PHP Tutorial
Php advanced, mysql database, php examples, php reference, php error handling.
Error handling in PHP is simple. An error message with filename, line number and a message describing the error is sent to the browser.
When creating scripts and web applications, error handling is an important part. If your code lacks error checking code, your program may look very unprofessional and you may be open to security risks.
This tutorial contains some of the most common error checking methods in PHP.
We will show different error handling methods:
- Simple "die()" statements
- Custom errors and error triggers
- Error reporting

Basic Error Handling: Using the die() function
The first example shows a simple script that opens a text file:
If the file does not exist you might get an error like this:
To prevent the user from getting an error message like the one above, we test whether the file exist before we try to access it:
Now if the file does not exist you get an error like this:
The code above is more efficient than the earlier code, because it uses a simple error handling mechanism to stop the script after the error.
However, simply stopping the script is not always the right way to go. Let's take a look at alternative PHP functions for handling errors.
Advertisement
Creating a Custom Error Handler
Creating a custom error handler is quite simple. We simply create a special function that can be called when an error occurs in PHP.
This function must be able to handle a minimum of two parameters (error level and error message) but can accept up to five parameters (optionally: file, line-number, and the error context):
Error Report levels
These error report levels are the different types of error the user-defined error handler can be used for:
Now lets create a function to handle errors:
The code above is a simple error handling function. When it is triggered, it gets the error level and an error message. It then outputs the error level and message and terminates the script.
Now that we have created an error handling function we need to decide when it should be triggered.
Set Error Handler
The default error handler for PHP is the built in error handler. We are going to make the function above the default error handler for the duration of the script.
It is possible to change the error handler to apply for only some errors, that way the script can handle different errors in different ways. However, in this example we are going to use our custom error handler for all errors:
Since we want our custom function to handle all errors, the set_error_handler() only needed one parameter, a second parameter could be added to specify an error level.
Testing the error handler by trying to output variable that does not exist:
The output of the code above should be something like this:
Trigger an Error
In a script where users can input data it is useful to trigger errors when an illegal input occurs. In PHP, this is done by the trigger_error() function.
In this example an error occurs if the "test" variable is bigger than "1":
An error can be triggered anywhere you wish in a script, and by adding a second parameter, you can specify what error level is triggered.
Possible error types:
- E_USER_ERROR - Fatal user-generated run-time error. Errors that can not be recovered from. Execution of the script is halted
- E_USER_WARNING - Non-fatal user-generated run-time warning. Execution of the script is not halted
- E_USER_NOTICE - Default. User-generated run-time notice. The script found something that might be an error, but could also happen when running a script normally
In this example an E_USER_WARNING occurs if the "test" variable is bigger than "1". If an E_USER_WARNING occurs we will use our custom error handler and end the script:
Now that we have learned to create our own errors and how to trigger them, lets take a look at error logging.
Error Logging
By default, PHP sends an error log to the server's logging system or a file, depending on how the error_log configuration is set in the php.ini file. By using the error_log() function you can send error logs to a specified file or a remote destination.
Sending error messages to yourself by e-mail can be a good way of getting notified of specific errors.
Send an Error Message by E-Mail
In the example below we will send an e-mail with an error message and end the script, if a specific error occurs:
And the mail received from the code above looks like this:
This should not be used with all errors. Regular errors should be logged on the server using the default PHP logging system.

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Can't use function return value in write context
I’m testing some form elements to make sure they have been filled in, just for the absence of a blank string essentially …
I usually test for an empty string which always makes me cringe a wee bit just in case I should find myself writing similar thing in my sql statements (instead of testing for null and so on) and thereby invoking the wrath of the sql gods.
I have also embraced NULL objects, they are clever things too.
Anyhow, I usually do something like this: (given $x is an array of form elements).
So I thought to myself try this, which seems more explicit:
Then blimey, up jumps this fatal error which is unfamiliar to me!
PHP Fatal error: Can’t use function return value in write context
Yeah, I have read it, but can someone walk me through exactly why it appears? What was doing the writing?
Garh! passed the 5000 posts mark (again) and didn’t celebrate it …
From what I remember (I have encountered it too) It has to do with the way empty works…
empty checks if a variable is empty. Since trim returns just a value, empty can not deal with it… it s internal workings of empty() I m afraid…
doh! http://php.net/manual/en/function.empty.php
gives trim even as an example… stupid me. so I guess that you knew what I was saying
http://php.net/manual/en/language.types.boolean.php: FALSE: the empty string, and the string “0”
so you could also do:

If I’m not mistaken it used to give that error when you tried
I agree with this. trim() returns a value. empty() doesn’t like that. If you put in another function that returns a value, you get the same error.
If you have such conditions for checking your variables, why not write up a custom function to check them?
Thanks for the replies.

Your hypothesis is the best (only?) yet, so shall try and internalise it in the hope of one day really understanding it.
Meanwhile I shall treat empty() with a bit more respect - I wonder if there are other PHP functions which behave similarly?
To reiterate, I know how to check my variables, when experimenting around trying to ‘improve’ my tests I fell upon this new (to me) error.
The full context is : In an Ajax framework whereupon an action is taken do a cursory check to make sure the user did not leave a text field empty. It is not supposed to replace a proper filtering or validation operation - just whack up an alert box.
I have little doubt that you know better what you are doing than me. You seem quite on top of things. I meant no offense, I was just throwing the option out there.
I’ll give that a shot.
[color=darkred]empty()[/color] and [color=darkred]isset()[/color] are treated in very much the same way by PHP. They’re both language constructs, which means they operate at a much lower level than other things like functions. Because of this, the process is much simpler than with a function.
During the stage where the PHP script is parsed and converted into a list of operations for the zend vm to execute, the parser can spot the [color=darkred]empty(…)[/color] statement (the token sequence that the parser recognises is [color=darkred]T_EMPTY '(' variable ')'[/color] ). The [color=darkred]variable[/color] token there is pretty forgiving in what it will accept, including things like function/method calls as well as variables in their many guises. Due to this forgiving statement (which is a good thing!), code like your [color=darkred]empty(trim(…))[/color] will happily get consumed as (at this stage) a valid call to [color=darkred]empty()[/color] .
Upon seeing the [color=darkred]empty(…)[/color] statement, the parser calls the engine function [color=darkred]zend_do_isset_or_isempty()[/color] . This function examines the statement and decides which opcodes need to be recorded for later (there are different opcodes for checking whether variables/array items/object properties are set or empty). But before that decision is made, a call to a function called [color=darkred]zend_check_writable_variable()[/color] is made. This is the part of the whole procedure which is most relevant to the thread here. Its job is simply to check that whatever was seen in place of the [color=darkred]variable[/color] token earlier is a “writable variable”, or not.
The source code for the function is very simple so, rather than describe it verbosely, here it is:
If you don’t read C, don’t be too put off. All that the function does is trigger a familiar error message if the [color=darkred]variable[/color] was a function/method call.
That’s pretty much it for how/when the “Can’t use function return value in write context” error message gets triggered with [color=darkred]empty()[/color] . As for why it needs to be a variable, well as the manual succinctly puts it, [color=darkred]empty()[/color] 's purpose is to (emphasis mine) “determine whether a variable is considered to be empty.” It is really that simple a reason.
Not functions, however the same “writable variable” check is made… (might not be a complete list, emphasis denotes what is passed to [color=darkred]zend_check_writable_variable()[/color] )
- with [color=darkred]isset()[/color] isset([color=darkgreen][b]pi()[/b][/color])
- with [color=darkred]unset()[/color] unset([color=darkgreen][b]pi()[/b][/color])
- on the left side of an assignment [color=darkgreen][b]pi()[/b][/color] = "cake"
- unary operations [color=darkgreen][b]pi()[/b][/color]++
- array/function references trim( &[color=darkgreen][b]pi()[/b][/color] ) array( "apple" => &[color=darkgreen][b]pi()[/b][/color] )
- with [color=darkred]list()[/color] list([color=darkgreen][b]pi()[/b][/color]) = array('apple')
- with [color=darkred]foreach()[/color] foreach ([color=darkgreen][b]pi()[/b][/color] as $a) foreach ($a as [color=darkgreen][b]pi()[/b][/color]) foreach ($a as $b => [color=darkgreen][b]pi()[/b][/color])
As you can see, all of those occasions are in situations where PHP, for one reason or another, expects to be able to write to (i.e. manipulate) a variable.
Hopefully that helps to clarify things a little. (:
Thank you very much for walking me through what is going on in the background and exposing the need for this to have access to a piece of writable memory.
We are indebted to you for your excellent and well thought out reply. .
Great reply. Isn’t it something that could be trivial to implement though - checking the return values of functions etc - seems like a change that would be useful. Is there a reason it only operates on variables (other than the manual saying so)?
You’re welcome, I don’t post much but like to help when I can.
Presumably, because using [COLOR="Sienna"]![/COLOR] is good enough for non-variables.
Are they equivalent then? Looks like they are, but I had it in my head that empty() returned different results on some data types than comparing to false… I guess not then!
Reposting this old chestnut, the variable tests grid - for any casual onlookers who stumbled across this thread and wondering what is the issue between different comparisons being aired here. Showing its age a bit now, but still a good reference.
Ooh, that’s a handy list.
A little off-topic, but I used to get into trouble with count() myself. I really don’t like it that it returns 1 if you pass it a variable with false as value.
But, if there aren’t any rows in the result set, $rows will end up being false.

Can’t you use $stmt->num_rows for that?
Certainly could do that, but in the past I’ve had a situation where the rows were passed around (and counted) and not the statement itself.
- Language Reference
Table of Contents
- Extending Exceptions
PHP has an exception model similar to that of other programming languages. An exception can be throw n, and caught (" catch ed") within PHP. Code may be surrounded in a try block, to facilitate the catching of potential exceptions. Each try must have at least one corresponding catch or finally block.
If an exception is thrown and its current function scope has no catch block, the exception will "bubble up" the call stack to the calling function until it finds a matching catch block. All finally blocks it encounters along the way will be executed. If the call stack is unwound all the way to the global scope without encountering a matching catch block, the program will terminate with a fatal error unless a global exception handler has been set.
The thrown object must be an instanceof Throwable . Trying to throw an object that is not will result in a PHP Fatal Error.
As of PHP 8.0.0, the throw keyword is an expression and may be used in any expression context. In prior versions it was a statement and was required to be on its own line.
A catch block defines how to respond to a thrown exception. A catch block defines one or more types of exception or error it can handle, and optionally a variable to which to assign the exception. (The variable was required prior to PHP 8.0.0.) The first catch block a thrown exception or error encounters that matches the type of the thrown object will handle the object.
Multiple catch blocks can be used to catch different classes of exceptions. Normal execution (when no exception is thrown within the try block) will continue after that last catch block defined in sequence. Exceptions can be throw n (or re-thrown) within a catch block. If not, execution will continue after the catch block that was triggered.
When an exception is thrown, code following the statement will not be executed, and PHP will attempt to find the first matching catch block. If an exception is not caught, a PHP Fatal Error will be issued with an " Uncaught Exception ... " message, unless a handler has been defined with set_exception_handler() .
As of PHP 7.1.0, a catch block may specify multiple exceptions using the pipe ( | ) character. This is useful for when different exceptions from different class hierarchies are handled the same.
As of PHP 8.0.0, the variable name for a caught exception is optional. If not specified, the catch block will still execute but will not have access to the thrown object.
A finally block may also be specified after or instead of catch blocks. Code within the finally block will always be executed after the try and catch blocks, regardless of whether an exception has been thrown, and before normal execution resumes.
One notable interaction is between the finally block and a return statement. If a return statement is encountered inside either the try or the catch blocks, the finally block will still be executed. Moreover, the return statement is evaluated when encountered, but the result will be returned after the finally block is executed. Additionally, if the finally block also contains a return statement, the value from the finally block is returned.
Global exception handler
If an exception is allowed to bubble up to the global scope, it may be caught by a global exception handler if set. The set_exception_handler() function can set a function that will be called in place of a catch block if no other block is invoked. The effect is essentially the same as if the entire program were wrapped in a try - catch block with that function as the catch .
Note : Internal PHP functions mainly use Error reporting , only modern Object-oriented extensions use exceptions. However, errors can be easily translated to exceptions with ErrorException . This technique only works with non-fatal errors, however. Example #1 Converting error reporting to exceptions <?php function exceptions_error_handler ( $severity , $message , $filename , $lineno ) { throw new ErrorException ( $message , 0 , $severity , $filename , $lineno ); } set_error_handler ( 'exceptions_error_handler' ); ?>
The Standard PHP Library (SPL) provides a good number of built-in exceptions .
Example #2 Throwing an Exception
The above example will output:
Example #3 Exception handling with a finally block
Example #4 Interaction between the finally block and return
Example #5 Nested Exception
Example #6 Multi catch exception handling
Example #7 Omitting the caught variable
Only permitted in PHP 8.0.0 and later.
Example #8 Throw as an expression
Improve This Page
User contributed notes 15 notes.

PHP 8.1: $GLOBALS variable restrictions
$GLOBALS is a special variable in PHP that references all variables in the global scope. $GLOBALS is an associative array, with array keys being the global variable name, and the array key being the global variable. $GLOBALS contains all global variables as well as other super global values such $_SERVER , $_GET , $_ENV , etc. where applicable.
Additionally, the $GLOBALS array was always assigned by reference (prior to PHP 8.1), even if it visibly assigned by-value.
It adds a significant amount of technical complexity to support this $GLOBALS variable behavior, particularly when the $GLOBALS array itself is modified which affects multiple global variables at once.
From PHP 8,1 and later , certain changes to the $GLOBALS array throws fatal errors. In general, modifying individual array elements is allowed , but making mass changes are not allowed .
Restrictions and Changes
All changes that affect the $GLOBALS array itself are not allowed since PHP 8.1. This includes destroying the $GLOBALS array, overwriting it with a new value, array operations, and creating references to $GLOBALS variable.

Mass changes to $GLOBALS are no longer allowed
Overwriting, unsetting, or otherwise making mass changes to the $GLOBALS array is no longer allowed.
For example, the all of the following modifications are allowed prior to PHP 8.1, but results in a fatal error in PHP 8.1 and later:
Referencing $GLOBALS is no longer allowed
Prior to PHP 8.1, it was possible to create a reference to $GLOBALS array, and modify that reference. All changes would then be reflected in the global variables as well.
From PHP 8.1, it is no longer allowed to create references to $GLOBALS variable.
Further, it is no longer allowed to pass $GLOBALS to a function that expects a parameter by-reference.
$GLOBALS['GLOBALS'] no longer exists
Prior to PHP 8.1, $GLOBALS variable contained a reference to itself at $GLOBALS['GLOBALS'] . It no longer exists in PHP 8.1 and later.
Backwards Compatibility Impact
Applications that mass-modify, unset, or populate the $GLOBALS array might need to perform said operations on individual array keys, or otherwise they will result in fatal errors since PHP 8.1.
Further, creating references to the $GLOBALS variable is no longer allowed, and it no longer behaves as if it was assigned when a standard variable is assigned $GLOBALS (i.e. $var = $GLOBALS ).
It is rare for a PHP application to make such changes to the $GLOBALS variable, and more often than not, there is a better approach to achieve the result, either using PSR-7 objects , or using mocking libraries when testing applications.
RFC Discussion Implementation
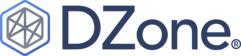
- Manage Email Subscriptions
- How to Post to DZone
- Article Submission Guidelines
- Manage My Drafts
Modern API Management : Dive into APIs’ growing influence across domains, prevalent paradigms, microservices, the role AI plays, and more.
Intro to AI: Dive into the fundamentals of artificial intelligence, machine learning, neural networks, ethics, and more.
Vector databases: Learn all about the specialized VDBMS — its initial setup, data preparation, collection creation, data querying, and more.
Open Source Migration Practices and Patterns : Explore key traits of migrating open-source software and its impact on software development.
- Laravel for Beginners: An Overview of the PHP Framework
- Is PHP Still the Best Language in 2024?
- These 37 Items Are Required for Magento 2 Launch
- PHP 8.2.12 Release that Every Developer Must Know About
- How To Get Started With New Pattern Matching in Java 21
- Real-Time Data Transfer from Apache Flink to Kafka to Druid for Analysis/Decision-Making
- Telemetry Pipelines Workshop: Routing Events With Fluent Bit
- How Scrum Teams Fail Stakeholders
PHP: Fatal error: Can’t use method return value in write context
Join the DZone community and get the full member experience.
Just a quick post to help anyone struggling with this error message, as this issue gets raised from time to time on support forums.
The reason for the error is usually that you’re attempting to use empty or isset on a function instead of a variable. While it may be obvious that this doesn’t make sense for isset(), the same cannot be said for empty(). You simply meant to check if the value returned from the function was an empty value; why shouldn’t you be able to do just that?
The reason is that empty($foo) is more or less syntactic sugar for isset($foo) && $foo. When written this way you can see that the isset() part of the statement doesn’t make sense for functions. This leaves us with simply the $foo part. The solution is to actually just drop the empty() part:
Instead of:
Simply drop the empty construct:
Published at DZone with permission of Mats Lindh , DZone MVB . See the original article here.
Opinions expressed by DZone contributors are their own.
Partner Resources
- About DZone
- Send feedback
- Community research
- Advertise with DZone
CONTRIBUTE ON DZONE
- Become a Contributor
- Core Program
- Visit the Writers' Zone
- Terms of Service
- Privacy Policy
- 3343 Perimeter Hill Drive
- Nashville, TN 37211
- [email protected]
Let's be friends:
PHP RFC: Arbitrary static variable initializers
- Date: 2022-11-06
- Author: Ilija Tovilo, [email protected]
- Status: Implemented
- Target Version: PHP 8.3
- Implementation: https://github.com/php/php-src/pull/9301
PHP allows declaring static variables in all functions. Static variables outlive the function call and are shared across future execution of the function.
The right hand side of the assignment static $i = 1 ; must currently be a constant expression. This means that it can't call functions, use parameters, amongst many other things. This limitation is hard to understand from a user perspective. This RFC suggests lifting this restriction by allowing the static variable initializer to contain arbitrary expressions.
Backwards incompatible changes
- Redeclaring static variables
Currently, redeclaring static variables is allowed, although the semantics are very questionable.
The static variable is overridden at compile time, resulting in both statements referring to the same underlying static variable initializer. This is not useful or intuitive. The new implementation is not compatible with this behavior but would instead result in the first initializer to win. Instead of switching from one dubious behavior to another, redeclaring static variables is disallowed in this RFC and results in a compile time error.
- ReflectionFunction::getStaticVariables()
ReflectionFunction :: getStaticVariables ( ) can be used to inspect a function's static variables and their current values. Currently, PHP automatically evaluates the underlying constant expression and initializes the static variable if the function has never been called. With this RFC this is no longer possible, as static variables may depend on values that are only known at runtime. Instead, the compiler will attempt to resolve the constant expression at compile time. If successful, the value will be embedded in the static variables table. Otherwise it will be initialized to null . After executing the function and assigning to the static variable the contents of the variable will be reflectable through ReflectionFunction :: getStaticVariables ( ) .
From the example above, it becomes more obvious why the initializer $initialValue cannot be evaluated before calling the function.
Other semantics
- Exceptions during initialization
An initializer might throw an exception. In that case, the static variable remains uninitialized and the initializer will be called again in the next execution.
When the static variable declaration overwrites an existing local variable that contains an object with a destructor that throws an exception, the assignment of the static variable is guaranteed to occur before the exception is thrown. This is analogous to assignments to regular variables.
The static variable declaration only runs the initializer if the static variable has not been initialized. When the initializer calls the current function recursively this check will be reached before the function has been initialized. This means that the initializer will be called multiple times. Note though that the assignment to the static variable still only happens once. This is a somewhat technical limitation where the opcode needs to release two values that could both execute user code and thus throw exceptions. Not reassigning the value avoids this issue. However, I cannot imagine a useful scenario for recursive static variable initializers, so semantics here are unlikely to matter.
- What initializers are known at compile-time?
In the discussion the question arose whether static variables depending on other static variables are known at compile time.
The answer is no. In this example it's clear that $a holds the value 0 until the initialization of $b . However, that's not necessarily the case. If $a is modified at any point between the two initializations the initial value of $b also changes.
Here's a quick explanation of how this is implemented: During compilation of static variables the initializer AST is passed to the zend_eval_const_expr function. It traverses the AST and tries to compile-time evaluate all nodes by evaluating their children first and then the node itself if the child nodes were successfully evaluated. If the evaluation fails the nodes stay AST nodes and will again be evaluated at runtime when more information is available (e.g. when class constants are declared). These expressions are currently considered for compile-time constant expression evaluation:
- Literals (strings, ints, bools, etc)
- Binary operations
- Binary comparisons
- Unary operations
- Coalesce operator
- Ternary operator
- Array access ( self :: FOO [ 'bar' ] )
- Array literals
- Magic constants (e.g. __FILE__ )
- Global constants (that are known at compile time)
- Class constants (that are known at compile time)
Voting starts 2023-03-21 and ends 2023-04-04.
As this is a language change, a 2/3 majority is required.
- Show pagesource
- Old revisions
- Back to top
Table of Contents
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
一直报这样的错误 #2
zhong0060 commented Sep 21, 2018
lanlin commented Sep 25, 2018
Sorry, something went wrong.
zhong0060 commented Sep 25, 2018 • edited
Lanlin commented sep 26, 2018 • edited, zhong0060 commented sep 26, 2018 • edited.
| | Demo: | $timers['route/for/timer'] = 10000; // micro seconds | $timers['tests/test/task_timer'] = 10000; // micro seconds | | This means countroller method under 'route/for/timer' will be | execute every per 10s. | */ $timers = ['testsw/task_timer']=10000;
// the end return $timers; Testsw.php <?php /**
Created by Zzz.
Date: 2018/9/21
Time: 下午9:09 */ class Testsw extends CI_Controller{
public function task() { $data = $this->input->post(); log_message('error', var_export($data, true)); }
public function task_timer(){ log_message('error', 'timer works!'); } public function send() { try { \CiSwoole\Core\Client::send( [ 'route' => 'testsw/task', 'params' => ['hope' => 'it works!'], ]); } catch (\Exception $e) { log_message('error', $e->getMessage()); log_message('error', $e->getTraceAsString()); } } }`
lanlin commented Sep 26, 2018
Zhong0060 commented sep 26, 2018.
No branches or pull requests

IMAGES
VIDEO
COMMENTS
Stack Overflow Public questions & answers; Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers; Talent Build your employer brand ; Advertising Reach developers & technologists worldwide; Labs The future of collective knowledge sharing; About the company
Currently in PHP, checking for null leads to deeper nesting and ... = 'baz'; // Assignments can only happen to writable values. It was previously suggested to allow the nullsafe operator in the left hand side of assignments and skip the assignment if the left hand ... // Compiler error: Cannot take reference of a nullsafe chain // 2 takes ...
This will result in the environment variable _SERVER to only expand in the container. If you use a single dollar sign, docker-compose will try to populate the value of the specified environment variable from the surrounding context into the compose file as described in the official compose docs .
Saved searches Use saved searches to filter your results more quickly
Assignment Operators. The basic assignment operator is "=". Your first inclination might be to think of this as "equal to". Don't. It really means that the left operand gets set to the value of the expression on the right (that is, "gets set to").
Assignments can only happen to writable values in adminController.php (line 1236) `` ` 0. Reply . Level 2. ekhlas. Posted 6 years ago. give me your database table detail and model code if you created model. look like there is something missing table creation or in model code. 0. Reply . Level 1.
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
As you can see, all of those occasions are in situations where PHP, for one reason or another, expects to be able to write to (i.e. manipulate) a variable. Hopefully that helps to clarify things a ...
finally. A finally block may also be specified after or instead of catch blocks. Code within the finally block will always be executed after the try and catch blocks, regardless of whether an exception has been thrown, and before normal execution resumes.. One notable interaction is between the finally block and a return statement. If a return statement is encountered inside either the try or ...
The following error is present when trying to view api.php using localhost: Fatal error: Assignments can only happen to writable values in /opt/lampp/htdocs/MyApi/api ...
The readonly modifier can only be applied to typed properties. The reason is that untyped properties have an implicit null default value, which counts as an initializing assignment, and would likely cause confusion.. Thanks to the introduction of the mixed type in PHP 8.0, a readonly property without type constraints can be created using the mixed type: ...
PHP 8.1: `$GLOBALS` variable restrictions. $GLOBALS is a special variable in PHP that references all variables in the global scope.$GLOBALS is an associative array ...
PHP: Fatal error: Can't use method return value in write context. By . Mats Lindh · ... . You simply meant to check if the value returned from the function was an empty value; why shouldn't ...
This means that the initializer will be called multiple times. Note though that the assignment to the static variable still only happens once. This is a somewhat technical limitation where the opcode needs to release two values that could both execute user code and thus throw exceptions. Not reassigning the value avoids this issue.
Tour Start here for a quick overview of the site Help Center Detailed answers to any questions you might have Meta Discuss the workings and policies of this site
Fatal error: Assignments can only happen to writable values in C:\Users\elvarp\Documents\webdev\iceleague\testing.php on line 39 Is this possible in php? Don't have enough knowledge to google this around.
Fatal error: Assignments can only happen to writable values in /home/web/btc/application/config/timers.php on line 16 A PHP Error was encountered Severity: Compile ...
{{ (>_<) }}This version of your browser is not supported. Try upgrading to the latest stable version. Something went seriously wrong.
Fatal errors: These are critical errors - for example, instantiating an object of a non-existent class, or calling a non-existent function. These errors cause the immediate termination of the script, and PHP's default behavior is to display them to the user when they take place.
php - 为什么我的 session 比我设置的时间更快到期? php - 在 mysql 表中上传大约 10,000,000 条记录的大型 CSV 文件也包含重复行. php - while 循环在 PHP 中不能正常工作吗? mysql - 如何将 Apache 访问日志导入 MySQL 表? javascript - JSON 对象和 JSON 文档有什么区别?
This ensures, that my_value won't change it's type to string or something else when you call set_value. But you can still set the value of my_value direct, because it's public. The final step is, to make my_value private and only access my_value over getter/setter methods