Home » JavaScript Tutorial » JavaScript Assignment Operators

JavaScript Assignment Operators
Summary : in this tutorial, you will learn how to use JavaScript assignment operators to assign a value to a variable.
Introduction to JavaScript assignment operators
An assignment operator ( = ) assigns a value to a variable. The syntax of the assignment operator is as follows:
In this syntax, JavaScript evaluates the expression b first and assigns the result to the variable a .
The following example declares the counter variable and initializes its value to zero:
The following example increases the counter variable by one and assigns the result to the counter variable:
When evaluating the second statement, JavaScript evaluates the expression on the right-hand first ( counter + 1 ) and assigns the result to the counter variable. After the second assignment, the counter variable is 1 .
To make the code more concise, you can use the += operator like this:
In this syntax, you don’t have to repeat the counter variable twice in the assignment.
The following table illustrates assignment operators that are shorthand for another operator and the assignment:
Operator | Meaning | Description |
---|---|---|
Assigns the value of to . | ||
Assigns the result of plus to . | ||
Assigns the result of minus to . | ||
Assigns the result of times to . | ||
Assigns the result of divided by to . | ||
Assigns the result of modulo to . | ||
Assigns the result of AND to . | ||
Assigns the result of OR to . | ||
Assigns the result of XOR to . | ||
Assigns the result of shifted left by to . | ||
Assigns the result of shifted right (sign preserved) by to . | ||
Assigns the result of shifted right by to . |
Chaining JavaScript assignment operators
If you want to assign a single value to multiple variables, you can chain the assignment operators. For example:
In this example, JavaScript evaluates from right to left. Therefore, it does the following:
- Use the assignment operator ( = ) to assign a value to a variable.
- Chain the assignment operators if you want to assign a single value to multiple variables.

JAVASCRIPT ASSIGNMENT OPERATORS
In this tutorial, you will learn about all the different assignment operators in javascript and how to use them in javascript.
Assignment Operators
In javascript, there are 16 different assignment operators that are used to assign value to the variable. It is shorthand of other operators which is recommended to use.
The assignment operators are used to assign value based on the right operand to its left operand.
The left operand must be a variable while the right operand may be a variable, number, boolean, string, expression, object, or combination of any other.
One of the most basic assignment operators is equal = , which is used to directly assign a value.
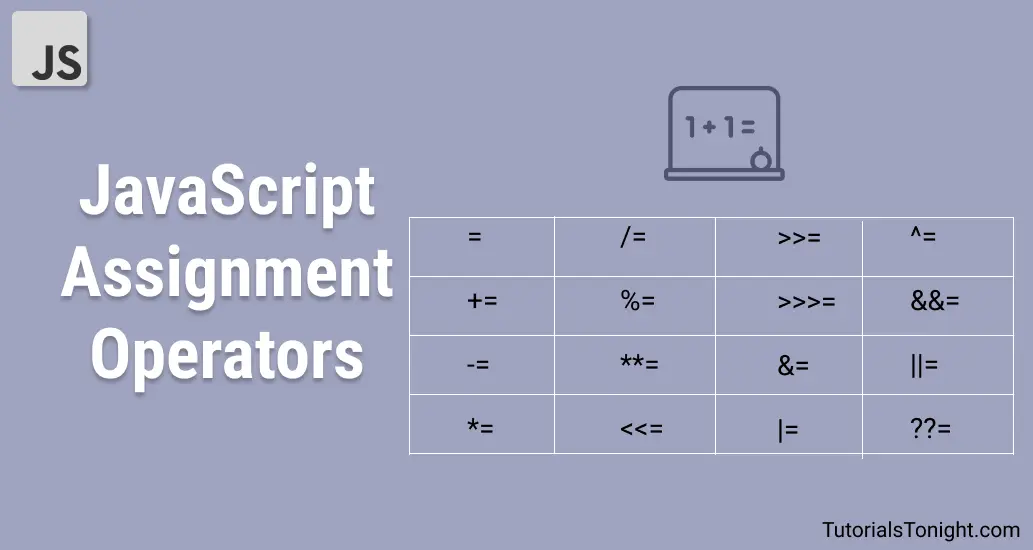
Assignment Operators List
Here is the list of all assignment operators in JavaScript:
In the following table if variable a is not defined then assume it to be 10.
Operator | Description | Example | Equivalent to |
---|---|---|---|
= | a = 10 | a = 10 | |
+= | a += 10 | a = a + 10 | |
-= | a -= 10 | a = a - 10 | |
*= | a *= 10 | a = a * 10 | |
/= | a /= 10 | a = a / 10 | |
%= | a %= 10 | a = a % 10 | |
**= | a **= 2 | a = a ** 2 | |
<<= | a <<= 1 | a = a << 1 | |
>>= | a >>= 2 | a = a >> 2 | |
>>>= | a >>>= 1 | a = a >>> 1 | |
&= | a &= 4 | a = a & 4 | |
|= | a |= 2 | a = a | 2 | |
^= | a ^= 5 | a = a ^ 5 | |
&&= | a &&= 3 | a = a && 3 | |
||= | a ||= 4 | a = a || 4 | |
??= | a ??= 2 | a = a ?? 2 |
Assignment operator
The assignment operator = is the simplest value assigning operator which assigns a given value to a variable.
The assignment operators support chaining, which means you can assign a single value in multiple variables in a single line.
Addition assignment operator
The addition assignment operator += is used to add the value of the right operand to the value of the left operand and assigns the result to the left operand.
On the basis of the data type of variable, the addition assignment operator may add or concatenate the variables.
Subtraction assignment operator
The subtraction assignment operator -= subtracts the value of the right operand from the value of the left operand and assigns the result to the left operand.
If the value can not be subtracted then it results in a NaN .
Multiplication assignment operator
The multiplication assignment operator *= assigns the result to the left operand after multiplying values of the left and right operand.
Division assignment operator
The division assignment operator /= divides the value of the left operand by the value of the right operand and assigns the result to the left operand.
Remainder assignment operator
The remainder assignment operator %= assigns the remainder to the left operand after dividing the value of the left operand by the value of the right operand.
Exponentiation assignment operator
The exponential assignment operator **= assigns the result of exponentiation to the left operand after exponentiating the value of the left operand by the value of the right operand.
Left shift assignment
The left shift assignment operator <<= assigns the result of the left shift to the left operand after shifting the value of the left operand by the value of the right operand.
Right shift assignment
The right shift assignment operator >>= assigns the result of the right shift to the left operand after shifting the value of the left operand by the value of the right operand.
Unsigned right shift assignment
The unsigned right shift assignment operator >>>= assigns the result of the unsigned right shift to the left operand after shifting the value of the left operand by the value of the right operand.
Bitwise AND assignment
The bitwise AND assignment operator &= assigns the result of bitwise AND to the left operand after ANDing the value of the left operand by the value of the right operand.
Bitwise OR assignment
The bitwise OR assignment operator |= assigns the result of bitwise OR to the left operand after ORing the value of left operand by the value of the right operand.
Bitwise XOR assignment
The bitwise XOR assignment operator ^= assigns the result of bitwise XOR to the left operand after XORing the value of the left operand by the value of the right operand.
Logical AND assignment
The logical AND assignment operator &&= assigns value to left operand only when it is truthy .
Note : A truthy value is a value that is considered true when encountered in a boolean context.
Logical OR assignment
The logical OR assignment operator ||= assigns value to left operand only when it is falsy .
Note : A falsy value is a value that is considered false when encountered in a boolean context.
Destructuring assignment
The two most used data structures in JavaScript are Object and Array .
- Objects allow us to create a single entity that stores data items by key.
- Arrays allow us to gather data items into an ordered list.
However, when we pass these to a function, we may not need all of it. The function might only require certain elements or properties.
Destructuring assignment is a special syntax that allows us to “unpack” arrays or objects into a bunch of variables, as sometimes that’s more convenient.
Destructuring also works well with complex functions that have a lot of parameters, default values, and so on. Soon we’ll see that.
Array destructuring
Here’s an example of how an array is destructured into variables:
Now we can work with variables instead of array members.
It looks great when combined with split or other array-returning methods:
As you can see, the syntax is simple. There are several peculiar details though. Let’s see more examples to understand it better.
It’s called “destructuring assignment,” because it “destructurizes” by copying items into variables. However, the array itself is not modified.
It’s just a shorter way to write:
Unwanted elements of the array can also be thrown away via an extra comma:
In the code above, the second element of the array is skipped, the third one is assigned to title , and the rest of the array items are also skipped (as there are no variables for them).
…Actually, we can use it with any iterable, not only arrays:
That works, because internally a destructuring assignment works by iterating over the right value. It’s a kind of syntax sugar for calling for..of over the value to the right of = and assigning the values.
We can use any “assignables” on the left side.
For instance, an object property:
In the previous chapter, we saw the Object.entries(obj) method.
We can use it with destructuring to loop over the keys-and-values of an object:
The similar code for a Map is simpler, as it’s iterable:
There’s a well-known trick for swapping values of two variables using a destructuring assignment:
Here we create a temporary array of two variables and immediately destructure it in swapped order.
We can swap more than two variables this way.
The rest ‘…’
Usually, if the array is longer than the list at the left, the “extra” items are omitted.
For example, here only two items are taken, and the rest is just ignored:
If we’d like also to gather all that follows – we can add one more parameter that gets “the rest” using three dots "..." :
The value of rest is the array of the remaining array elements.
We can use any other variable name in place of rest , just make sure it has three dots before it and goes last in the destructuring assignment.
Default values
If the array is shorter than the list of variables on the left, there will be no errors. Absent values are considered undefined:
If we want a “default” value to replace the missing one, we can provide it using = :
Default values can be more complex expressions or even function calls. They are evaluated only if the value is not provided.
For instance, here we use the prompt function for two defaults:
Please note: the prompt will run only for the missing value ( surname ).
Object destructuring
The destructuring assignment also works with objects.
The basic syntax is:
We should have an existing object on the right side, that we want to split into variables. The left side contains an object-like “pattern” for corresponding properties. In the simplest case, that’s a list of variable names in {...} .
For instance:
Properties options.title , options.width and options.height are assigned to the corresponding variables.
The order does not matter. This works too:
The pattern on the left side may be more complex and specify the mapping between properties and variables.
If we want to assign a property to a variable with another name, for instance, make options.width go into the variable named w , then we can set the variable name using a colon:
The colon shows “what : goes where”. In the example above the property width goes to w , property height goes to h , and title is assigned to the same name.
For potentially missing properties we can set default values using "=" , like this:
Just like with arrays or function parameters, default values can be any expressions or even function calls. They will be evaluated if the value is not provided.
In the code below prompt asks for width , but not for title :
We also can combine both the colon and equality:
If we have a complex object with many properties, we can extract only what we need:
The rest pattern “…”
What if the object has more properties than we have variables? Can we take some and then assign the “rest” somewhere?
We can use the rest pattern, just like we did with arrays. It’s not supported by some older browsers (IE, use Babel to polyfill it), but works in modern ones.
It looks like this:
In the examples above variables were declared right in the assignment: let {…} = {…} . Of course, we could use existing variables too, without let . But there’s a catch.
This won’t work:
The problem is that JavaScript treats {...} in the main code flow (not inside another expression) as a code block. Such code blocks can be used to group statements, like this:
So here JavaScript assumes that we have a code block, that’s why there’s an error. We want destructuring instead.
To show JavaScript that it’s not a code block, we can wrap the expression in parentheses (...) :
Nested destructuring
If an object or an array contains other nested objects and arrays, we can use more complex left-side patterns to extract deeper portions.
In the code below options has another object in the property size and an array in the property items . The pattern on the left side of the assignment has the same structure to extract values from them:
All properties of options object except extra which is absent in the left part, are assigned to corresponding variables:
Finally, we have width , height , item1 , item2 and title from the default value.
Note that there are no variables for size and items , as we take their content instead.
Smart function parameters
There are times when a function has many parameters, most of which are optional. That’s especially true for user interfaces. Imagine a function that creates a menu. It may have a width, a height, a title, an item list and so on.
Here’s a bad way to write such a function:
In real-life, the problem is how to remember the order of arguments. Usually, IDEs try to help us, especially if the code is well-documented, but still… Another problem is how to call a function when most parameters are ok by default.
That’s ugly. And becomes unreadable when we deal with more parameters.
Destructuring comes to the rescue!
We can pass parameters as an object, and the function immediately destructurizes them into variables:
We can also use more complex destructuring with nested objects and colon mappings:
The full syntax is the same as for a destructuring assignment:
Then, for an object of parameters, there will be a variable varName for the property incomingProperty , with defaultValue by default.
Please note that such destructuring assumes that showMenu() does have an argument. If we want all values by default, then we should specify an empty object:
We can fix this by making {} the default value for the whole object of parameters:
In the code above, the whole arguments object is {} by default, so there’s always something to destructurize.
Destructuring assignment allows for instantly mapping an object or array onto many variables.
The full object syntax:
This means that property prop should go into the variable varName and, if no such property exists, then the default value should be used.
Object properties that have no mapping are copied to the rest object.
The full array syntax:
The first item goes to item1 ; the second goes into item2 , and all the rest makes the array rest .
It’s possible to extract data from nested arrays/objects, for that the left side must have the same structure as the right one.
We have an object:
Write the destructuring assignment that reads:
- name property into the variable name .
- years property into the variable age .
- isAdmin property into the variable isAdmin (false, if no such property)
Here’s an example of the values after your assignment:
The maximal salary
There is a salaries object:
Create the function topSalary(salaries) that returns the name of the top-paid person.
- If salaries is empty, it should return null .
- If there are multiple top-paid persons, return any of them.
P.S. Use Object.entries and destructuring to iterate over key/value pairs.
Open a sandbox with tests.
Open the solution with tests in a sandbox.
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
Array vs Object Destructuring in JavaScript – What’s the Difference?
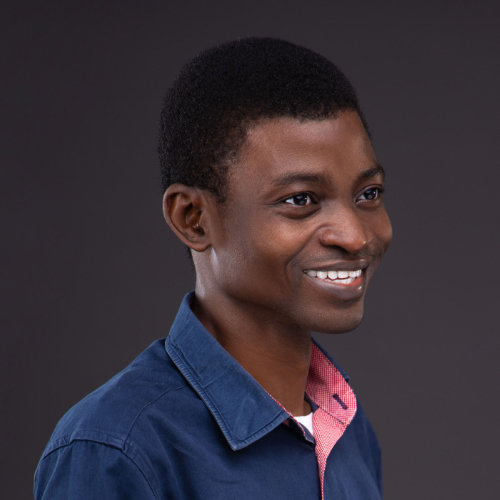
The destructuring assignment in JavaScript provides a neat and DRY way to extract values from your arrays and objects.
This article aims to show you exactly how array and object destructuring assignments work in JavaScript.
So, without any further ado, let’s get started with array destructuring.
What Is Array Destructuring?
Array destructuring is a unique technique that allows you to neatly extract an array’s value into new variables.
For instance, without using the array destructuring assignment technique, you would copy an array’s value into a new variable like so:
Try it on StackBlitz
Notice that the snippet above has a lot of repeated code which is not a DRY ( D on’t R epeat Y ourself) way of coding.
Let’s now see how array destructuring makes things neater and DRYer.
You see, like magic, we’ve cleaned up our code by placing the three new variables (that is, firstName , lastName , and website ) into an array object ( [...] ). Then, we assigned them the profile array's values.
In other words, we instructed the computer to extract the profile array’s values into the variables on the left-hand side of the assignment operator .
Therefore, JavaScript will parse the profile array and copy its first value ( "Oluwatobi" ) into the destructuring array’s first variable ( firstName ).
Likewise, the computer will extract the profile array’s second value ( "Sofela" ) into the destructuring array’s second variable ( lastName ).
Lastly, JavaScript will copy the profile array’s third value ( "codesweetly.com" ) into the destructuring array’s third variable ( website ).
Notice that the snippet above destructured the profile array by referencing it. However, you can also do direct destructuring of an array. Let’s see how.
How to Do Direct Array Destructuring
JavaScript lets you destructure an array directly like so:
Suppose you prefer to separate your variable declarations from their assignments. In that case, JavaScript has you covered. Let’s see how.
How to Use Array Destructuring While Separating Variable Declarations from Their Assignments
Whenever you use array destructuring, JavaScript allows you to separate your variable declarations from their assignments.
Here’s an example:
What if you want "Oluwatobi" assigned to the firstName variable—and the rest of the array items to another variable? How you do that? Let’s find out below.
How to Use Array Destructuring to Assign the Rest of an Array Literal to a Variable
JavaScript allows you to use the rest operator within a destructuring array to assign the rest of a regular array to a variable.
Note: Always use the rest operator as the last item of your destructuring array to avoid getting a SyntaxError .
Now, what if you only want to extract "codesweetly.com" ? Let's discuss the technique you can use below.
How to Use Array Destructuring to Extract Values at Any Index
Here’s how you can use array destructuring to extract values at any index position of a regular array:
In the snippet above, we used commas to skip variables at the destructuring array's first and second index positions.
By so doing, we were able to link the website variable to the third index value of the regular array on the right side of the assignment operator (that is, "codesweetly.com" ).
At times, however, the value you wish to extract from an array is undefined . In that case, JavaScript provides a way to set default values in the destructuring array. Let’s learn more about this below.
How Default Values Work in an Array Destructuring Assignment
Setting a default value can be handy when the value you wish to extract from an array does not exist (or is set to undefined ).
Here’s how you can set one inside a destructuring array:
In the snippet above, we set "Tobi" and "CodeSweetly" as the default values of the firstName and website variables.
Therefore, in our attempt to extract the first index value from the right-hand side array, the computer defaulted to "CodeSweetly" —because only a zeroth index value exists in ["Oluwatobi"] .
So, what if you need to swap firstName ’s value with that of website ? Again, you can use array destructuring to get the job done. Let’s see how.
How to Use Array Destructuring to Swap Variables’ Values
You can use the array destructuring assignment to swap the values of two or more different variables.
Here's an example:
In the snippet above, we used direct array destructuring to reassign the firstName and website variables with the values of the array literal on the right-hand side of the assignment operator.
As such, firstName ’s value will change from "Oluwatobi" to "CodeSweetly" . While website ’s content will change from "CodeSweetly" to "Oluwatobi" .
Keep in mind that you can also use array destructuring to extract values from a regular array to a function’s parameters. Let’s talk more about this below.
How to Use Array Destructuring to Extract Values from an Array to a Function’s Parameters
Here’s how you can use array destructuring to extract an array’s value to a function’s parameter:
In the snippet above, we used an array destructuring parameter to extract the profile array’s values into the getUserBio ’s firstName and lastName parameters.
Note: An array destructuring parameter is typically called a destructuring parameter .
Here’s another example:
In the snippet above, we used two array destructuring parameters to extract the profile array’s values into the getUserBio ’s website and userName parameters.
There are times you may need to invoke a function containing a destructuring parameter without passing any argument to it. In that case, you will need to use a technique that prevents the browser from throwing a TypeError .
Let’s learn about the technique below.
How to Invoke a Function Containing Array Destructuring Parameters Without Supplying Any Argument
Consider the function below:
Now, let’s invoke the getUserBio function without passing any argument to its destructuring parameter:
Try it on CodeSandBox
After invoking the getUserBio function above, the browser will throw an error similar to TypeError: undefined is not iterable .
The TypeError message happens because functions containing a destructuring parameter expect you to supply at least one argument.
So, you can avoid such error messages by assigning a default argument to the destructuring parameter.
Notice in the snippet above that we assigned an empty array as the destructuring parameter’s default argument.
So, let’s now invoke the getUserBio function without passing any argument to its destructuring parameter:
The function will output:
Keep in mind that you do not have to use an empty array as the destructuring parameter’s default argument. You can use any other value that is not null or undefined .
So, now that we know how array destructuring works, let's discuss object destructuring so we can see the differences.
What Is Object Destructuring in JavaScript?
Object destructuring is a unique technique that allows you to neatly extract an object’s value into new variables.
For instance, without using the object destructuring assignment technique, we would extract an object’s value into a new variable like so:
Let’s now see how the object destructuring assignment makes things neater and DRY.
You see, like magic, we’ve cleaned up our code by placing the three new variables into a properties object ( {...} ) and assigning them the profile object’s values.
In other words, we instructed the computer to extract the profile object’s values into the variables on the left-hand side of the assignment operator .
Therefore, JavaScript will parse the profile object and copy its first value ( "Oluwatobi" ) into the destructuring object’s first variable ( firstName ).
Likewise, the computer will extract the profile object’s second value ( "Sofela" ) into the destructuring object’s second variable ( lastName ).
Lastly, JavaScript will copy the profile object’s third value ( "codesweetly.com" ) into the destructuring object’s third variable ( website ).
Keep in mind that in { firstName: firstName, lastName: lastName, website: website } , the keys are references to the profile object’s properties – while the keys’ values represent the new variables.

Alternatively, you can use shorthand syntax to make your code easier to read.
In the snippet above, we shortened { firstName: firstName, age: age, gender: gender } to { firstName, age, gender } . You can learn more about the shorthand technique here .
Observe that the snippets above illustrated how to assign an object’s value to a variable when both the object’s property and the variable have the same name.
However, you can also assign a property’s value to a variable of a different name. Let’s see how.
How to Use Object Destructuring When the Property’s Name Differs from That of the Variable
JavaScript permits you to use object destructuring to extract a property’s value into a variable even if both the property and the variable’s names are different.
In the snippet above, the computer successfully extracted the profile object’s values into the variables named forename , surname , and onlineSite —even though the properties and variables are of different names.
Note: const { firstName: forename } = profile is equivalent to const forename = profile.firstName .
In the snippet above, the computer successfully extracted the profile object’s value into the surname variable—even though the property and variable are of different names.
Note: const { lastName: { familyName: surname } } = profile is equivalent to const surname = profile.lastName.familyName .
Notice that so far, we’ve destructured the profile object by referencing it. However, you can also do direct destructuring of an object. Let’s see how.
How to Do Direct Object Destructuring
JavaScript permits direct destructuring of a properties object like so:
Suppose you prefer to separate your variable declarations from their assignments. In that case, JavaScript has you covered. Let see how.
How to Use Object Destructuring While Separating Variable Declarations from Their Assignments
Whenever you use object destructuring, JavaScript allows you to separate your variable declarations from their assignments.
- Make sure that you encase the object destructuring assignment in parentheses. By so doing, the computer will know that the object destructuring is an object literal, not a block.
- Place a semicolon ( ; ) after the parentheses of an object destructuring assignment. By doing so, you will prevent the computer from interpreting the parentheses as an invocator of a function that may be on the previous line.
What if you want "Oluwatobi" assigned to the firstName variable—and the rest of the object’s values to another variable? How can you do this? Let’s find out below.
How to Use Object Destructuring to Assign the Rest of an Object to a Variable
JavaScript allows you to use the rest operator within a destructuring object to assign the rest of an object literal to a variable.
Note: Always use the rest operator as the last item of your destructuring object to avoid getting a SyntaxError .
At times, the value you wish to extract from a properties object is undefined . In that case, JavaScript provides a way to set default values in the destructuring object. Let’s learn more about this below.
How Default Values Work in an Object Destructuring Assignment
Setting a default value can be handy when the value you wish to extract from an object does not exist (or is set to undefined ).
Here’s how you can set one inside a destructuring properties object:
Therefore, in our attempt to extract the second property’s value from the right-hand side object, the computer defaulted to "CodeSweetly" —because only a single property exists in {firstName: "Oluwatobi"} .
So, what if you need to swap firstName ’s value with that of website ? Again, you can use object destructuring to get the job done. Let’s see how below.
How to Use Object Destructuring to Swap Values
You can use the object destructuring assignment to swap the values of two or more different variables.
The snippet above used direct object destructuring to reassign the firstName and website variables with the values of the object literal on the right-hand side of the assignment operator.
Keep in mind that you can also use object destructuring to extract values from properties to a function’s parameters. Let’s talk more about this below.
How to Use Object Destructuring to Extract Values from Properties to a Function’s Parameters
Here’s how you can use object destructuring to copy a property’s value to a function’s parameter:
In the snippet above, we used an object destructuring parameter to copy the profile object’s values into getUserBio ’s firstName and lastName parameters.
Note: An object destructuring parameter is typically called a destructuring parameter .
In the snippet above, we used two destructuring parameters to copy the profile object’s values into getUserBio ’s website and userName parameters.
Note: If you are unclear about the destructuring parameter above, you may grasp it better by reviewing this section .
How to Invoke a Function Containing Destructured Parameters Without Supplying Any Argument
After invoking the getUserBio function above, the browser will throw an error similar to TypeError: (destructured parameter) is undefined .
Notice that in the snippet above, we assigned an empty object as the destructuring parameter’s default argument.
Keep in mind that you do not have to use an empty object as the destructuring parameter’s default argument. You can use any other value that is not null or undefined .

Wrapping It Up
Array and object destructuring work similarly. The main difference between the two destructuring assignments is this:
- Array destructuring extracts values from an array. But object destructuring extracts values from a JavaScript object.
This article discussed how array and object destructuring works in JavaScript. We also looked at the main difference between the two destructuring assignments.
Thanks for reading!
And here's a useful ReactJS resource:
I wrote a book about React!
- It's beginner’s friendly ✔
- It has live code snippets ✔
- It contains scalable projects ✔
- It has plenty of easy-to-grasp examples ✔
The React Explained Clearly book is all you need to understand ReactJS.
Click Here to Get Your Copy

Read more posts .
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Popular Tutorials
Popular examples, reference materials, learn python interactively, js introduction.
- Getting Started
- JS Variables & Constants
- JS console.log
- JavaScript Data types
- JavaScript Operators
- JavaScript Comments
- JS Type Conversions
JS Control Flow
- JS Comparison Operators
- JavaScript if else Statement
- JavaScript for loop
- JavaScript while loop
- JavaScript break Statement
- JavaScript continue Statement
- JavaScript switch Statement
JS Functions
- JavaScript Function
- Variable Scope
- JavaScript Hoisting
- JavaScript Recursion
- JavaScript Objects
- JavaScript Methods & this
- JavaScript Constructor
- JavaScript Getter and Setter
- JavaScript Prototype
JavaScript Array
- JS Multidimensional Array
- JavaScript String
- JavaScript for...in loop
- JavaScript Number
- JavaScript Symbol
Exceptions and Modules
- JavaScript try...catch...finally
- JavaScript throw Statement
- JavaScript Modules
- JavaScript ES6
- JavaScript Arrow Function
- JavaScript Default Parameters
- JavaScript Template Literals
JavaScript Spread Operator
- JavaScript Map
- JavaScript Set
- Destructuring Assignment
- JavaScript Classes
- JavaScript Inheritance
- JavaScript for...of
- JavaScript Proxies
JavaScript Asynchronous
- JavaScript setTimeout()
- JavaScript CallBack Function
- JavaScript Promise
- Javascript async/await
- JavaScript setInterval()
Miscellaneous
- JavaScript JSON
- JavaScript Date and Time
- JavaScript Closure
- JavaScript this
- JavaScript use strict
- Iterators and Iterables
- JavaScript Generators
- JavaScript Regular Expressions
- JavaScript Browser Debugging
- Uses of JavaScript
JavaScript Tutorials
- JavaScript Array splice()
- JavaScript Array shift()
- JavaScript Array unshift()
JavaScript Multidimensional Array
- JavaScript Array pop()
- JavaScript Array slice()
An array is an object that can store multiple values at once.
In the above example, we created an array to record the age of five students.
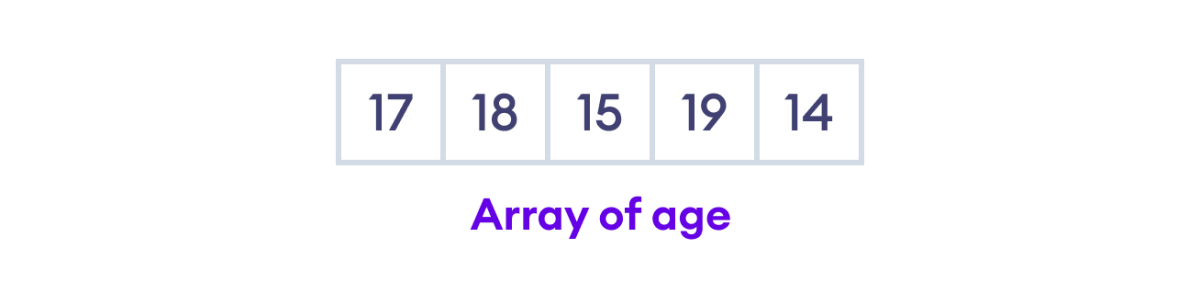
Why Use Arrays?
Arrays allow us to organize related data by grouping them within a single variable.
Suppose you want to store a list of fruits. Using only variables, this process might look like this:
Here, we've only listed a few fruits. But what if we need to store 100 fruits?
For such a case, the easiest solution is to store them in an array.
An array can store many values in a single variable, making it easy to access them by referring to the corresponding index number.
- Create an Array
We can create an array by placing elements inside an array literal [] , separated by commas. For example,
- numbers - Name of the array.
- [10, 30, 40, 60, 80] - Elements of the array.
Here are a few examples of JavaScript arrays:
Note: Unlike many other programming languages, JavaScript allows us to create arrays with mixed data types.
- Access Elements of an Array
Each element of an array is associated with a number called an index , which specifies its position inside the array.
Consider the following array:
Here is the indexing of each element:
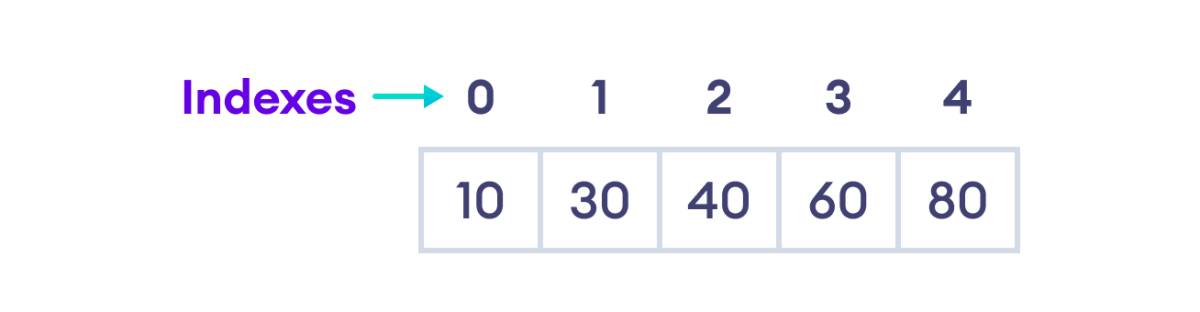
We can use an array index to access the elements of the array.
Code | Description |
---|---|
Accesses the first element . | |
Accesses the second element . | |
Accesses the third element . | |
Accesses the fourth element . | |
Accesses the fifth element . |
Let's look at an example.
Remember: Array indexes always start with 0 , not 1.
- Add Element to an Array
We can add elements to an array using built-in methods like push() and unshift() .
1. Using the push() Method
The push() method adds an element at the end of the array.
2. Using the unshift() Method
The unshift() method adds an element at the beginning of the array.
To learn more, visit Array push() and Array unshift() .
- Change the Elements of an Array
We can add or change elements by accessing the index value. For example,
Here, we changed the array element in index 1 (second element) from work to exercise .
- Remove Elements From an Array
We can remove an element from any specified index of an array using the splice() method.
In this example, we removed the element at index 2 (the third element) using the splice() method.
Notice the following code:
Here, (2, 1) means that the splice() method deletes one element starting from index 2 .
Note: Suppose you want to remove the second, third, and fourth elements. You can use the following code to do so:
To learn more, visit JavaScript Array splice() .
- Array Methods
JavaScript has various array methods to perform useful operations. Some commonly used array methods in JavaScript are:
Method | Description |
---|---|
Joins two or more arrays and returns a result. | |
Converts an array to a string of (comma-separated) array values. | |
Searches an element of an array and returns its position (index). | |
Returns the first value of the array element that passes a given test. | |
Returns the first index of the array element that passes a given test. | |
Calls a function for each element. | |
Checks if an array contains a specified element. | |
Sorts the elements alphabetically in strings and ascending order in numbers. | |
Selects part of an array and returns it as a new array. | |
Removes or replaces existing elements and/or adds new elements. |
To learn more, visit JavaScript Array Methods .
More on Javascript Array
You can also create an array using JavaScript's new keyword. For example,
Note : It's better to create an array using an array literal [] for greater readability and execution speed.
We can remove an element from an array using built-in methods like pop() and shift() .
1. Remove the last element using pop().
2. Remove the first element using shift().
To learn more, visit Array pop() and Array shift() .
We can find the length of an array using the length property. For example,
In JavaScript, arrays are a type of object. However,
- Arrays use numbered indexes to access elements.
- Objects use named indexes (keys) to access values.
Since arrays are objects, the array elements are stored by reference . Hence, when we assign an array to another variable, we are just pointing to the same array in memory.
So, changing one will change the other because they're essentially the same array. For example,
Here, we modified the copied array arr1 , which also modified the original array arr .
- JavaScript forEach
Table of Contents
- Introduction
Sorry about that.
Related Tutorials
JavaScript Tutorial
JavaScript forEach()
JavaScript Set and WeakSet
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
Spread syntax (...)
The spread ( ... ) syntax allows an iterable, such as an array or string, to be expanded in places where zero or more arguments (for function calls) or elements (for array literals) are expected. In an object literal, the spread syntax enumerates the properties of an object and adds the key-value pairs to the object being created.
Spread syntax looks exactly like rest syntax. In a way, spread syntax is the opposite of rest syntax. Spread syntax "expands" an array into its elements, while rest syntax collects multiple elements and "condenses" them into a single element. See rest parameters and rest property .
Description
Spread syntax can be used when all elements from an object or array need to be included in a new array or object, or should be applied one-by-one in a function call's arguments list. There are three distinct places that accept the spread syntax:
- Function arguments list ( myFunction(a, ...iterableObj, b) )
- Array literals ( [1, ...iterableObj, '4', 'five', 6] )
- Object literals ( { ...obj, key: 'value' } )
Although the syntax looks the same, they come with slightly different semantics.
Only iterable values, like Array and String , can be spread in array literals and argument lists. Many objects are not iterable, including all plain objects that lack a Symbol.iterator method:
On the other hand, spreading in object literals enumerates the own properties of the value. For typical arrays, all indices are enumerable own properties, so arrays can be spread into objects.
All primitives can be spread in objects. Only strings have enumerable own properties, and spreading anything else doesn't create properties on the new object.
When using spread syntax for function calls, be aware of the possibility of exceeding the JavaScript engine's argument length limit. See Function.prototype.apply() for more details.
Spread in function calls
Replace apply().
It is common to use Function.prototype.apply() in cases where you want to use the elements of an array as arguments to a function.
With spread syntax the above can be written as:
Any argument in the argument list can use spread syntax, and the spread syntax can be used multiple times.
Apply for new operator
When calling a constructor with new , it's not possible to directly use an array and apply() , because apply() calls the target function instead of constructing it, which means, among other things, that new.target will be undefined . However, an array can be easily used with new thanks to spread syntax:
Spread in array literals
A more powerful array literal.
Without spread syntax, the array literal syntax is no longer sufficient to create a new array using an existing array as one part of it. Instead, imperative code must be used using a combination of methods, including push() , splice() , concat() , etc. With spread syntax, this becomes much more succinct:
Just like spread for argument lists, ... can be used anywhere in the array literal, and may be used more than once.
Copying an array
You can use spread syntax to make a shallow copy of an array. Each array element retains its identity without getting copied.
Spread syntax effectively goes one level deep while copying an array. Therefore, it may be unsuitable for copying multidimensional arrays. The same is true with Object.assign() — no native operation in JavaScript does a deep clone. The web API method structuredClone() allows deep copying values of certain supported types . See shallow copy for more details.
A better way to concatenate arrays
Array.prototype.concat() is often used to concatenate an array to the end of an existing array. Without spread syntax, this is done as:
With spread syntax this becomes:
Array.prototype.unshift() is often used to insert an array of values at the start of an existing array. Without spread syntax, this is done as:
With spread syntax, this becomes:
Note: Unlike unshift() , this creates a new arr1 , instead of modifying the original arr1 array in-place.
Conditionally adding values to an array
You can make an element present or absent in an array literal, depending on a condition, using a conditional operator .
When the condition is false , we spread an empty array, so that nothing gets added to the final array. Note that this is different from the following:
In this case, an extra undefined element is added when isSummer is false , and this element will be visited by methods such as Array.prototype.map() .
Spread in object literals
Copying and merging objects.
You can use spread syntax to merge multiple objects into one new object.
A single spread creates a shallow copy of the original object (but without non-enumerable properties and without copying the prototype), similar to copying an array .
Overriding properties
When one object is spread into another object, or when multiple objects are spread into one object, and properties with identical names are encountered, the property takes the last value assigned while remaining in the position it was originally set.
Conditionally adding properties to an object
You can make an element present or absent in an object literal, depending on a condition, using a conditional operator .
The case where the condition is false is an empty object, so that nothing gets spread into the final object. Note that this is different from the following:
In this case, the watermelon property is always present and will be visited by methods such as Object.keys() .
Because primitives can be spread into objects as well, and from the observation that all falsy values do not have enumerable properties, you can simply use a logical AND operator:
In this case, if isSummer is any falsy value, no property will be created on the fruits object.
Comparing with Object.assign()
Note that Object.assign() can be used to mutate an object, whereas spread syntax can't.
In addition, Object.assign() triggers setters on the target object, whereas spread syntax does not.
You cannot naively re-implement the Object.assign() function through a single spreading:
In the above example, the spread syntax does not work as one might expect: it spreads an array of arguments into the object literal, due to the rest parameter. Here is an implementation of merge using the spread syntax, whose behavior is similar to Object.assign() , except that it doesn't trigger setters, nor mutates any object:
Specifications
Specification |
---|
Browser compatibility
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Rest parameters
- Rest property
- Function.prototype.apply()
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
How to Merge Array of Objects by Property using Lodash?
Merging an array of objects by property using Lodash is a common operation when you need to consolidate data that share a common identifier. For example, if you have a list of user objects and you want to combine them into a single object based on a unique property, Lodash provides useful functions to simplify this process.
Below are different approaches to merging objects in an array by a specific property using Lodash:
Table of Content
Using _.merge( ) and _.groupBy ( )
Using _.keyby ( ) and _.assign ( ).
In this approach, we first group the objects in the array based on a common property using _.groupBy ( ) . Then, we merge the objects within each group using _.merge ( ). This method is useful when we have multiple objects that should be combined into one based on a shared key.
Example: This code groups an array of student objects by their id and merges the objects within each group into a single object, consolidating information for each student.
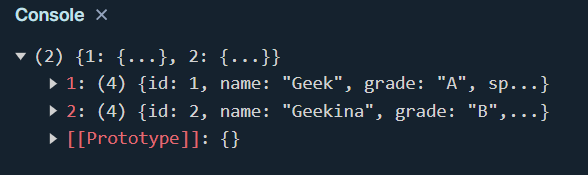
In this approach, we first use _.keyBy ( ) to turn the array of objects into an object, where each key is the value of the specified property. Then, we use _.assign ( ) or _.merge ( ) to combine these objects. This method is efficient and works well when we want to quickly merge objects by a property.
Example: This code keys an array of student objects by their id and merges the keyed objects into a single object, consolidating the information for each student.
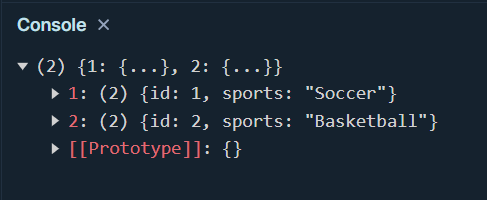
Please Login to comment...
Similar reads.
- Web Technologies
- JavaScript-Lodash
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
How TO - The Spread Operator (...)
Learn how to use the three dots operator (...) a.k.a the spread operator in JavaScript.
The Spread Operator
The JavaScript spread operator ( ... ) expands an iterable (like an array) into more elements.
This allows us to quickly copy all or parts of an existing array into another array:
Assign the first and second items from numbers to variables and put the rest in an array:
The spread operator is often used to extract only what's needed from an array:
We can use the spread operator with objects too:
Notice the properties that did not match were combined, but the property that did match, color , was overwritten by the last object that was passed, updateMyVehicle . The resulting color is now yellow.
See also : JavaScript ES6 Tutorial .

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Is there a "null coalescing" operator in JavaScript?
Is there a null coalescing operator in Javascript?
For example, in C#, I can do this:
The best approximation I can figure out for Javascript is using the conditional operator:
Which is sorta icky IMHO. Can I do better?
- null-coalescing-operator
- null-coalescing
- 39 note from 2018: x ?? y syntax is now in stage 1 proposal status - nullish coalescing – Aprillion Commented Jun 3, 2018 at 9:00
- 4 There is now a Babel plugin which incorporates this exact syntax. – Jonathan Sudiaman Commented Jul 29, 2019 at 14:56
- 11 Note from 2019: now is stage 3 status! – Daniel Schaffer Commented Jul 29, 2019 at 16:19
- 5 Note from January 2020: Nullish coalescing operator is available natively in Firefox 72 but optional chaining operator is still not. – Kir Kanos Commented Jan 8, 2020 at 13:49
- 6 The nullish coalescing operator ( x ?? y ) and optional chaining operator ( user.address?.street ) are now both Stage 4. Here's a good description about what that means: 2ality.com/2015/11/tc39-process.html#stage-4%3A-finished . – Mass Dot Net Commented Feb 21, 2020 at 14:40
19 Answers 19
JavaScript now supports the nullish coalescing operator (??) . It returns its right-hand-side operand when its left-hand-side operand is null or undefined , and otherwise returns its left-hand-side operand.
Please check compatibility before using it.
The JavaScript equivalent of the C# null coalescing operator ( ?? ) is using a logical OR ( || ):
There are cases (clarified below) that the behaviour won't match that of C#, but this is the general, terse way of assigning default/alternative values in JavaScript.
Clarification
Regardless of the type of the first operand, if casting it to a Boolean results in false , the assignment will use the second operand. Beware of all the cases below:
This means:
- 56 Strings like "false", "undefined", "null", "0", "empty", "deleted" are all true since they are non-empty strings. – some Commented Jan 25, 2009 at 5:25
- 111 Of note is that || returns the first "truey" value or the last "falsey" one (if none can evaluate to true) and that && works in the opposite way: returning the last truey value or the first falsey one. – Justin Johnson Commented Oct 25, 2010 at 2:09
- 2 @JustinJohnson makes a good point. This answer compares all three: ?? vs || vs && . – Inigo Commented Dec 28, 2021 at 22:07
this solution works like the SQL coalesce function, it accepts any number of arguments, and returns null if none of them have a value. It behaves like the C# ?? operator in the sense that "", false, and 0 are considered NOT NULL and therefore count as actual values. If you come from a .net background, this will be the most natural feeling solution.

- 16 Apologies for such a late addition, but I just wanted to note for completeness that this solution does have the caveat that it has no short-circuit evaluation; if your arguments are function calls then they will all be evaluated regardless of whether their value is returned, which differs from the behaviour of the logical OR operator, so is worth noting. – Haravikk Commented Nov 15, 2017 at 11:45
- I tried to integrate the comment from Haravikk but the edit queue is full now. – Valerio Bozz Commented Jun 20 at 14:39
Yes, it is coming soon. See proposal here and implementation status here .
It looks like this:
If || as a replacement of C#'s ?? isn't good enough in your case, because it swallows empty strings and zeros, you can always write your own function:
- 1 alert(null || '') still alerts an empty string, and I think I actually like that alert('' || 'blah') alerts blah rather than an empty string - good to know though! (+1) – Daniel Schaffer Commented Jan 24, 2009 at 18:56
- 2 I think I might actually prefer defining a function that returns false if (strictly) null/undefined and true otherwise - using that with a logical or; it could be more readable than many nested functions calls. e.g. $N(a) || $N(b) || $N(c) || d is more readable than $N($N($N(a, b), c), d) . – Bob Commented Nov 28, 2013 at 6:13
Nobody has mentioned in here the potential for NaN , which--to me--is also a null-ish value. So, I thought I'd add my two-cents.
For the given code:
If you were to use the || operator, you get the first non-false value:
If you use the new ?? (null coalescing) operator, you will get c , which has the value: NaN
Neither of these seem right to me. In my own little world of coalesce logic, which may differ from your world, I consider undefined, null, and NaN as all being "null-ish". So, I would expect to get back d (zero) from the coalesce method.
If anyone's brain works like mine, and you want to exclude NaN , then this custom coalesce method (unlike the one posted here ) will accomplish that:
For those who want the code as short as possible, and don't mind a little lack of clarity, you can also use this as suggested by @impinball. This takes advantage of the fact that NaN is never equal to NaN. You can read up more on that here: Why is NaN not equal to NaN?

- Best practices - treat arguments as array-like, take advantage of NaN !== NaN ( typeof + num.toString() === 'NaN' is redundant), store current argument in variable instead of arguments[i] . – Claudia Commented Aug 17, 2015 at 0:54
?? vs || vs &&
None of the other answers compares all three of these. Since Justin Johnson's comment has so many votes, and since double question mark vs && in javascript was marked a duplicate of this one, it makes sense to include && in an answer.
First in words, inspired by Justin Johnson's comment:
|| returns the first "truey" value, else the last value whatever it is.
&& returns the first "falsey" value, else the last value whatever it is.
?? returns the first non-null, non-undefined value, else the last value, whatever it is.
Then, demonstrated in live code:
let F1, F2 = null, F3 = 0, F4 = '', F5 = parseInt('Not a number (NaN)'), T1 = 3, T2 = 8 console.log( F1 || F2 || F3 || F4 || F5 || T1 || T2 ) // 3 (T1) console.log( F1 || F2 || F3 || F4 || F5 ) // NaN (F5) console.log( T1 && T2 && F1 && F2 && F3 && F4 && F5 ) // undefined (F1) console.log( T1 && T2 ) // 8 (T2) console.log( F1 ?? F2 ?? F3 ?? F4 ?? F5 ?? T1 ) // 0 (F3) console.log( F1 ?? F2) // null (F2)
Logical nullish assignment, 2020+ solution
A new operator is currently being added to the browsers, ??= . This combines the null coalescing operator ?? with the assignment operator = .
NOTE: This is not common in public browser versions yet . Will update as availability changes.
??= checks if the variable is undefined or null, short-circuiting if already defined. If not, the right-side value is assigned to the variable.
Basic Examples
Object/array examples.
Browser Support Jan '22 - 89%
Mozilla Documentation
Yes, and its proposal is Stage 4 now. This means that the proposal is ready for inclusion in the formal ECMAScript standard. You can already use it in recent desktop versions of Chrome, Edge and Firefox, but we will have to wait for a bit longer until this feature reaches cross-browser stability.
Have a look at the following example to demonstrate its behavior:
// note: this will work only if you're running latest versions of aforementioned browsers const var1 = undefined; const var2 = "fallback value"; const result = var1 ?? var2; console.log(`Nullish coalescing results in: ${result}`);
Previous example is equivalent to:
const var1 = undefined; const var2 = "fallback value"; const result = (var1 !== null && var1 !== undefined) ? var1 : var2; console.log(`Nullish coalescing results in: ${result}`);
Note that nullish coalescing will not threat falsy values the way the || operator did (it only checks for undefined or null values), hence the following snippet will act as follows:
// note: this will work only if you're running latest versions of aforementioned browsers const var1 = ""; // empty string const var2 = "fallback value"; const result = var1 ?? var2; console.log(`Nullish coalescing results in: ${result}`);
For TypesScript users, starting off TypeScript 3.7 , this feature is also available now.

- This is gigantic! – Gerard ONeill Commented Oct 9, 2021 at 0:48
After reading your clarification, @Ates Goral's answer provides how to perform the same operation you're doing in C# in JavaScript.
@Gumbo's answer provides the best way to check for null; however, it's important to note the difference in == versus === in JavaScript especially when it comes to issues of checking for undefined and/or null .
There's a really good article about the difference in two terms here . Basically, understand that if you use == instead of === , JavaScript will try to coalesce the values you're comparing and return what the result of the comparison after this coalescence.
beware of the JavaScript specific definition of null. there are two definitions for "no value" in javascript. 1. Null: when a variable is null, it means it contains no data in it, but the variable is already defined in the code. like this:
in such case, the type of your variable is actually Object. test it.
Undefined: when a variable has not been defined before in the code, and as expected, it does not contain any value. like this:
if such case, the type of your variable is 'undefined'.
notice that if you use the type-converting comparison operator (==), JavaScript will act equally for both of these empty-values. to distinguish between them, always use the type-strict comparison operator (===).
- 2 Actually, null is a value. It's a special value of type Object. A variable being set to null means it contains data, the data being a reference to the null object. A variable can be defined with value undefined in your code. This is not the same as the variable not being declared. – Ates Goral Commented Jan 24, 2009 at 18:54
- 1 The actual difference between a variable being declared or not: alert(window.test)/*undefined*/; alert("test" in window)/*false*/; window.test = undefined; alert(window.test)/*undefined*/; alert("test" in window)/*true*/; for (var p in window) {/*p can be "test"*/} – Ates Goral Commented Jan 24, 2009 at 18:59
- 2 however (a bit paradoxal) you can define a variable with the undefined value var u = undefined; – serge Commented Sep 11, 2015 at 13:08
- 1 @AtesGoral re null. While what you say is true, by convention , "null" represents "the absence of (useful) data" . Hence it is considered to be "no data". And lets not forget that this is an answer to a question about "a null coalescing operator"; in this context, null is definitely treated as "no data" - regardless of how it is represented internally. – ToolmakerSteve Commented Oct 7, 2019 at 20:31
There are two items here:
const foo = '' || 'default string';
console.log(foo); // output is 'default string'
- Nullish coalescing operator
const foo = '' ?? 'default string';
console.log(foo); // output is empty string i.e. ''
The nullish coalescing operator (??) is a logical operator that returns its right-hand side operand when its left-hand side operand is null or undefined, and otherwise returns its left-hand side operand.
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Nullish_coalescing_operator
- 1 Before the release of the operator, this talk was necessary. But since your answer is just inferior to and much later in time than @faithful's answer, I'd argue you had too much talk. – Gerard ONeill Commented Oct 9, 2021 at 0:53
Note that React's create-react-app tool-chain supports the null-coalescing since version 3.3.0 (released 5.12.2019) . From the release notes:
Optional Chaining and Nullish Coalescing Operators We now support the optional chaining and nullish coalescing operators! // Optional chaining a?.(); // undefined if `a` is null/undefined b?.c; // undefined if `b` is null/undefined // Nullish coalescing undefined ?? 'some other default'; // result: 'some other default' null ?? 'some other default'; // result: 'some other default' '' ?? 'some other default'; // result: '' 0 ?? 300; // result: 0 false ?? true; // result: false
This said, in case you use create-react-app 3.3.0+ you can start using the null-coalesce operator already today in your React apps.
It will hopefully be available soon in Javascript, as it is in proposal phase as of Apr, 2020. You can monitor the status here for compatibility and support - https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Nullish_coalescing_operator
For people using Typescript, you can use the nullish coalescing operator from Typescript 3.7
From the docs -
You can think of this feature - the ?? operator - as a way to “fall back” to a default value when dealing with null or undefined . When we write code like let x = foo ?? bar(); this is a new way to say that the value foo will be used when it’s “present”; but when it’s null or undefined , calculate bar() in its place.
Need to support old browser and have a object hierarchy
may use this,
ECMAScript 2021 enabled two new features:
- Nullish coalescing operator (??) which is a logical operator that returns its right-hand side operand when its left-hand side operand is either null or undefined, and otherwise returns its left-hand side operand.
let b = undefined ?? 5; console.log(b); // 5
- Logical nullish assignment (x ??= y) operator which only assigns if x has a nullish value (null or undefined).
const car = {speed : 20}; car.speed ??= 5; console.log(car.speed); car.name ??= "reno"; console.log(car.name);
More about Logical nullish assignment can be found here https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Logical_nullish_assignment
More about Nullish coalescing operator can be found here https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Nullish_coalescing_operator

Now it has full support in latest version of major browsers like Chrome, Edge, Firefox , Safari etc. Here's the comparison between the null operator and Nullish Coalescing Operator
Those who are using Babel, need to upgrade to the latest version to use nullish coalescing (??):
Babel 7.8.0 supports the new ECMAScript 2020 features by default: you don't need to enable individual plugins for nullish coalescing (??), optional chaining (?.) and dynamic import() anymore with preset-env
From https://babeljs.io/blog/2020/01/11/7.8.0
Chain multiple values / several values
- "short circuit" is enabled: do not evaluate any further if one of the first values is valid
- that means order matters, the most left values are prioritized

I was trying to check if an input is null and then use the value accordingly. This is my code.
So if inputValue is null then valueToBeConsidered = falseCondition and if inputValue has a value then valueToBeConsidered = trueCondition
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged javascript operators null-coalescing-operator null-coalescing or ask your own question .
- The Overflow Blog
- Ryan Dahl explains why Deno had to evolve with version 2.0
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- Feedback requested: How do you use tag hover descriptions for curating and do...
- What does a new user need in a homepage experience on Stack Overflow?
Hot Network Questions
- Retroactively specifying `-only` or `-or-later` for GPLv2 in an adopted project
- Why is global state hard to test? Doesn't setting the global state at the beginning of each test solve the problem?
- Linear Regulator Pass Transistor vs Parallel Regulators
- How old were Phineas and Ferb? What year was it?
- Bending moment equation explanation
- bash script quoting frustration
- How do we know for sure that the dedekind axiom is logically independent of the other axioms?
- C - mini string lib
- Parallel use of gerund phrases and noun phrases
- How can rotate an object about a specific point that I know the coordinates of
- How to remove files which confirm to a certain number pattern
- How can I push back on my co-worker's changes that I disagree with?
- Fitting 10 pieces of pizza in a box
- Pairing and structuring elements from a JSON array, with jq
- How can I put node of a forest correctly?
- Why do only 2 USB cameras work while 4 USB cameras cannot stream at once?
- Can I repair these deck support posts, or do I need to replace them?
- "Knocking it out of the park" sports metaphor American English vs British English?
- What is the difference between an `.iso` OS for a network and an `.iso` OS for CD?
- Sun rise on Venus from East or West (North as North Eclliptic Pole)
- block-structure matrix
- Does the overall mAh of the battery add up when batteries are parallel?
- In Moon, why does Sam ask GERTY to activate a third clone before the rescue team arrives?
- Is it possible for a company to dilute my shares to the point they are insignificant

IMAGES
COMMENTS
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
Unpacking values from a regular expression match. When the regular expression exec() method finds a match, it returns an array containing first the entire matched portion of the string and then the portions of the string that matched each parenthesized group in the regular expression. Destructuring assignment allows you to unpack the parts out of this array easily, ignoring the full match if ...
When your "test" function returns, you're correct that "names" is "gone". However, its value is not, because it's been assigned to a global variable. The value of the "names" local variable was a reference to an array object. That reference was copied into the global variable, so now that global variable also contains a reference to the array object.
Use the correct assignment operator that will result in x being 15 (same as x = x + y ). Start the Exercise. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more. At a high level, an expression is a valid unit of code that resolves to a value. There are two types of expressions: those that have side effects (such as assigning values) and those that ...
An assignment operator ( =) assigns a value to a variable. The syntax of the assignment operator is as follows: let a = b; Code language: JavaScript (javascript) In this syntax, JavaScript evaluates the expression b first and assigns the result to the variable a. The following example declares the counter variable and initializes its value to zero:
In this tutorial, you will learn about all the different assignment operators in javascript and how to use them in javascript. Assignment Operators. In javascript, there are 16 different assignment operators that are used to assign value to the variable. It is shorthand of other operators which is recommended to use.
It's called "destructuring assignment," because it "destructurizes" by copying items into variables. However, the array itself is not modified. It's just a shorter way to write: // let [firstName, surname] = arr; let firstName = arr [0]; let surname = arr [1]; Ignore elements using commas.
If you need to remove one or more elements from a specific position of an array, you can use the splice() method. The first parameter of splice() is the starting index, while the second is the number of items to remove from the array. So .splice(1, 3) means "start at index = 1 and remove 3 elements".
@cameronjonesweb Actually, what it does is create a new array with the same contents as ar1, append ar2 and then return the new array. Just leave out the assignment part (ar1 = ...) from that line of code and you'll see that the original ar1 did not in fact change. If you want to avoid making a copy, you'll need push.Don't believe me, believe the docs: "The concat() method returns a new array ...
The destructuring assignment syntax unpack object properties into variables: let {firstName, lastName} = person; It can also unpack arrays and any other iterables: let [firstName, lastName] = person;
JavaScript Exponentiation Assignment Operator in JavaScript is represented by "**=". This operator is used to raise the value of the variable to the power of the operand which is right. This can also be explained as the first variable is the power of the second operand. The exponentiation operator is equal to Math.pow(). Syntax: a **= b or a = a **
Javascript operators are used to perform different types of mathematical and logical computations. Examples: The Assignment Operator = assigns values. The Addition Operator + adds values. The Multiplication Operator * multiplies values. The Comparison Operator > compares values
This example shows three ways to create new array: first using array literal notation, then using the Array() constructor, and finally using String.prototype.split() to build the array from a string. js. // 'fruits' array created using array literal notation. const fruits = ["Apple", "Banana"];
Oluwatobi Sofela. The destructuring assignment in JavaScript provides a neat and DRY way to extract values from your arrays and objects. This article aims to show you exactly how array and object destructuring assignments work in JavaScript. So, without any further ado, let's get started with array destructuring.
In JavaScript, an array is an object that can store multiple values at once. In this tutorial, you will learn about JavaScript arrays with the help of examples. ... JavaScript Spread Operator; JavaScript Map; JavaScript Set; Destructuring Assignment; JavaScript Classes; ... when we assign an array to another variable, we are just pointing to ...
The simple answer is that JavaScript allows access to children of an Object via the square brackets. So you could define your class: MyClass = function(){. // Set some defaults that belong to the class via dot syntax or array syntax. this.some_property = 'my value is a string';
JavaScript Exponentiation Assignment Operator in JavaScript is represented by "**=". This operator is used to raise the value of the variable to the power of the operand which is right. This can also be explained as the first variable is the power of the second operand. The exponentiation operator is equal to Math.pow(). Syntax: a **= b or a = a **
Creating an Array. Using an array literal is the easiest way to create a JavaScript Array. Syntax: const array_name = [ item1, item2, ... ]; It is a common practice to declare arrays with the const keyword. Learn more about const with arrays in the chapter: JS Array Const.
The spread (...) syntax allows an iterable, such as an array or string, to be expanded in places where zero or more arguments (for function calls) or elements (for array literals) are expected. In an object literal, the spread syntax enumerates the properties of an object and adds the key-value pairs to the object being created. Spread syntax looks exactly like rest syntax.
In javascript, when I use the assignment operator to assign one object to another, does it copy the values from one to the another, or do they both now point to the same data?. Or does the assignment operator do anything in this case?
In this article, we will learn about the Multiple inline styles. Multiple inline styles can be merged in two ways both ways are described below: Table of Content Using Spread operator:Using Object.assign(): Method 1: Using Spread Operator:The ... operator is called a spread operator. The spread operator in this case concatenates both the objects in
Example. Assign the first and second items from numbers to variables and put the rest in an array: const numbersOne = [1, 2, 3]; const numbersTwo = [4, 5, 6]; const numbersCombined = [...numbersOne, ...numbersTwo]; Try it Yourself ». The spread operator is often used to extract only what's needed from an array:
beware of the JavaScript specific definition of null. there are two definitions for "no value" in javascript. 1. Null: when a variable is null, it means it contains no data in it, but the variable is already defined in the code. like this: var myEmptyValue = 1; myEmptyValue = null;