecting one of the points, e.g. A , on g and then constructing the line segment from the reflection of A to B , as reflections preserve lengths.
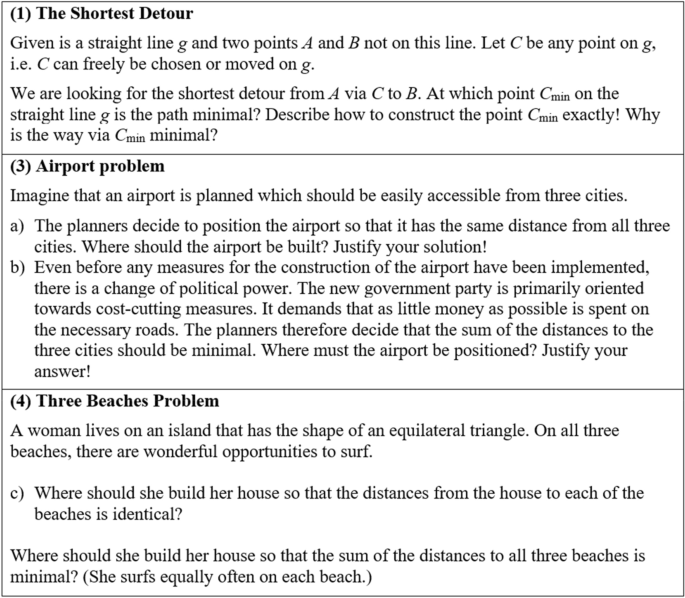
Three of the five problems used in our study
Part a) of the ‘Three Beaches’ problem (Fig. 2 , bottom), finding the incircle of an equilateral triangle, should be a routine-procedure as this topic had been discussed in the lecture. Students working on part b) of this problem needed to realize that in an equilateral triangle, all points have the same sum of distances to the sides (Viviani’s problem). This could be justified by showing that the three perpendiculars of a point to a side in such a triangle add up to the height of this triangle, for example by geometrical addition or by calculating areas.
Like Problem (4), Problem (3) (Fig. 2 , middle) contained an a)-part which is a routine task—finding the circumcircle of a (non-regular) triangle—and a b)-part that constitutes a problem for the students.
These tasks were chosen because they actually represented problems for our students, and expected PS processes appeared neither too long nor too short for a reasonable workload by students and for our analyses. Further, the problems covered the content of the accompanying lecture, and the problems could be solved both with and without DGS.
Differences between working with and without DGS: With DGS many examples can be generated quickly, so that an overview of the situation and the solution can be obtained in a short time. For the justifications, however, with and without DGS, students had to reflect, think, and reason to find appropriate arguments.
4.2 Framework for the analysis of the empirical data
For the analyses of our students’ PS processes, we used the protocol analysis framework by Schoenfeld ( 1985 , Chapter 9) with adaptations and operationalizations by Rott ( 2014 ), following two phases of coding.
Process coding: With his framework, Schoenfeld ( 1985 ) intended to “identify major turning points in a solution. This is done by parsing a [PS process] into macroscopic chunks called episodes” (p. 314). An episode is “a period of time during which an individual or a problem-solving group is engaged in one large task […] or a closely related body of tasks in the service of the same goal […]” (p. 292). Please note, the term “episode” refers to coded process data, whereas “phase” refers to parts of PS models. Schoenfeld (p. 296) continued: “Once a protocol has been parsed into episodes, each episode is characterized” using one of six categories (see also Schoenfeld, 1992a , p. 189):
Reading or rereading the problem.
Analysing the problem (in a coherent and structured way).
Exploring aspects of the problem (in a much less structured way than in Analysis).
Planning all or part of a solution.
Implementing a plan.
Verifying a solution.
According to Schoenfeld ( 1985 ), Planning-Implementation can be coded simultaneously.
The idea of episodes as macroscopic chunks implies a certain length, thus individual statements do not comprise an episode; for example, quickly checking an interim result is not coded as a verification episode. Also, PS processes are coded by watching videos, not by reading transcripts (Schoenfeld, 1992a ).
Schoenfeld’s framework was chosen to answer our first research question, for two reasons. (i) The episode types he proposed cover a lot of the variability of phases also identified by us (see Sect. 2.1.3 ). (ii) Coding episodes and coding episode types in independent steps offers the possibility of adding inductively new types of episodes.
After parsing a PS process into episodes, we coded the episodes with Schoenfeld’s categories (deductive categories), but also generated new episode types to characterize these episodes (inductive categories). While coding, we observed initial difficulties in coding the deductive episodes reliably; especially differentiating between Analysis and Exploration episode types was difficult (as predicted by Schoenfeld, 1992a , p. 194). We noticed that Schoenfeld’s ( 1985 , Chapter 9) empirical framework referred to his theoretical model of PS processes (ibid., Chapter 4) which was based on Pólya’s ( 1945 ) list of questions and guidelines. Recognizing an analogy between Schoenfeld’s framework and Pólya’s work (see Fig. 1 ), we were able to operationalize their descriptions in a coding manual (see Rott, 2014 ).
When the deductive episode types did not fit our observations, we inductively added a new episode type. This happened three times. Especially in the DGS environment, where students showed behaviour that was not directly related to solving the task, new types of activities occurred. For example, students talked about the software and how to use it. This kind of behaviour was coded by us as Organization . When it took students more than 30 s to write down their findings (without developing any new results or ideas), this episode was coded as Writing . Discussions about things which were not related to mathematics, but for example daily life, were coded as Digression . These codings were used only when activities did not align with numbers 2–6 of Schoenfeld’s list.
This coding of the videotapes was done independently by different research assistants and the first author. We then applied the “percentage of agreement” ( P A ) approach to compute the interrater-agreement as described in the TIMSS 1999 video study (Jacobs et al. 2003 , pp. 99–105), gaining more than P A = 0.7 for parsing PS processes into episodes and more than P A = 0.85 for characterizing the episode types. More importantly, every process was coded by at least two raters. Whenever those codes did not coincide, we attained agreement by recoding together (as in Schoenfeld’s study, 1992a , p. 194).
Product coding : To be able to compare successful and unsuccessful PS processes, students’ products produced in the 45-min sessions were rated. Because the focus was on processes, product rating finally was reduced to a dichotomous right/wrong coding without going into detail regarding students’ argumentations (these will be analysed and the results reported in forthcoming papers). Rating was done independently by a research assistant and the first author with an interrater-agreement of Cohen’s kappa > 0.9. Differing cases were discussed and recoded consensually.
5 Results of our empirical study and implications for our descriptive model
In this section, we briefly illustrate results of our data analyses, which underline the need to go beyond existing models. We summarize key findings of our empirical study and illustrate how these have contributed to the development of our descriptive model of PS processes. After this, we highlight how answering our research questions based on our theoretical and empirical analyses contributes to the development of our descriptive model. Finally, we present and describe our descriptive model.
5.1 Sample problem-solving processes and codings to illustrate the procedure of analysis
To illustrate our analyses and codings of students’ PS processes, we present three sample processes, the first two in detail and the third one only briefly. The first two were paper-and-pencil processes and stem from the same group of students, belonging to parts a) and b) of the ‘Three Beaches’ problem. The third process shows a group of students working on the ‘Shortest Detour’ problem with DGS. Our codings of the different episodes are highlighted in italics .
5.1.1 Group U1-C, Three Beaches (part a))
After reading the Three Beaches problem (00:25–01:30), the three students of group C from tutorial U1 try to understand it. They remember the Airport problem in which they had to find a point with the same distance to all three vertices of a triangle and they try to identify the differences between both problems. The students wonder whether they should again use the perpendicular bisectors of the sides of the triangle or the bisectors of the angles of the triangle ( Analysis , 01:30–05:05). They agree to use the bisectors of the angles and construct their solutions with compasses and ruler. One of the students claims that in the case of an equilateral triangle, perpendicular and angle bisectors would be identical and convinces the others by constructing a triangle and both bisectors with compasses and ruler ( Planning-Implementation , 05:05–06:05). Finally, the students verify their solution by discussing the meaning of the distance from a point to the sides of a triangle, as they initially were not sure how to measure this distance (06:05–07:40). Even though the Analysis episode was quite long (see Fig. 3 ), this part of the task was actually not a problem for the students as they remembered a way to solve it.
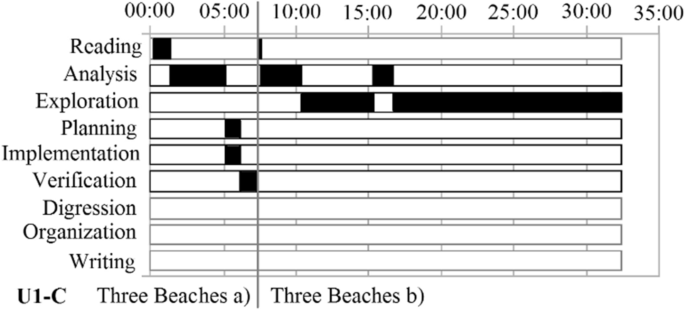
Process codings of the group U1-C, working on the ‘Three Beaches’ problem
5.1.2 Group U1-C, three beaches (part b))
After reading part b) of the problem (07:45–07:55), the students discuss whether the requested point is the same as in a) ( Analysis , 07:55–10:25). They agree to try out and construct a triangle each, place points in it, draw perpendiculars to the sides, and measure the distances. One student asks whether it is allowed to place the point on a vertex and thus have two distances become zero ( Exploration , 10:25–15:30). After this, the students discuss the meaning of distance, particularly the meaning of a distance related to a side of a triangle. They agree that any point on a side, even the vertex, would satisfy the condition of the problem, thus being a suitable site for the ‘house’ ( Analysis , 15:30–16:40). The students wonder why the distance from one vertex to its opposing side (the height of the triangle) is as large as the sum of the distances from the centre of the incircle (from part a)). They remember that the angle bisectors intersect each other in a ratio of 1/3 to 2/3. Thereafter, they continue to place points in their triangles (not on sides) and measure their distances. They finally agree on the [wrong] hypothesis that any point on the angle bisectors is a point with a minimal sum of the distances to the sides; other points in the triangle would have a slightly larger sum [because of inaccuracies in their drawings]. They realize, however, that they cannot give any reasons for their solution ( Exploration , 16:40–32:30). The codings are represented in Fig. 3 (right).
5.1.3 Group Ulap2-TV, shortest detour
Ulap2-TV working on the shortest detour problem (Fig. 4 ) is an example of a process with more transitions. The students solve the first case of the problem ( A and B on different sides of g ) within 5 min ( Planning-Implementation , Verification ) and then explore the second case ( A and B on the same side of g ) for more than 17 min before solving the problem.
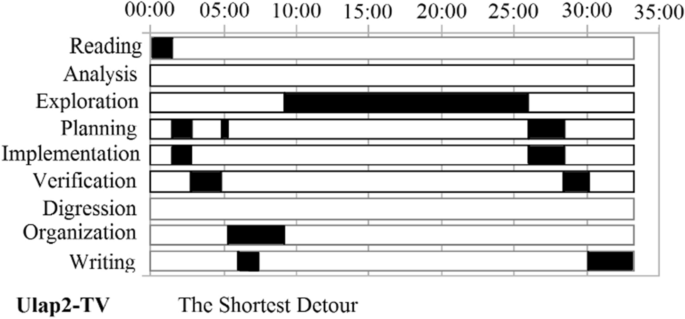
Process coding of the group Ulap2-TV, working on the ‘Shortest Detour’ problem
We selected these three PS processes from our study, as they are examples of our empirical data in several aspects: They illustrate both learning environments (paper-and-pencil and DGS), they incorporate all types of episodes (except for Digression ) and, therefore, all types of phases discussed in the PS research literature, and they include linear and cyclic progressions (see below). The routine process (Three Beaches, part a)) is rather atypical as the students take a lot of time analysing the task, before implementing routine techniques ( Planning-Implementation ). The two PS processes (Three Beaches, part b) and Shortest Detour) are typical for our students, spending a lot of time in Exploration episodes. In the DGS environment, we see that the students take some time to handle the software ( Organization ). Compared to free-hand drawings in paper-and-pencil environments, the students in the DGS environment need to think about constructions ( Planning ) before exploring the situation.
5.2 From theoretical models and empirical results to a descriptive model of problem-solving processes
In the following, the coded episodes from all 33 PS processes of our empirical study are used to answer the first research question. What parts or phases of the established models are suited to describe the analysed processes? Which transitions between phases can be observed? The systematic comparison of PS models from the literature (Sect. 2.1.3 ) is the theoretical underpinning of answering these questions. This process aims at generating a descriptive process model suitable for representing students’ actual PS processes.
5.2.1 Different types of episodes that are suited to describing empirical processes
Within the observed processes, all of Schoenfeld’s episode types could be identified with high interrater agreement. Thus, based on our data, we saw no need to merge phases like Understanding and Planning , even though some models suggest doing so.
More specifically, structured approaches of Planning could be differentiated from unstructured approaches which we call Explorations as suggested by Schoenfeld ( 1985 , Chapters 4 & 9) (in 6 out of 33 non-routine processes, both Exploration and Planning were coded).
Furthermore, in some processes, Planning and Implementation episodes can be differentiated from each other (as suggested by Pólya, 1945 ); there are, however, processes in which those two episode types cannot be distinguished as the problem solvers often do not announce their plans (as predicted by Mason et al. 1982 ). In those PS processes, these two episode types are merged to Planning-Implementation (as done by Schoenfeld as well).
Verification episodes are rare, but can be found in our data. As our students do not show signs of trying to reflect on their use of PS strategies, we decided not to distinguish this episode type into ‘checking’ and ‘reflection’.
Incubation and illumination could not be observed in our sample. This was expected as the students did not have the time to incubate.
Altogether, the following theoretically recorded phases could be identified in our empirical data and are part of our model: understanding (analysis), exploration, planning, implementation (sometimes as planning-implementation), and verification.
5.2.2 Transitions between phases: linearity and non-linearity of the processes
Apart from the phases that occur, the transitions between these phases are of interest. Transitions have been coded between nearly all possible ordered pairs of episode types. If the phases proceed according to Pólya’s or Schoenfeld’s model ( Analysis → Exploration → Planning → Implementation → Verification ), we consider this as a linear process. If phases are omitted within a process but this order is still intact we regard this process still as ‘linear’. In contrast, a process is considered by us as non-linear or cyclic, if this order is violated (e.g., Planning → Exploration ). We also checked whether non-linear processes are cyclic in the sense of Wilson et al. (backward steps are possible after all types of episodes), or whether they are cyclic in the sense of Schoenfeld and Mason et al. (backward steps only before Implementation ).
The first sample process (Three Beaches, part a) illustrates a strictly linear approach as in Pólya’s model, represented in the descending order of the time bars (Fig. 3 , left). The second example (Three Beaches, part b) shows a cyclic process as after the first Exploration , an Analysis was coded (Fig. 3 , right). The third example (Shortest Detour) starts in a linear way; then, after a first Verification , the students go back to Planning-Implementation and Exploration episodes. Thus, overall, their process is cyclic (and not in a way that would fit Schoenfeld’s model as the linear order is broken after a Verification ).
We checked all our process codings for their order of episodes (see Table 1 ). In our sample, a third of the processes are non-linear; thus, a strictly linear model is not suited to describing our students’ PS processes.
5.3 Deriving a model for describing problem-solving processes
Using the results of our empirical study as described in Sects. 5.1 and 5.2 , our findings result in a descriptive model of PS processes. We consider this model as an answer to our first research question. We identified phases from (mostly normative) models in our data, then empirically refined these phases, and took the relevance of their sequencing into account as illustrated in Fig. 5 .
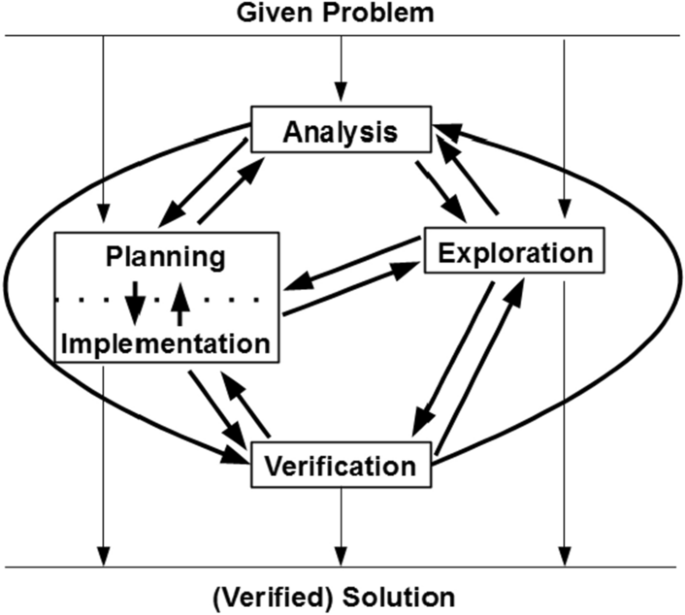
Descriptive model of problem-solving processes
In our descriptive model (see Fig. 5 ), we distinguish between structured ( Planning ) and unstructured ( Exploration ) approaches in accordance with the model of Schoenfeld ( 1985 ). It is also possible to differentiate between explicit planning ( Planning and Implementation coded separately) as well as implicit planning, which means (further) developing a plan while executing it ( Planning and Implementation coded jointly), as suggested by Mason et al. ( 1982 ). Our descriptive model combines ideas from different models in the literature. Furthermore, linear processes can be displayed (using only arrows that point downwards in the direction of the solution) as can non-linear processes (using at least one arrow that points upwards). Therefore, with this model, linear and non-linear PS processes can explicitly be distinguished from each other. Please note that we use ‘(verified) solution’ with a restriction in brackets, as not all processes lead to a verified or even correct solution. Our model is a model of the outer structure as it describes the observable sequence of the different phases.
In the following, we illustrate how far our descriptive model can also respond to our second research question. We use it to describe, as well as to distinguish different types of PS processes.
6 Using our descriptive model to analyse problem-solving processes
Below, we illustrate how our descriptive model (Fig. 5 ) can be used to analyse and compare students’ PS processes. We first reconstruct different processes of student groups and then propose a new way to represent typical transitions in students’ PS processes.
6.1 Representing students’ problem-solving processes
In contrast to the process coding by Schoenfeld, which contains specific information about the duration of episodes, our analyses are more abstract. We focus on the empirically found types of episodes and transitions between these episodes. This is done following Schoenfeld ( 1985 ), who emphasised: “The juncture between episodes is, in most cases, where managerial decisions (or their absence) will make or break a solution” (p. 300). Focusing on the transitions between episodes is one important characteristic that distinguishes different types of PS processes. Using our descriptive model allows one to do this.
For each process, the transitions between episodes can be displayed with our model (Fig. 5 ). In the following, we consider only the five content-related episode types, but not Reading , Organization , Writing , and Digression, as activities of the latter types of episodes do not contribute to the solution and they are not ordered as in Pólya’s or Schoenfeld’s phases.
For example, the routine process of group U1-C (Three Beaches, part a), see Sect. 5.1 ), starts with an Analysis , followed by a merged Planning-Implementation and a Verification or, in short: [A,P-I,V]; thereafter, this process ends. This means, there are four different transitions in this process indicated by arrows: Start → A, A → P-I, P-I → V, and V → End. Thus, in Fig. 6 (left), these transitions are illustrated with arrows. In this case, these transitions each occur only once, which is indicated by a circled number 1.
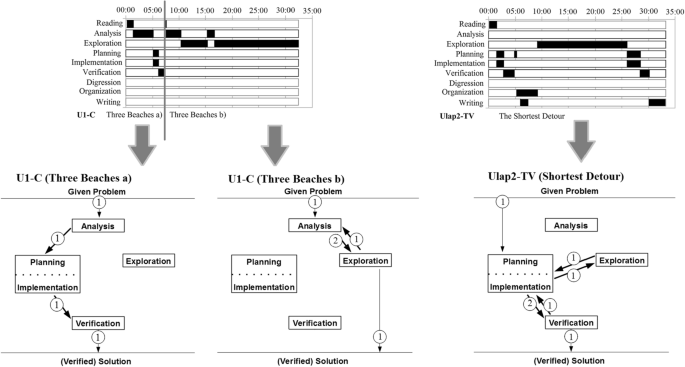
Translation from Schoenfeld codings to a representation using the descriptive model; the circled numbers indicate the number of times a transition occurs
The second example (U1-C, Three Beaches, part b)) consists of the following episodes: Analysis–Exploration–Analysis–Exploration [A,E,A,E]. This means that there are five transitions in this process: Start → A, A → E, E → A, A → E, and E → End (see Fig. 6 , middle). Please notice that the transition A → E is observed twice.
The final example shows group Ulap2-TV (Shortest Detour), which starts with a Planning-Implementation and proceeds through [P-I,V,P,E,P-I,V] with a total of seven transitions, two of which are P-I → V (ignoring Organization and Writing , Fig. 6 , right).
This reduction to transitions, neglecting the exact order and the duration of episodes, enables one to do a specific comparison of processes and an accumulation of several PS processes (e.g., from all DGS processes, see Sect. 6.2 ). The focus is now on transitions and how often they happen, which indicates different types of PS processes as shown below. This ‘translation’ from the Schoenfeld coding to the representation in our descriptive model has been done for all 33 processes. The directions of the arrows indicate from which phase to which the transitions are occurring, e.g., from analysis to planning; the numbers on the arrows show how often these transitions were coded (they do not indicate an order).
The three selected processes already show clearly different paths, for example, linear vs. cyclic (see Sect. 2.2.4).
6.2 Characterizing types of problem-solving processes by accumulation
Students’ PS processes can be successful or non-successful or conducted in paper-and-pencil or DGS contexts. Looking at different groups of students simultaneously can be fruitful, as such accumulations allow one to look at patterns in existing transitions. Our descriptive model allows one to consider several processes at once, via accumulation.
Representations of single processes, as presented in Fig. 6 and in the boxes in Fig. 7 , can be combined by adding up all coded transitions (which would be impossible with time bars used by Schoenfeld). For such an accumulation, we count all transitions between types of episodes and display them in numbers next to the arrows representing the number of those transitions. For example, six of the processes in the outer boxes start with a transition from the given problem to Planning , while one process begins with an Analysis . This is shown in the centre box by the numbers 6 and 1 in the arrows from the given problem to Planning and Analysis , respectively (see Fig. 7 for the combination of all processes regarding task 3a). Arrows were drawn only where transitions actually occurred in this task. Looking at the arrows that start at the ‘given problem’ or that lead to the ‘(verified) solution’, one can see how many processes were accumulated. All episode types (small boxes) must have the same number of transitions towards as well as from this episode type.
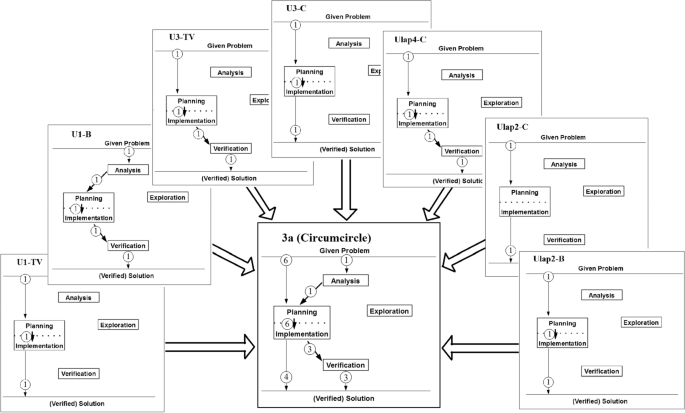
Centre rectangle: Accumulation of seven different group processes regarding task 3a)
To show the usability of our model, we distinguish between working on routine tasks and on problems in Sect. 6.2.1 ; thereafter, the routine processes are not further considered.
6.2.1 Routine vs. non-routine processes
In our study, two sub-tasks (3a) and 4a)) were routine tasks in which the students were asked to find special points in triangles. If we look at the accumulations of those processes in our model, clear patterns emerge: There are no Exploration episodes at all, either in the seven processes of task 3a) (Fig. 8 , left) nor in the eight processes of tasks 4a) (Fig. 8 , middle). Instead, there are Planning and/or Implementation episodes in all 15 processes. In some of those processes, Planning and Implementation can clearly be coded as two separate episodes. In other processes, it is not possible to discriminate between these episode types as two distinct episodes in the empirical data (see Fig. 8 ).
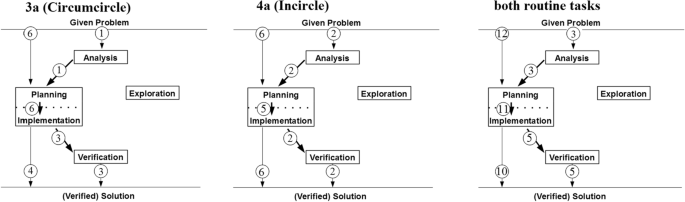
Accumulation of seven processes for the routine task 3a) (left) and eight processes for task 4a) (middle), 15 processes in total (right)
Most processes (12 out of 15) show no need for analysing the task but start directly with Planning and/or Implementation . Even though there are five Verification episodes, these verifications are often only short checking activities with no reflection in the sense of Pólya; however, the length and quality of an episode cannot be seen in the model. Additionally, all of these 15 processes are linear (as can be seen by the arrows, which point only downwards).
In contrast to these routine tasks, non-routine processes are often non-linear and contain at least one Exploration episode. In Fig. 9 , in direct comparison to Fig. 8 , the seven PS processes of problem 3a) (left), the eight PS processes of problem 3b) (middle), and an accumulation of the 15 PS processes (right) are shown. Overall, in these 15 processes, 17 Exploration episodes were coded, which can be seen in Fig. 9 (right): 4 processes start with an Exploration ; 12 times there is an Exploration after an Analysis episode; and once after Planning-Implementation .
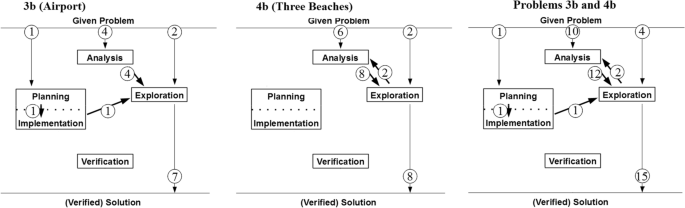
Accumulation of seven processes for problem 3b) (left) and eight processes for problem 4b) (middle), 15 problem-solving processes in total (right)
In Fig. 10 (right), an accumulation is given of all 33 PS processes of all five problems. The differences of the routine and the PS processes (e.g., the latter containing Exploration episodes and being cyclic) can be seen by comparing Figs. 8 and 9 .
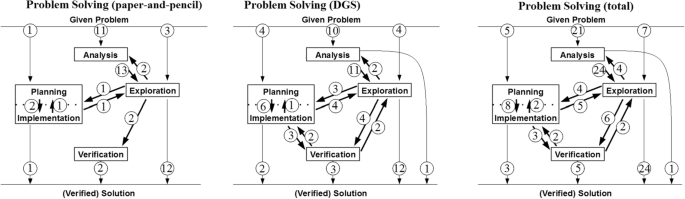
Accumulation of transitions in problem-solving processes, paper-and-pencil (left) vs. DGS (middle); all problem-solving processes (right)
6.2.2 Successful and unsuccessful problem-solving processes
One of Schoenfeld's ( 1985 ) major results was the importance of self-regulatory activities in PS processes. Schoenfeld was not able to characterize successful PS processes; however, he identified characteristics of processes that did not end in a verified solution. The unsuccessful problem solvers were most often those who missed out on self-regulatory activities (i.e., controlling interim results or planning next steps); they engaged in a behaviour that Schoenfeld called “wild goose chase” and that he described this way:
Approximately 60% of the protocols were of the type [...], where the students read the problem, picked a solution direction (often with little analysis or rationalization), and then pursued that approach until they ran out of time. In contrast, successful solution attempts came in a variety of shapes and sizes—but they consistently contained a significant amount of self-regulatory activity, which could clearly be seen as contributing to the problem solvers’ success. (Schoenfeld, 1992a , p. 195)
We made similar observations looking at the processes of our students; several of them, who did not show any signs of structured actions or process evaluations, were not able to solve the tasks. Thus, to test if this observation was statistically significant, we had to operationalize the PS type “wild goose chase”, as Schoenfeld had provided no operational definition for this phenomenon. A process is considered by us to be a “wild goose chase”, if it consists of only Exploration or Analysis & Exploration episodes, whereas processes that are not of this type contain planning and/or verifying activities (only considering content-related episode types). In our descriptive model, by definition, wild goose chase processes look like the process manifested by U1-C (Three Beaches, part b) (Fig. 6 , middle).
To check if the kind of behaviour in these processes is interrelated with success or failure of the related products (see Sect. 4.2 ), a chi-square test was used (because of the nominal character of the process categories, no Pearson or Spearman correlation could be calculated). The null hypothesis was ‘there is no correlation between the PS type wild goose chase and (no) success in the product’.
The entries in Table 2 consist of the observed numbers of process–product combinations; the expected numbers assuming statistical independence (calculated by the marginal totals) are added in parentheses. The entries in the main diagonal are apparently higher than the expected values. The test shows a significant correlation ( p < 0.01) between the problem solvers’ behaviour and their success. Therefore, the null hypothesis can be rejected, there is a correlation between showing wild goose chase-behaviour in PS processes and not being successful in solving the problem.
6.2.3 Paper-and-pencil vs. DGS environment processes
Looking at the processes of the non-routine tasks indicates that the tasks were ‘problems’ for the students, as these processes showed no signs of routine behaviour (see Sect. 6.2.1 ). Instead, we see many transitions between different episodes and the typical cyclic structure of PS processes. Comparing accumulations of all 15 paper-and-pencil with all 18 DGS PS processes, we see some interesting differences, which our model helps to reveal (see Fig. 10 ). The time the students worked on the problem was set in the tutorials and, therefore, identical in both environments and in all processes. At the end of this paper, we discuss three aspects that our comparisons revealed; more detailed analyses are planned for forthcoming papers.
We coded more transitions in DGS than in paper-and-pencil processes (73 transitions in 18 DGS processes, in short: 73/18 or on average 4 transitions per DGS process compared to 52/15 or 3.5 transitions per paper-and-pencil process). If transitions are a sign of self-regulation (Schoenfeld, 1985 ; Wilson et al. 1993 ), our students in the DGS environment seem to better regulate their processes (please note that Organization episodes are not counted here; including them would further add transitions to DGS processes). However, there might be more transitions (and thus episodes) in DGS processes because of having more time for exploring situations and generating examples, which does not take as much time as in paper-and-pencil processes.
We see more Planning (and Implementation ) episodes in DGS than in paper-and-pencil processes (9/18 or Planning in 50% of the DGS processes compared to 2/15 or 13% in paper-and-pencil processes). Using Schoenfeld’s conceptualization of Planning and Exploration episodes, the DGS processes seem to be more structured—especially since there are less Exploration episodes in DGS than in paper-and-pencil processes (17/18 compared to 21/15), even though there are more episodes in the DGS environment (see above). There seems to be a need for students in the DGS environment to plan their actions, especially when it comes to complex constructions that cannot be sketched freehandedly as in the paper-and-pencil environment. Considering the success of the students (6 solutions in the DGS environment compared to 3 in the paper-and-pencil environment), this hypothesis is supported. As already existing research indicates, better regulated PS processes should be more successful. Please note that successful solutions cannot be obtained by stating only correct hypotheses, which would favour the DGS environment; solutions coded as ‘correct’ had to be argued for.
We double checked our codings to make sure that this result was not an artefact of the coding, that the students actually planned their actions, not only using the DGS (which was coded in Organization episodes). This result could be due to our setting, as our student peer groups had only one computer and thus needed to talk about their actions. In future studies, it should be investigated if this phenomenon can be replicated in environments in which each student has his or her own computer.
We also observed more Verification episodes in DGS compared to paper-and-pencil processes (7/18 or 39% compared to 2/15 or 13%). There could be different reasons for this observation, e.g., students not trusting the technology, or just the simplicity of using the dragmode to check results compared to making drawings in the paper-and-pencil environment.
The results of using our descriptive model for comparisons of PS processes appear to be insightful. The purpose of this section was to illustrate these insights and the use of our empirical model of PS processes. Accumulating PS processes of several groups is a key to enabling comparisons such as the ones presented.
7 Discussion
The goal of this paper was to present a descriptive model of PS processes, that is, a model suited to the description and analyses of empirically observed PS processes. So far, existing research has mainly discussed and applied normative models for PS processes, which are generally used to instruct people, particularly students, about ideal ways of approaching problems. There exist a few, well accepted, models of PS processes in mathematics education (Fig. 1 ); however, these models only partly allow represention of and emphasis on the non-linearity of real and empirical PS processes, and they do not have the potential to compare processes across groups of students. For the generation of our descriptive model of PS processes, following our first research question, (1) the existing models were compared. It turned out that similarities and fine differences exist between the current normative models, especially regarding the phases of PS processes and their sequencing. We identified which elements of the existing models could be useful for the generation of a descriptive model, linking theoretical considerations from research literature with regard to our empirical data. Analysing PS processes of students working on geometric problems, we observed that distinctive episodes (esp. the distinction between Planning and Exploration ) and transitions between episodes, were essential. Classifying the episodes was mostly possible with the existing models, but characterising their transitions and sequencing required extension of the existing models, which resulted in a juxtaposition of components for our descriptive phase model (e.g., allowing us to code, separately or in combination, Planning-Implementation or to regard the (non-)linearity of processes).
Our generated descriptive model turned out not only to provide valuable insights into problems solving processes of students, but also with respect to our second research question, (2), to compare, contrast, and characterise the idiosyncratic characteristics of students’ PS processes (using Explorations or not, linear or cyclic processes, including Verification and Planning or not). Our developed descriptive model can be used to analyse processes of students ‘at once’, in accumulation, which allowed us to group and characterise comparisons of students’ processes, which was not possible with the existing models. As demonstrated in Sect. 6.2 , our model further allows one to distinguish students’ PS processes while working on routine versus problem tasks. Applying our descriptive model to routine tasks, we detected linear processes, whereas in problem tasks cyclic processes were characteristic. Furthermore, in routine tasks, no Exploration episodes could be coded. Most of the students expressed no need for analysing the task but started directly with Planning and/or Implementation.
Our descriptive model also allows one to recognize a type of PS behaviour already described by Schoenfeld ( 1992a ) as “wild goose chases”. Our data illustrated that wild goose chase processes are statistically correlated with unsuccessful attempts at solving the given problems.
In addition, our descriptive model indicated differences between paper and pencil and DGS processes. In the latter context, students showed more transitions, more Planning (and Implementation ), and more Verification episodes. This result revealed significantly different approaches that students embarked on when working on problems in paper and pencil or DGS environments. These findings might indicate that in the DGS environment in our study, students better regulated their processes (cf. Schoenfeld, 1985 , 1992b ; Wilson et al. 1993 )—a hypothesis yet to be confirmed.
A limitation of our study might be the difficulty of the problems given to our students; only 9 of 33 processes ended with a correct solution. In future studies, problems should be used that better differentiate between successful and unsuccessful problem solvers. Also, our descriptive model has so far been grounded only in university students’ geometric PS processes. Even though geometry is particularly suited for learning mathematical PS in general and heuristics in specific (see Schoenfeld, 1985 ), other contexts and fields of mathematics might highlight other challenges students face. Further empirical evidence is needed to see how far our model is also useful and suitable to describe other contexts with respect to specifics of their mathematical fields. Following some of our ideas and insights, Rott ( 2014 ) has already conducted such a study: fifth graders working on problems from geometry, number theory, combinatorics, and arithmetic. Similar results as in the study presented here, were seen and indicate the value of our descriptive model. More research in this regard is a desideratum.
Regarding teaching, using our model can be helpful to discuss with students on a meta-level these documented distinct phases of PS processes, transitions between them, and the possibility of going back to each phase during a PS process. This might help students to be aware of their processes, of different ways to gain a solution and justification, and to be more flexible during PS processes. More reflection on this aspect is also a desideratum for future research.
Change history
12 march 2021.
ESM was added
Artzt, A., & Armour-Thomas, E. (1992). Development of a cognitive-metacognitive framework for protocol analysis of mathematical problem solving in small groups. Cognition and Instruction, 9 (2), 137–175.
Article Google Scholar
Dewey, J. (1910). How we think . D C Heath.
Book Google Scholar
Fernandez, M. L., Hadaway, N., & Wilson, J. W. (1994). Problem solving: Managing it all. The Mathematics Teacher, 87 (3), 195–199.
Gawlick, T. (2002). On Dynamic Geometry Software in the regular classroom. ZDM, 34 (3), 85–92.
Google Scholar
Hadamard, J. (1945). The psychology of invention in the mathematical field . Princeton University Press.
Halmos, P. R. (1980). The heart of mathematics. The American Mathematical Monthly, 87 (7), 519–524.
Jacinto, H., & Carreira, S. (2017). Mathematical problem solving with technology: The techno-mathematical fluency of a student-with-GeoGebra. International Journal of Science and Mathematics Education, 15, 1115–1136.
Jacobs, J., Garnier, H., Gallimore, R., Hollingsworth, H., Givvin, K. B., Rust, K. et al. (2003). Third International Mathematics and Science Study 1999 Video Study Technical Report. Volume 1: Mathematics . National Center for Education Statistics. Institute of Education Statistics, U. S. Department of Education.
KMK. (2004). Beschlüsse der Kultusministerkonferenz. Bildungsstandards im Fach Mathematik für den Mittleren Schulabschluss [Educational standards in mathematics for secondary school leaving certificates] . Wolters Kluwer.
Koyuncu, I., Akyuz, D., & Cakiroglu, E. (2015). Investigation plane geometry problem-solving strategies of prospective mathematics teachers in technology and paper-and-pencil environments. International Journal of Mathematics and Science Education, 13, 837–862.
Liljedahl, P., Santos-Trigo, M., Malaspina, U., & Bruder, R. (2016). Problem solving in mathematics education . ICME Topical Surveys.
Mason, J., Burton, L., & Stacey, K. (1982). Thinking mathematically . Pearson.
NCTM. (2000). National Council of Teachers of Mathematics. Principles and standards of school mathematics . NCTM.
NCTM. (2014). Principles to actions. Ensuring mathematical success for all . NCTM.
Neuhaus, K. (2002). Die Rolle des Kreativitätsproblems in der Mathematikdidaktik [The role of the creativity problem in mathematics education] . Dr. Köster.
Philipp, K. (2013). Experimentelles Denken. Theoretische und empirische Konkretisierung einer mathematischen Kompetenz [Experimental thinking] . Springer.
Poincaré, H. (1908). Science et méthode [Science and method] . Flammarion.
Pólya, G. (1945). How to solve it . Princeton University Press.
Rott, B. (2014). Mathematische Problembearbeitungsprozesse von Fünftklässlern—Entwicklung eines deskriptiven Phasenmodells [Problem-solving processes of fifth graders: Developing a descriptive phase model]. Journal für Mathematik-Didaktik, 35, 251–282.
Schoenfeld, A. H. (1985). Mathematical problem solving . Academic Press.
Schoenfeld, A. H. (1992a). On paradigms and methods: What do you do when the ones you know don’t do what you want them to? Issues in the analysis of data in the form of videotapes. The Journal of the Learning Sciences, 2 (2), 179–214.
Schoenfeld, A. H. (1992b). Learning to think mathematically: Problem solving, metacognition, and sensemaking in mathematics. In D. A. Grouws (Ed.), Handbook for research on mathematics teaching and learning (pp. 334–370). MacMillan.
Sträßer, R. (2002). Research on dynamic geometry software (DGS)—An introduction. ZDM, 34 (3), 65.
Wallas, G. (1926). The art of thought . C.A. Watts & Co.
Wilson, J. W., Fernandez, M. L., & Hadaway, N. (1993). Mathematical problem solving. In P. S. Wilson (Ed.), Research ideas for the classroom: High school mathematics (pp. 57–77). MacMillan.
Yimer, A., & Ellerton, N. F. (2010). A five-phase model for mathematical problem solving: Identifying synergies in pre-service-teachers’ metacognitive and cognitive actions. ZDM - The International Journal on Mathematics Education, 42, 245–261.
Download references
Open Access funding enabled and organized by Projekt DEAL.. Open Access funding enabled and organized by Projekt DEAL.
Author information
Authors and affiliations.
Universität zu Köln, Köln, Germany
Benjamin Rott
Universität Oldenburg, Oldenburg, Germany
Birte Specht
Universität Bremen, Bremen, Germany
Christine Knipping
You can also search for this author in PubMed Google Scholar
Corresponding author
Correspondence to Benjamin Rott .
Additional information
Publisher's note.
Springer Nature remains neutral with regard to jurisdictional claims in published maps and institutional affiliations.
Supplementary Information
Below is the link to the electronic supplementary material.
Supplementary file 1 (PDF 172 KB)
Rights and permissions.
Open Access This article is licensed under a Creative Commons Attribution 4.0 International License, which permits use, sharing, adaptation, distribution and reproduction in any medium or format, as long as you give appropriate credit to the original author(s) and the source, provide a link to the Creative Commons licence, and indicate if changes were made. The images or other third party material in this article are included in the article's Creative Commons licence, unless indicated otherwise in a credit line to the material. If material is not included in the article's Creative Commons licence and your intended use is not permitted by statutory regulation or exceeds the permitted use, you will need to obtain permission directly from the copyright holder. To view a copy of this licence, visit http://creativecommons.org/licenses/by/4.0/ .
Reprints and permissions
About this article
Rott, B., Specht, B. & Knipping, C. A descriptive phase model of problem-solving processes. ZDM Mathematics Education 53 , 737–752 (2021). https://doi.org/10.1007/s11858-021-01244-3
Download citation
Accepted : 18 February 2021
Published : 09 March 2021
Issue Date : August 2021
DOI : https://doi.org/10.1007/s11858-021-01244-3
Share this article
Anyone you share the following link with will be able to read this content:
Sorry, a shareable link is not currently available for this article.
Provided by the Springer Nature SharedIt content-sharing initiative
- Mathematical problem solving
- Descriptive process model
- Find a journal
- Publish with us
- Track your research

IMAGES
VIDEO
COMMENTS
List the three phases of the computer problem-solving model. Algorithm-Development Phase Implementation Phase Maintenance Phase. How does the computer problem-solving model differ from Polya's. In Polya's list, the human executes the plan and evaluates the results. In a computer solution, a program is written that expresses the plan in a ...
Object-oriented design: A problem-solving methodology that produces a solution to a problem in terms of self-contained entities called objects. Describe the steps in the implementation phase. The implementation phase includes coding (translating the algorithm into a computer language) and testing (compiling and running the program).
Identify the people, information (data), and things needed to resolve the problem. Step. Description. Step 3: Select an Alternative. After you have evaluated each alternative, select the alternative that comes closest to solving the problem with the most advantages and fewest disadvantages.
The development of an algorithm (a plan) is a key step in solving a problem. Once we have an algorithm, we can translate it into a computer program in some programming language. Our algorithm development process consists of five major steps. Step 1: Obtain a description of the problem. Step 2: Analyze the problem.
Study with Quizlet and memorize flashcards containing terms like The first strategy to use when given a problem, Don't reinvent the wheel, three phases of the computer problem-solving model and more.
The three phases of the process are formulation, analysis, and interpretation. During the formulation phase of the process, the analyst defines the problem, determines assessment criteria, and develops alternatives. These elements are followed by an analysis phase using modeling and optimization. The last phase, interpretation, encompasses ...
Solving a complex computational problem is an adaptive process that follows iterative cycles of ideation, testing, debugging, and further development. Computational problem solving involves systematically evaluating the state of one's own work, identifying when and how a given operation requires fixing, and implementing the needed corrections.
The Six Step Problem Solving Model provides individuals and teams with an effective framework for approaching and resolving problems. By defining the problem, analyzing it thoroughly, generating potential solutions, choosing the most suitable option, implementing it effectively, and continuously evaluating and adjusting strategies, you can ...
Here is a six-step process to follow when using a problem-solving model: 1. Define the problem. First, determine the problem that your team needs to solve. During this step, teams may encourage open and honest communication so everyone feels comfortable sharing their thoughts and concerns.
Computer Science Illuminated (7th Edition) Edit edition Solutions for Chapter 7 Problem 44E: List the phases of the computer problem-solving model. … Solutions for problems in chapter 7 1E
The Problem-Solving Process. Problem-solving is an important part of planning and decision-making. The process has much in common with the decision-making process, and in the case of complex decisions, can form part of the process itself. We face and solve problems every day, in a variety of guises and of differing complexity.
Finding a suitable solution for issues can be accomplished by following the basic four-step problem-solving process and methodology outlined below. Step. Characteristics. 1. Define the problem. Differentiate fact from opinion. Specify underlying causes. Consult each faction involved for information. State the problem specifically.
Essentially every problem-solving heuristic in mathematics goes back to George Polya's How to Solve It; my approach is no exception. However, this cyclic description might help to keep the process cognitively present. A few months ago, I produced a video describing this the three stages of the problem-solving cycle: Understand, Strategize, and Implement.
The first two steps are for defining and measuring the problem. The third step is the analysis. And the fourth and fifth steps are improve and control, and address solutions. 3 Basic Steps of Problem Solving. As the name suggests, problem solving starts with a problem and ends with solutions. The step in the middle is the analysis.
1).List the four steps in Polya's How-To-Solve-It list. 2).Describe the four steps listed in Exercise 1 in your own words. 3).List the problem-solving strategies discussed in this chapter. 4).Apply the problem-solving strategies to the following situations. a. Buying a toy for your four-year-old cousin.
VIDEO ANSWER: List the three phases of the computer problem-solving model. Get 5 free video unlocks on our app with code GOMOBILE Invite sent!
The Six Step Problem Solving Model Problem solving models are used to address the many challenges that arise in the workplace. While many people regularly solve problems, there are a range of different approaches that can be used to find a solution. Complex challenges for teams, working groups and boards etc., are usually solved more quickly by ...
Using a problem solving model enables a group to consider all possible causes of a problem and all possible solutions. A problem solving model uses a series of logical steps to help a group identify the most important causes and the best solution. Following the model not only helps the group arrive at a solution, it helps the group arrive at a
Complementary to existing normative models, in this paper we suggest a descriptive phase model of problem solving. Real, not ideal, problem-solving processes contain errors, detours, and cycles, and they do not follow a predetermined sequence, as is presumed in normative models. To represent and emphasize the non-linearity of empirical processes, a descriptive model seemed essential. The ...
Iterative algorithms use WHILE statements. List the four steps in Polya's How To Solve It List. Describe the four steps listed in Exercise 37 in your own words. List the problem-solving strategies discussed in this chapter. Apply the problem-solving strategies to the following situations.
Click here 👆 to get an answer to your question ️ List the three phases of the computer problem-solving model. List the three phases of the computer problem-solving model. - brainly.com See what teachers have to say about Brainly's new learning tools!
Computer Science Illuminated | 6th Edition. ISBN-13: 9781284055917 ISBN: 1284055914 Authors: Agnes G Loeffler, Nell Dale, John Lewis, Michael N Hart, John Lewis Rent | Buy. This is an alternate ISBN. View the primary ISBN for: Computer Science Illuminated 6th Edition Textbook Solutions.
Computer Science Illuminated (7th Edition) Edit edition This problem has been solved: Solutions for Chapter 7 Problem 44E: List the phases of the computer problem-solving model. …