Home » JavaScript Tutorial » JavaScript Ternary Operator

JavaScript Ternary Operator
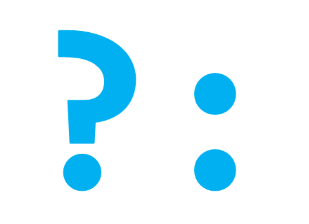
Summary : in this tutorial, you will learn how to use the JavaScript ternary operator to make your code more concise.
Introduction to JavaScript ternary operator
When you want to execute a block if a condition evaluates to true , you often use an if…else statement. For example:
In this example, we show a message that a person can drive if the age is greater than or equal to 16. Alternatively, you can use a ternary operator instead of the if-else statement like this:
Or you can use the ternary operator in an expression as follows:
Here’s the syntax of the ternary operator:
In this syntax, the condition is an expression that evaluates to a Boolean value, either true or false .
If the condition is true , the first expression ( expresionIfTrue ) executes. If it is false, the second expression ( expressionIfFalse ) executes.
The following shows the syntax of the ternary operator used in an expression:
In this syntax, if the condition is true , the variableName will take the result of the first expression ( expressionIfTrue ) or expressionIfFalse otherwise.
JavaScript ternary operator examples
Let’s take some examples of using the ternary operator.
1) Using the JavaScript ternary operator to perform multiple statements
The following example uses the ternary operator to perform multiple operations, where each operation is separated by a comma. For example:
In this example, the returned value of the ternary operator is the last value in the comma-separated list.
2) Simplifying ternary operator example
See the following example:
If the locked is 1, then the canChange variable is set to false , otherwise, it is set to true . In this case, you can simplify it by using a Boolean expression as follows:
3) Using multiple JavaScript ternary operators example
The following example shows how to use two ternary operators in the same expression:
It’s a good practice to use the ternary operator when it makes the code easier to read. If the logic contains many if...else statements, you should avoid using the ternary operators.
- Use the JavaScript ternary operator ( ?: )to make the code more concise.
Popular Tutorials
Popular examples, reference materials, learn python interactively, js introduction.
- Getting Started
- JS Variables & Constants
- JS console.log
- JavaScript Data types
JavaScript Operators
- JavaScript Comments
- JS Type Conversions
JS Control Flow
- JS Comparison Operators
- JavaScript if else Statement
- JavaScript for loop
- JavaScript while loop
- JavaScript break Statement
- JavaScript continue Statement
- JavaScript switch Statement
JS Functions
- JavaScript Function
- Variable Scope
- JavaScript Hoisting
- JavaScript Recursion
- JavaScript Objects
- JavaScript Methods & this
- JavaScript Constructor
- JavaScript Getter and Setter
- JavaScript Prototype
- JavaScript Array
- JS Multidimensional Array
- JavaScript String
- JavaScript for...in loop
- JavaScript Number
- JavaScript Symbol
Exceptions and Modules
- JavaScript try...catch...finally
- JavaScript throw Statement
- JavaScript Modules
- JavaScript ES6
- JavaScript Arrow Function
- JavaScript Default Parameters
- JavaScript Template Literals
- JavaScript Spread Operator
- JavaScript Map
- JavaScript Set
- Destructuring Assignment
- JavaScript Classes
- JavaScript Inheritance
- JavaScript for...of
- JavaScript Proxies
JavaScript Asynchronous
- JavaScript setTimeout()
- JavaScript CallBack Function
- JavaScript Promise
- Javascript async/await
- JavaScript setInterval()
Miscellaneous
- JavaScript JSON
- JavaScript Date and Time
- JavaScript Closure
- JavaScript this
- JavaScript use strict
- Iterators and Iterables
- JavaScript Generators
- JavaScript Regular Expressions
- JavaScript Browser Debugging
- Uses of JavaScript
JavaScript Tutorials
JavaScript if...else Statement
JavaScript Comparison and Logical Operators
JavaScript null and undefined
- JavaScript typeof Operator
- JavaScript while and do...while Loop
JavaScript Ternary Operator
A ternary operator can be used to replace an if..else statement in certain situations. Before you learn about ternary operators, be sure to check the JavaScript if...else tutorial .
- What is a Ternary operator?
A ternary operator evaluates a condition and executes a block of code based on the condition.
Its syntax is:
The ternary operator evaluates the test condition.
- If the condition is true , expression1 is executed.
- If the condition is false , expression2 is executed.
The ternary operator takes three operands, hence, the name ternary operator. It is also known as a conditional operator.
Let's write a program to determine if a student passed or failed in the exam based on marks obtained.
Example: JavaScript Ternary Operator
Suppose the user enters 78 . Then the condition marks >= 40 is checked which evaluates to true . So the first expression pass is assigned to the result variable.
Suppose the use enters 35 . Then the condition marks >= 40 evaluates to false . So the second expression fail is assigned to the result variable.
Ternary Operator Used Instead of if...else
In JavaScript, a ternary operator can be used to replace certain types of if..else statements. For example,
You can replace this code
The output of both programs will be the same.
- Nested ternary operators
You can also nest one ternary operator as an expression inside another ternary operator. For example,
Note : You should try to avoid nested ternary operators whenever possible as they make your code hard to read.
Table of Contents
- Ternary operator used instead if...else
Video: JavaScript Ternary Operators
Sorry about that.
Related Tutorials
JavaScript Tutorial
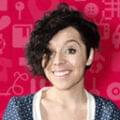
Quick Tip: How to Use the Ternary Operator in JavaScript
Share this article
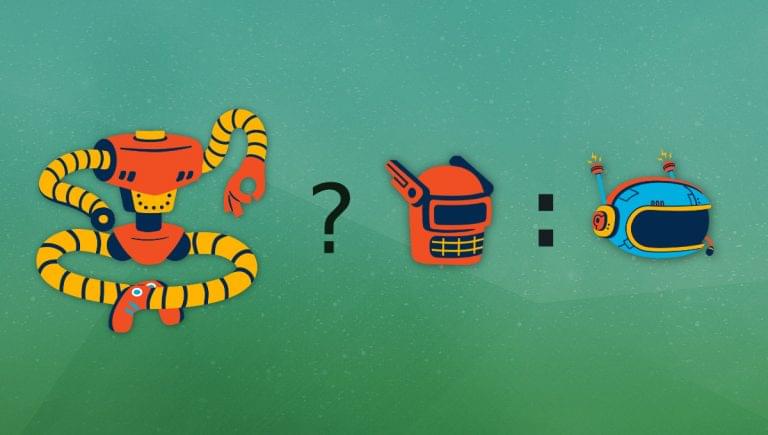
Using the Ternary Operator for Value Assignment
Using the ternary operator for executing expressions, using the ternary operator for null checks, nested conditions, codepen example, faqs on how to use the ternary operator in javascript.
In this tutorial, we’ll explore the syntax of the ternary operator in JavaScript and some of its common uses.
The ternary operator (also known as the conditional operator ) can be used to perform inline condition checking instead of using if...else statements. It makes the code shorter and more readable. It can be used to assign a value to a variable based on a condition, or execute an expression based on a condition.
The ternary operator accepts three operands; it’s the only operator in JavaScript to do that. You supply a condition to test, followed by a questions mark, followed by two expressions separated by a colon. If the condition is considered to be true ( truthy ), the first expression is executed; if it’s considered to be false, the final expression is executed.
It’s used in the following format:
Here, condition is the condition to test. If its value is considered to be true , expr1 is executed. Otherwise, if its value is considered to be false , expr2 is executed.
expr1 and expr2 are any kind of expression. They can be variables, function calls, or even other conditions.
For example:
One of the most common use cases of ternary operators is to decide which value to assign to a variable. Often, a variable’s value might depend on the value of another variable or condition.
Although this can be done using the if...else statement, it can make the code longer and less readable. For example:
In this code example, you first define the variable message . Then, you use the if...else statement to determine the value of the variable.
This can be simply done in one line using the ternary operator:
Ternary operators can be used to execute any kind of expression.
For example, if you want to decide which function to run based on the value of a variable, you can do it like this using the if...else statement:
This can be done in one line using the ternary operator:
If feedback has the value yes , then the sayThankYou function will be called and executed. Otherwise, the saySorry function will be called and executed.
In many cases, you might be handling variables that may or may not have a defined value — for example, when retrieving results from user input, or when retrieving data from a server.
Using the ternary operator, you can check that a variable is not null or undefined just by passing the variable name in the position of the condition operand.
This is especially useful when the variable is an object . If you try to access a property on an object that’s actually null or undefined , an error will occur. Checking that the object is actually set first can help you avoid errors.
In the first part of this code block, book is an object with two properties — name and author . When the ternary operator is used on book , it checks that it’s not null or undefined . If it’s not — meaning it has a value — the name property is accessed and logged into the console. Otherwise, if it’s null, No book is logged into the console instead.
Since book is not null , the name of the book is logged in the console. However, in the second part, when the same condition is applied, the condition in the ternary operator will fail, since book is null . So, “No book” will be logged in the console.
Although ternary operators are used inline, multiple conditions can be used as part of a ternary operator’s expressions. You can nest or chain more than one condition to perform condition checks similar to if...else if...else statements.
For example, a variable’s value may depend on more than one condition. It can be implemented using if...else if...else :
In this code block, you test multiple conditions on the score variable to determine the letter grading of the variable.
These same conditions can be performed using ternary operators as follows:
The first condition is evaluated, which is score < 50 . If it’s true , then the value of grade is F . If it’s false , then the second expression is evaluated which is score < 70 .
This keeps going until either all conditions are false , which means the grade’s value will be A , or until one of the conditions is evaluated to be true and its truthy value is assigned to grade .
In this live example, you can test how the ternary operator works with more multiple conditions.
If you enter a value less than 100, the message “Too Low” will be shown. If you enter a value greater than 100, the message “Too High” will be shown. If you enter 100, the message “Perfect” will be shown.
See the Pen Ternary Operator in JS by SitePoint ( @SitePoint ) on CodePen .
As explained in the examples in this tutorial, the ternary operator in JavaScript has many use cases. In many situations, the ternary operator can increase the readability of our code by replacing lengthy if...else statements.
Related reading:
- 25+ JavaScript Shorthand Coding Techniques
- Quick Tip: How to Use the Spread Operator in JavaScript
- Back to Basics: JavaScript Object Syntax
- JavaScript: Novice to Ninja
What is the Syntax of the Ternary Operator in JavaScript?
The ternary operator in JavaScript is a shorthand way of writing an if-else statement. It is called the ternary operator because it takes three operands: a condition, a result for true, and a result for false. The syntax is as follows: condition ? value_if_true : value_if_false In this syntax, the condition is an expression that evaluates to either true or false. If the condition is true, the operator returns the value_if_true . If the condition is false, it returns the value_if_false .
Can I Use Multiple Ternary Operators in a Single Statement?
Yes, you can use multiple ternary operators in a single statement. This is known as “nesting”. However, it’s important to note that using too many nested ternary operators can make your code harder to read and understand. Here’s an example of how you can nest ternary operators: let age = 15; let beverage = (age >= 21) ? "Beer" : (age < 18) ? "Juice" : "Cola"; console.log(beverage); // Output: "Juice"
Can Ternary Operators Return Functions in JavaScript?
Yes, the ternary operator can return functions in JavaScript. This can be useful when you want to execute different functions based on a condition. Here’s an example: let greeting = (time < 10) ? function() { alert("Good morning"); } : function() { alert("Good day"); }; greeting();
How Does the Ternary Operator Compare to If-Else Statements in Terms of Performance?
In terms of performance, the difference between the ternary operator and if-else statements is negligible in most cases. Both are used for conditional rendering, but the ternary operator can make your code more concise.
Can Ternary Operators be Used Without Else in JavaScript?
No, the ternary operator in JavaScript requires both a true and a false branch. If you don’t need to specify an action for the false condition, consider using an if statement instead.
How Can I Use the Ternary Operator with Arrays in JavaScript?
You can use the ternary operator with arrays in JavaScript to perform different actions based on the condition. Here’s an example: let arr = [1, 2, 3, 4, 5]; let result = arr.length > 0 ? arr[0] : 'Array is empty'; console.log(result); // Output: 1
Can Ternary Operators be Used for Multiple Conditions?
Yes, you can use ternary operators for multiple conditions. However, it can make your code harder to read if overused. Here’s an example: let age = 20; let type = (age < 13) ? "child" : (age < 20) ? "teenager" : "adult"; console.log(type); // Output: "teenager"
Can Ternary Operators be Used in Return Statements?
Yes, you can use ternary operators in return statements. This can make your code more concise. Here’s an example: function isAdult(age) { return (age >= 18) ? true : false; } console.log(isAdult(20)); // Output: true
Can Ternary Operators be Used with Strings in JavaScript?
Yes, you can use ternary operators with strings in JavaScript. Here’s an example: let name = "John"; let greeting = (name == "John") ? "Hello, John!" : "Hello, Stranger!"; console.log(greeting); // Output: "Hello, John!"
Can Ternary Operators be Used with Objects in JavaScript?
Yes, you can use ternary operators with objects in JavaScript. Here’s an example: let user = { name: "John", age: 20 }; let greeting = (user.age >= 18) ? "Hello, Adult!" : "Hello, Kid!"; console.log(greeting); // Output: "Hello, Adult!"
Dianne is SitePoint's newsletter editor. She especiallly loves learning about JavaScript, CSS and frontend technologies.
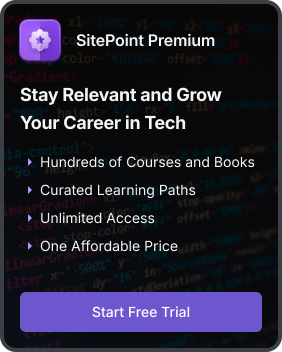
How to Use the Ternary Operator in JavaScript

Conditional statements allow programmers to control the execution of code based on logical conditions. Like many other programming languages, JavaScript features if/else statements that achieve this goal.
What Is The Ternary (Conditional) Operator in JavaScript?
An alternative to the if/else statement, the ternary operator allows JavaScript developers to write concise conditional statements. It is written as “?:” and takes three operands; a logical condition, a value to return if true, and a value to return if false .
But it’s common knowledge among developers that if/else statements with lots of conditions can get messy. They often make scripts unnecessarily long, difficult to debug, and hard to maintain. Fortunately, JavaScript’s ternary operator provides an alternative to the if/else statement, allowing for more concise and maintainable code.
In this article, we’ll write conditional statements with the ternary operator and learn to use it in different situations.
More From Rory Spanton Polynomial Regression: An Introduction
Writing a Simple Expression With the Ternary Operator
The ternary operator gets its name by being the only operator in JavaScript that takes three operands, or parts. The first part of a ternary operation is a logical condition that returns a true or false value. Then, after a question mark , come two expressions, separated by a colon. The first is an expression to execute if the logical condition is true, while the second expression executes if the condition is false. The generic form of the function is below.
The ternary expression is an alternative to the conventional if/else statement. The if/else statement below is equivalent to the ternary operation above.
Because of its similarities to the if/else statement, using a simple ternary operation is straightforward. Let’s say we have a website where users can make an account. Once users sign in, we want to give them a custom greeting. We can create a basic function to do this using the ternary operator.
This function takes a condition called signedIn , which is a true or false value that relates to whether a user has logged into their account. If the condition is true, the ternary operator returns a personalized greeting. If the condition is false, it returns a generic greeting.
This is a neat way of writing simple logic. If we used an if/else statement instead of a ternary operation, the function would take up more space to achieve exactly the same result.
Evaluate Truthy/Falsy Conditions With Ternary Operator
Just like if/else statements in JavaScript, ternary expressions can evaluate truthy or falsy conditions. These conditions might return true or false values, but might also return other values that JavaScript coerces to be true or false. For example, if a condition returns null , NaN , 0 , an empty string ( “” ) or undefined , JavaScript will treat it as false in a ternary operation.
This behavior comes in handy when dealing with conditions that return missing values. We can use our custom greeting function from the previous example to show this. Giving this function a condition that returns null executes the “false” expression by default.
Although truthy and falsy conditions can be useful, they can also have unintended consequences. For example, we could assign a value of 1 or 0 to our condition signedIn . But a mistake in this assignment could result in signedIn having a NaN or undefined value. JavaScript would then treat the condition as false without any warning, which could lead to unexpected behavior.
To avoid situations like this, we can set conditions that test for exact values. If we only wanted to output a personalized greeting if signedIn has a value of 1 , we could write the following.
Using the Ternary Operator With Many Conditions
The ternary operator can also be an alternative to more complex if/else statements with several conditions. For example, we could check that members on our website are both signed in and have a paid membership before giving them a custom greeting. We can do this in a ternary operation by adding the extra condition using the && operator.
Again, this phrasing is more concise than an if/else statement. But we can go even further with the ternary operator by specifying multiple “else” conditions.
For instance, we could use the ternary operator to determine which stage of life a customer is in based on their age. If we wanted to classify users as children, teenagers, adults, or seniors, we could use the following function ternary operation:
Chaining together ternary operators like this saves plenty of space. Rewriting this function as a chain of if/else statements takes up around twice as many lines.
This is also an example where using if/else statements leads to less readable code. Although the ternary operator displays each condition and its corresponding return value in the same line, the if/else statement separates these pairings. This makes the logic of the if/else code harder to follow at a glance. If used within longer scripts, this might also make such code harder to debug, giving further reason to use the ternary operator.
Strengths and Limitations of the Ternary Operator
As seen above, the ternary operator in JavaScript has many strengths as an alternative to if/else statements.
Strengths of the Ternary Operator in JavaScript
- It can represent conditional statements more concisely than if/else statements
- In cases where conditions and return values are simple, ternary operator statements are easier to read than if/else statements
- As a longstanding feature of JavaScript, it has excellent cross-browser compatibility
Yet there are situations where programmers should avoid using the ternary operator.
Limitations of the Ternary Operator in JavaScript
- Conditional statements with longer expressions can be hard to read with the ternary operator’s inline syntax. These expressions could otherwise be split across many lines in an if/else statement, resulting in better readability.
- Nested conditions are also better expressed as if/else statements than with the ternary operator. Although you can nest conditional statements with the ternary operator, the result is often messy and hard to debug. If/else statements lend themselves better to nested conditions because they are split over many lines. This visual separation makes each condition easier to understand and maintain.
More in JavaScript 8 Common JavaScript Data Structures
Start Using the Ternary Operator in JavaScript
In summary, the ternary operator is a great way of phrasing conditional statements. Although it isn’t suited to dense expressions or nested conditions, it can still replace long if/else statements with shorter, more readable code. Though not to be overused, it is still essential knowledge for any accomplished JavaScript programmer.
Built In’s expert contributor network publishes thoughtful, solutions-oriented stories written by innovative tech professionals. It is the tech industry’s definitive destination for sharing compelling, first-person accounts of problem-solving on the road to innovation.
Great Companies Need Great People. That's Where We Come In.

Hiring? Flexiple helps you build your dream team of developers and designers .
JavaScript Ternary Operator – Syntax and Example Use Case

Harsh Pandey
Last updated on 16 Apr 2024
The JavaScript Ternary Operator introduces a concise way to execute conditional logic in JavaScript code. Known as the conditional operator, it serves as a compact alternative to the if-else statement, enabling developers to write cleaner, more readable code. It operates on three operands: a condition, a value if the condition is true, and a value if the condition is false, structured as condition ? valueIfTrue : valueIfFalse . This operator shines in situations requiring simple decisions and assignments based on a condition. Its syntax and usage are straightforward, making it a favorite tool for optimizing code brevity and clarity.
What is the Ternary Operator?
The Ternary Operator in JavaScript is a conditional operator that facilitates the execution of code based on a ternary (three-part) condition. It is represented by the syntax condition ? expressionIfTrue : expressionIfFalse , making it the only operator in JavaScript that takes three operands. This operator evaluates the condition: if the condition is true, it returns the value of expressionIfTrue ; if false, it returns the value of expressionIfFalse .
For example:
In this case, the condition 5 > 3 is true, so the operator returns "Yes". The Ternary Operator streamlines decision-making in code, allowing for more compact expressions than traditional if-else statements. It is ideal for simple conditional assignments and inline conditions.
Syntax Description
The syntax of the JavaScript Ternary Operator consists of three parts: a condition, an expression to execute if the condition is true, and an expression to execute if the condition is false. This is represented as condition ? expressionIfTrue : expressionIfFalse . The operator first evaluates the condition. If the condition is truthy, it executes and returns the value of expressionIfTrue . If the condition is falsy, it executes and returns the value of expressionIfFalse .
For instance:
Here, the condition age >= 18 evaluates to true, thus the ternary operator returns "Eligible to vote". This succinct syntax makes the ternary operator ideal for quick conditional checks and assignments within JavaScript code, promoting readability and reducing the need for more verbose if-else statements.
Ternary Operator Used Instead of if...else
The Ternary Operator can be used instead of if...else statements to streamline conditional logic in JavaScript. It excels in scenarios where a simple condition needs to determine one of two values or actions. The ternary operator's compact form allows for inline conditional evaluations and assignments, reducing the complexity and length of code compared to traditional if...else constructs.
For example, using if...else:
The same logic with the ternary operator:
This example demonstrates how the ternary operator condenses multiple lines of an if...else statement into a single, readable line. It's particularly useful for assignments and return statements where the goal is to choose between one of two values based on a condition.
How to Use Nested Ternary Operators
To use nested ternary operators in JavaScript, one ternary operation is placed inside another, allowing for multiple conditions to be evaluated in a concise manner. This technique can handle complex logic within a single line of code, though it's crucial to maintain readability. Each nested ternary should follow the same syntax as a standard ternary operator: condition ? expressionIfTrue : expressionIfFalse .
In this example, multiple conditions determine the final grade based on a score. The first condition checks if the score is greater than or equal to 90, returning "A" if true. If false, it moves to the next condition, and so on, until a match is found or the last option is returned. While powerful, nested ternary operators should be used sparingly to avoid complicating the code. Clarity often trumps conciseness, especially when conditions become complex.
Multiple Ternary Operators Can Make Your Code Unreadable
Using multiple ternary operators in JavaScript can make your code unreadable due to the complexity and compactness of nested conditions. While the ternary operator is valuable for its brevity and inline conditional logic, overuse, especially with nesting, can lead to code that is difficult to understand at a glance. This decreases maintainability and can introduce bugs that are hard to trace.
In this snippet, the nested ternary operators evaluate the speed and return a message. While functional, deciphering the logic requires careful examination, contrasting with the clarity offered by more verbose conditional statements like if-else. It's advisable to limit the use of nested ternary operators and opt for alternatives that prioritize readability, especially in complex scenarios.
In conclusion, the JavaScript Ternary Operator offers a succinct and efficient way to perform conditional operations within your code. It stands out for its simplicity, allowing for quick decision-making between two possible outcomes based on a single condition. The ternary operator enhances code readability and maintainability, especially in situations where traditional if...else statements would introduce unnecessary complexity. However, when using nested ternary operators, it's essential to balance conciseness with clarity to ensure that your code remains accessible to others. Embracing the ternary operator can significantly streamline your JavaScript coding practices, provided it's applied judiciously and with consideration for fellow developers.
Work with top startups & companies. Get paid on time.
Try a top quality developer for 7 days. pay only if satisfied..
// Find jobs by category
You've got the vision, we help you create the best squad. Pick from our highly skilled lineup of the best independent engineers in the world.
- Ruby on Rails
- Elasticsearch
- Google Cloud
- React Native
- Contact Details
- 2093, Philadelphia Pike, DE 19703, Claymont
- [email protected]
- Explore jobs
- We are Hiring!
- Write for us
- 2093, Philadelphia Pike DE 19703, Claymont
Copyright @ 2024 Flexiple Inc
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
Assignment (=)
The assignment ( = ) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. This allows multiple assignments to be chained in order to assign a single value to multiple variables.
A valid assignment target, including an identifier or a property accessor . It can also be a destructuring assignment pattern .
An expression specifying the value to be assigned to x .
Return value
The value of y .
Thrown in strict mode if assigning to an identifier that is not declared in the scope.
Thrown in strict mode if assigning to a property that is not modifiable .
Description
The assignment operator is completely different from the equals ( = ) sign used as syntactic separators in other locations, which include:
- Initializers of var , let , and const declarations
- Default values of destructuring
- Default parameters
- Initializers of class fields
All these places accept an assignment expression on the right-hand side of the = , so if you have multiple equals signs chained together:
This is equivalent to:
Which means y must be a pre-existing variable, and x is a newly declared const variable. y is assigned the value 5 , and x is initialized with the value of the y = 5 expression, which is also 5 . If y is not a pre-existing variable, a global variable y is implicitly created in non-strict mode , or a ReferenceError is thrown in strict mode. To declare two variables within the same declaration, use:
Simple assignment and chaining
Value of assignment expressions.
The assignment expression itself evaluates to the value of the right-hand side, so you can log the value and assign to a variable at the same time.
Unqualified identifier assignment
The global object sits at the top of the scope chain. When attempting to resolve a name to a value, the scope chain is searched. This means that properties on the global object are conveniently visible from every scope, without having to qualify the names with globalThis. or window. or global. .
Because the global object has a String property ( Object.hasOwn(globalThis, "String") ), you can use the following code:
So the global object will ultimately be searched for unqualified identifiers. You don't have to type globalThis.String ; you can just type the unqualified String . To make this feature more conceptually consistent, assignment to unqualified identifiers will assume you want to create a property with that name on the global object (with globalThis. omitted), if there is no variable of the same name declared in the scope chain.
In strict mode , assignment to an unqualified identifier in strict mode will result in a ReferenceError , to avoid the accidental creation of properties on the global object.
Note that the implication of the above is that, contrary to popular misinformation, JavaScript does not have implicit or undeclared variables. It just conflates the global object with the global scope and allows omitting the global object qualifier during property creation.
Assignment with destructuring
The left-hand side of can also be an assignment pattern. This allows assigning to multiple variables at once.
For more information, see Destructuring assignment .
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Assignment operators in the JS guide
- Destructuring assignment
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript operators.
Javascript operators are used to perform different types of mathematical and logical computations.
The Assignment Operator = assigns values
The Addition Operator + adds values
The Multiplication Operator * multiplies values
The Comparison Operator > compares values
JavaScript Assignment
The Assignment Operator ( = ) assigns a value to a variable:
Assignment Examples
Javascript addition.
The Addition Operator ( + ) adds numbers:

JavaScript Multiplication
The Multiplication Operator ( * ) multiplies numbers:
Multiplying
Types of javascript operators.
There are different types of JavaScript operators:
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- String Operators
- Logical Operators
- Bitwise Operators
- Ternary Operators
- Type Operators
JavaScript Arithmetic Operators
Arithmetic Operators are used to perform arithmetic on numbers:
Arithmetic Operators Example
Arithmetic operators are fully described in the JS Arithmetic chapter.
Advertisement
JavaScript Assignment Operators
Assignment operators assign values to JavaScript variables.
The Addition Assignment Operator ( += ) adds a value to a variable.
Assignment operators are fully described in the JS Assignment chapter.
JavaScript Comparison Operators
Comparison operators are fully described in the JS Comparisons chapter.
JavaScript String Comparison
All the comparison operators above can also be used on strings:
Note that strings are compared alphabetically:
JavaScript String Addition
The + can also be used to add (concatenate) strings:
The += assignment operator can also be used to add (concatenate) strings:
The result of text1 will be:
When used on strings, the + operator is called the concatenation operator.
Adding Strings and Numbers
Adding two numbers, will return the sum, but adding a number and a string will return a string:
The result of x , y , and z will be:
If you add a number and a string, the result will be a string!
JavaScript Logical Operators
Logical operators are fully described in the JS Comparisons chapter.
JavaScript Type Operators
Type operators are fully described in the JS Type Conversion chapter.
JavaScript Bitwise Operators
Bit operators work on 32 bits numbers.
The examples above uses 4 bits unsigned examples. But JavaScript uses 32-bit signed numbers. Because of this, in JavaScript, ~ 5 will not return 10. It will return -6. ~00000000000000000000000000000101 will return 11111111111111111111111111111010
Bitwise operators are fully described in the JS Bitwise chapter.
Test Yourself With Exercises
Multiply 10 with 5 , and alert the result.
Start the Exercise
Test Yourself with Exercises!
Exercise 1 » Exercise 2 » Exercise 3 » Exercise 4 » Exercise 5 »

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Python Operators
Precedence and associativity of operators in python.
- Python Arithmetic Operators
- Difference between / vs. // operator in Python
- Python - Star or Asterisk operator ( * )
- What does the Double Star operator mean in Python?
- Division Operators in Python
- Modulo operator (%) in Python
- Python Logical Operators
- Python OR Operator
- Difference between 'and' and '&' in Python
- not Operator in Python | Boolean Logic
Ternary Operator in Python
- Python Bitwise Operators
Python Assignment Operators
Assignment operators in python.
- Walrus Operator in Python 3.8
- Increment += and Decrement -= Assignment Operators in Python
- Merging and Updating Dictionary Operators in Python 3.9
- New '=' Operator in Python3.8 f-string
Python Relational Operators
- Comparison Operators in Python
- Python NOT EQUAL operator
- Difference between == and is operator in Python
- Chaining comparison operators in Python
- Python Membership and Identity Operators
- Difference between != and is not operator in Python
In Python programming, Operators in general are used to perform operations on values and variables. These are standard symbols used for logical and arithmetic operations. In this article, we will look into different types of Python operators.
- OPERATORS: These are the special symbols. Eg- + , * , /, etc.
- OPERAND: It is the value on which the operator is applied.
Types of Operators in Python
- Arithmetic Operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Identity Operators and Membership Operators
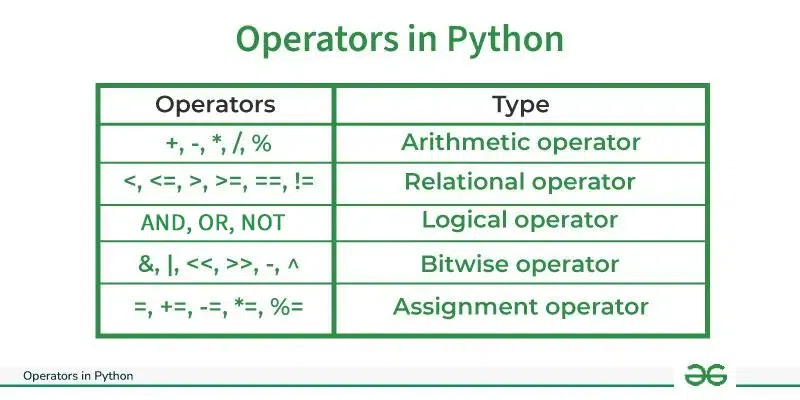
Arithmetic Operators in Python
Python Arithmetic operators are used to perform basic mathematical operations like addition, subtraction, multiplication , and division .
In Python 3.x the result of division is a floating-point while in Python 2.x division of 2 integers was an integer. To obtain an integer result in Python 3.x floored (// integer) is used.
Example of Arithmetic Operators in Python
Division operators.
In Python programming language Division Operators allow you to divide two numbers and return a quotient, i.e., the first number or number at the left is divided by the second number or number at the right and returns the quotient.
There are two types of division operators:
Float division
- Floor division
The quotient returned by this operator is always a float number, no matter if two numbers are integers. For example:
Example: The code performs division operations and prints the results. It demonstrates that both integer and floating-point divisions return accurate results. For example, ’10/2′ results in ‘5.0’ , and ‘-10/2’ results in ‘-5.0’ .
Integer division( Floor division)
The quotient returned by this operator is dependent on the argument being passed. If any of the numbers is float, it returns output in float. It is also known as Floor division because, if any number is negative, then the output will be floored. For example:
Example: The code demonstrates integer (floor) division operations using the // in Python operators . It provides results as follows: ’10//3′ equals ‘3’ , ‘-5//2’ equals ‘-3’ , ‘ 5.0//2′ equals ‘2.0’ , and ‘-5.0//2’ equals ‘-3.0’ . Integer division returns the largest integer less than or equal to the division result.
Precedence of Arithmetic Operators in Python
The precedence of Arithmetic Operators in Python is as follows:
- P – Parentheses
- E – Exponentiation
- M – Multiplication (Multiplication and division have the same precedence)
- D – Division
- A – Addition (Addition and subtraction have the same precedence)
- S – Subtraction
The modulus of Python operators helps us extract the last digit/s of a number. For example:
- x % 10 -> yields the last digit
- x % 100 -> yield last two digits
Arithmetic Operators With Addition, Subtraction, Multiplication, Modulo and Power
Here is an example showing how different Arithmetic Operators in Python work:
Example: The code performs basic arithmetic operations with the values of ‘a’ and ‘b’ . It adds (‘+’) , subtracts (‘-‘) , multiplies (‘*’) , computes the remainder (‘%’) , and raises a to the power of ‘b (**)’ . The results of these operations are printed.
Note: Refer to Differences between / and // for some interesting facts about these two Python operators.
Comparison of Python Operators
In Python Comparison of Relational operators compares the values. It either returns True or False according to the condition.
= is an assignment operator and == comparison operator.
Precedence of Comparison Operators in Python
In Python, the comparison operators have lower precedence than the arithmetic operators. All the operators within comparison operators have the same precedence order.
Example of Comparison Operators in Python
Let’s see an example of Comparison Operators in Python.
Example: The code compares the values of ‘a’ and ‘b’ using various comparison Python operators and prints the results. It checks if ‘a’ is greater than, less than, equal to, not equal to, greater than, or equal to, and less than or equal to ‘b’ .
Logical Operators in Python
Python Logical operators perform Logical AND , Logical OR , and Logical NOT operations. It is used to combine conditional statements.
Precedence of Logical Operators in Python
The precedence of Logical Operators in Python is as follows:
- Logical not
- logical and
Example of Logical Operators in Python
The following code shows how to implement Logical Operators in Python:
Example: The code performs logical operations with Boolean values. It checks if both ‘a’ and ‘b’ are true ( ‘and’ ), if at least one of them is true ( ‘or’ ), and negates the value of ‘a’ using ‘not’ . The results are printed accordingly.
Bitwise Operators in Python
Python Bitwise operators act on bits and perform bit-by-bit operations. These are used to operate on binary numbers.
Precedence of Bitwise Operators in Python
The precedence of Bitwise Operators in Python is as follows:
- Bitwise NOT
- Bitwise Shift
- Bitwise AND
- Bitwise XOR
Here is an example showing how Bitwise Operators in Python work:
Example: The code demonstrates various bitwise operations with the values of ‘a’ and ‘b’ . It performs bitwise AND (&) , OR (|) , NOT (~) , XOR (^) , right shift (>>) , and left shift (<<) operations and prints the results. These operations manipulate the binary representations of the numbers.
Python Assignment operators are used to assign values to the variables.
Let’s see an example of Assignment Operators in Python.
Example: The code starts with ‘a’ and ‘b’ both having the value 10. It then performs a series of operations: addition, subtraction, multiplication, and a left shift operation on ‘b’ . The results of each operation are printed, showing the impact of these operations on the value of ‘b’ .
Identity Operators in Python
In Python, is and is not are the identity operators both are used to check if two values are located on the same part of the memory. Two variables that are equal do not imply that they are identical.
Example Identity Operators in Python
Let’s see an example of Identity Operators in Python.
Example: The code uses identity operators to compare variables in Python. It checks if ‘a’ is not the same object as ‘b’ (which is true because they have different values) and if ‘a’ is the same object as ‘c’ (which is true because ‘c’ was assigned the value of ‘a’ ).
Membership Operators in Python
In Python, in and not in are the membership operators that are used to test whether a value or variable is in a sequence.
Examples of Membership Operators in Python
The following code shows how to implement Membership Operators in Python:
Example: The code checks for the presence of values ‘x’ and ‘y’ in the list. It prints whether or not each value is present in the list. ‘x’ is not in the list, and ‘y’ is present, as indicated by the printed messages. The code uses the ‘in’ and ‘not in’ Python operators to perform these checks.
in Python, Ternary operators also known as conditional expressions are operators that evaluate something based on a condition being true or false. It was added to Python in version 2.5.
It simply allows testing a condition in a single line replacing the multiline if-else making the code compact.
Syntax : [on_true] if [expression] else [on_false]
Examples of Ternary Operator in Python
The code assigns values to variables ‘a’ and ‘b’ (10 and 20, respectively). It then uses a conditional assignment to determine the smaller of the two values and assigns it to the variable ‘min’ . Finally, it prints the value of ‘min’ , which is 10 in this case.
In Python, Operator precedence and associativity determine the priorities of the operator.
Operator Precedence in Python
This is used in an expression with more than one operator with different precedence to determine which operation to perform first.
Let’s see an example of how Operator Precedence in Python works:
Example: The code first calculates and prints the value of the expression 10 + 20 * 30 , which is 610. Then, it checks a condition based on the values of the ‘name’ and ‘age’ variables. Since the name is “ Alex” and the condition is satisfied using the or operator, it prints “Hello! Welcome.”
Operator Associativity in Python
If an expression contains two or more operators with the same precedence then Operator Associativity is used to determine. It can either be Left to Right or from Right to Left.
The following code shows how Operator Associativity in Python works:
Example: The code showcases various mathematical operations. It calculates and prints the results of division and multiplication, addition and subtraction, subtraction within parentheses, and exponentiation. The code illustrates different mathematical calculations and their outcomes.
To try your knowledge of Python Operators, you can take out the quiz on Operators in Python .
Python Operator Exercise Questions
Below are two Exercise Questions on Python Operators. We have covered arithmetic operators and comparison operators in these exercise questions. For more exercises on Python Operators visit the page mentioned below.
Q1. Code to implement basic arithmetic operations on integers
Q2. Code to implement Comparison operations on integers
Explore more Exercises: Practice Exercise on Operators in Python
Please Login to comment...
Similar reads.
- python-basics
- Python-Operators
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Unary, Binary, and Ternary Operators in JavaScript – Explained with Examples
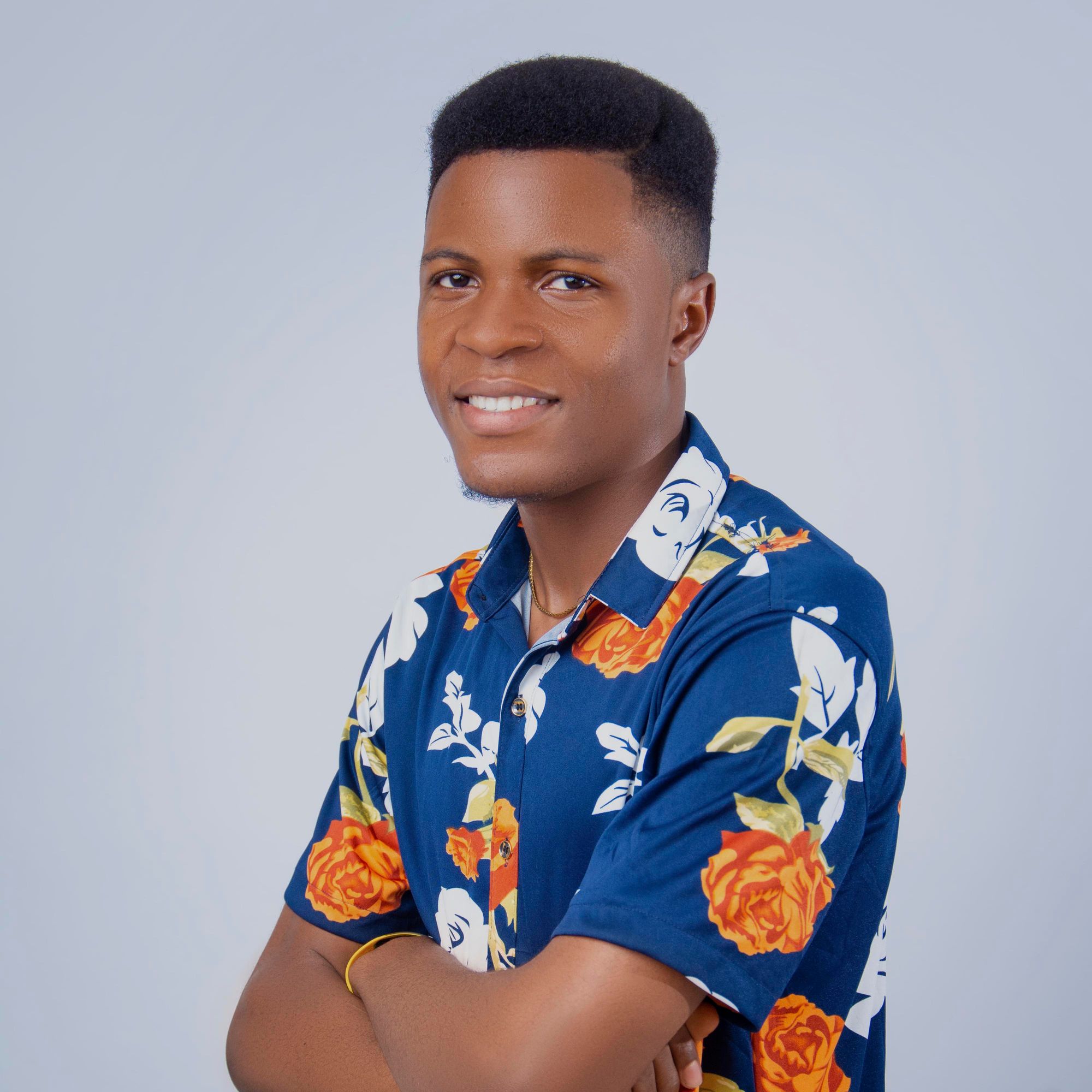
There are many operators in JavaScript that let you carry out different operations.
These operators can be categorized based on the number of operands they require, and I'll be using examples to explain these categories in this tutorial.
The three categories of operators based on the number of operands they require are:
- Unary operators: which require one operand (Un)
- Binary operators: which require two operands (Bi)
- Ternary operators: which require three operands (Ter)
Note that these categories do not only apply to JavaScript. They apply to programming in general.
In the rest of this article, I will share some examples of operators that fall under each category.
I have a video version of this topic you can watch if you're interested.
What is an Operand?
First, let's understand what an operand is. In an operation, an operand is the data that is being operated on . The operand combined with the operator makes an operation.
Look at this example:
Here we have a sum operation (which we will learn more about later). This operation involves the plus operator + , and there are two operands here: 20 and 30 .
Now that we understand operands, let's see examples of operators and the categories they fall under.
What is a Unary Operator?
These operators require one operand for operation. Providing two or more can result in a syntax error. Here are some examples of operators that fall under this category.
the typeof operator
The typeof operator returns the data type of a value. It requires only one operand. Here's an example:
If you pass two operands to it, you'd get an error:
The delete operator
You can use the delete operator to delete an item in an array or delete a property in an object. It's a unary operator that requires only one operand. Here's an example with an array:
Note that deleting items from an array with the delete operator is not the right way to do this. I explained why in this article here
And here's an example with an object:
The Unary plus + operator
This operator is not to be confused with the arithmetic plus operator which I will explain later in this article. The unary plus operator attempts to convert a non-number value to a number. It returns NaN where impossible. Here's an example:
As you can see here again, only one operand is required, which comes after the operator.
I'll stop with these three examples. But know that there are more unary operators such as the increment ++ , decrement ++ , and Logical NOT ! operators, to name a few.
What is a Binary Operator?
These operators require two operands for operation. If one or more than two operands are provided, such operators result in a syntax error.
Let's look at some operators that fall under this category
Arithmetic Operators
All arithmetic operators are binary operators. You have the first operand on the left of the operator, and the second operand on the right of the operator. Here are some examples:
If you don't provide two operands, you will get a syntax error. For example:
Comparison Operators
All comparison operators also require two operands. Here are some examples:
Assignment Operator =
The assignment operator is also a binary operator as it requires two operands. For example:
On the left, you have the first operand, the variable ( const number ), and on the right, you have the second operand, the value ( 20 ).
You're probably asking: "isn't const number two operands?". Well, const and number makes up one operand. The reason for this is const defines the behavior of number . The assignment operator = does not need const . So you can actually use the operator like this:
But it's good practice to always use a variable keyword.
So like I said, think of const number as one operand, and the value on the right as the second operand.
What is a Ternary Operator?
These operators require three operands. In JavaScript, there is one operator that falls under this category – the conditional operator. In other languages, perhaps, there could be more examples.
The Conditional Operator ? ... :
The conditional operator requires three operands:
- the conditional expression
- the truthy expression which gets evaluated if the condition is true
- the falsy expression which gets evaluated if the condition is false .
You can learn more about the Conditional Operator here
Here's an example of how it works:
The first operand – the conditional expression – is score > 50 .
The second operand – the truthy expression – is "Good", which will be returned to the variable scoreRating if the condition is true .
The third operand – the falsy expression – is "Poor", which will be returned to the variable scoreRating if the condition is false .
I've written an article related to this operator that you can check out here . It's about why a ternary operator is not a conditional operator in JavaScript.
Operations in JavaScript involve one or more operands and an operator. And operators can be categorized based on the number of operands they require.
In this article, we've looked at the three categories of operators: unary , binary , and ternary . We also looked at the examples of operators in JavaScript that fall under each category.
Please share this article if you find it helpful.
Developer Advocate and Content Creator passionate about sharing my knowledge on Tech. I simplify JavaScript / ReactJS / NodeJS / Frameworks / TypeScript / et al My YT channel: youtube.com/c/deeecode
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started

IMAGES
VIDEO
COMMENTS
The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark (?), then an expression to execute if the condition is truthy followed by a colon (:), and finally the expression to execute if the condition is falsy. This operator is frequently used as an alternative to an if ...
It works perfectly: > var t = 1 == 1 ? 1 : 0; undefined. > t. 1. You could say that the return value of the assignment operation is undefined, not the value of t. Edit: But actually if I read the specification correctly, it seems that it should return the value of the expression. As @T.J. Crowder mentioned, it seems the var is responsible for ...
Inside the function, we use the ternary operator to check if the number is even or odd. If the number modulo 2 equals 0 (meaning it's divisible by 2 with no remainder), then the condition evaluates to true, and the string "even" is assigned to the result variable. If the condition evaluates to false (meaning the number is odd), the string "odd ...
JavaScript ternary operator examples. Let's take some examples of using the ternary operator. 1) Using the JavaScript ternary operator to perform multiple statements. The following example uses the ternary operator to perform multiple operations, where each operation is separated by a comma. For example:
In this example, I used the ternary operator to determine whether a user's age is greater than or equal to 18. Firstly, I used the prompt() built-in JavaScript function. This function opens a dialog box with the message What is your age? and the user can enter a value. I store the user's input in the age variable.
A ternary operator can be used to replace an if..else statement in certain situations. Before you learn about ternary operators, be sure to check the JavaScript if...else tutorial. What is a Ternary operator? A ternary operator evaluates a condition and executes a block of code based on the condition. Its syntax is: condition ? expression1 ...
Multiple Ternary Operators Can Make Your Code Unreadable. In the previous examples, we've seen how we can improve our code while maintaining readability. But you have to be careful when using multiple ternary operators. Imagine we had an extra ternary operator in our previous example: const score = 45 const scoreRating = score > 70 ?
The ternary operator in JavaScript is a shorthand way of writing an if-else statement. It is called the ternary operator because it takes three operands: a condition, a result for true, and a ...
An assignment operator assigns a value to its left operand based on the value of its right operand. The simple assignment operator is equal (=), which assigns the value of its right operand to its left operand.That is, x = f() is an assignment expression that assigns the value of f() to x. There are also compound assignment operators that are shorthand for the operations listed in the ...
JavaScript Ternary Operator. JavaScript Ternary Operator (Conditional Operator) is a concise way to write a conditional (if-else) statement. Ternary Operator takes three operands i.e. condition, true value and false value. In this article, we are going to learn about Ternary Operator.
Writing a Simple Expression With the Ternary Operator The ternary operator gets its name by being the only operator in JavaScript that takes three operands, or parts. The first part of a ternary operation is a logical condition that returns a true or false value. Then, after a question mark, come two expressions, separated by a colon. The first ...
The Ternary Operator can be used instead of if...else statements to streamline conditional logic in JavaScript. It excels in scenarios where a simple condition needs to determine one of two values or actions. The ternary operator's compact form allows for inline conditional evaluations and assignments, reducing the complexity and length of code ...
The reader must know about and understand the meaning of the comma operator and the meaning of the assignment operator when it is used in an expression. More, they have to juggle with the possible values of exp.id == "xyz" struggling to understand when a value is assigned to selectedData and when the callback of Array.some() returns true and why.
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
The reason for this is that the addition operator (+ to the common folk) has higher "precedence" (6) than the conditional/ternary operator (15). I know the numbers appear backwards. Precedence simply means that each type of operator in a language is evaluated in a particular predefined order (and not just left-to-right).
JavaScript Assignment Operators. Assignment operators assign values to JavaScript variables. The Addition Assignment Operator (+=) adds a value to a variable. ... ternary operator: Note. Comparison operators are fully described in the JS Comparisons chapter. JavaScript String Comparison.
Conclusion. In this tutorial, you've learned the 7 types of JavaScript operators: Arithmetic, assignment, comparison, logical, ternary, typeof, and bitwise operators. These operators can be used to manipulate values and variables to achieve a desired outcome. Congratulations on finishing this guide!
The ternary operator is a conditional operator that takes three operands: a condition, a value to be returned if the condition is true, and a value to be returned if the condition is false. It evaluates the condition and returns one of the two specified values based on whether the condition is true or false. Syntax of Ternary Operator: The ...
I don't find two this.xs being redundant in that specific syntax, as there are two operators, and both of the operators need their operands. The case just happens to use the same variable in the operands of both of the operators. If the case was const x = (y) ? 1 : 2;, you wouldn't think 1 was redundant. For what you're after, we'd need a brand ...
Assignment Operators in Python. Let's see an example of Assignment Operators in Python. Example: The code starts with 'a' and 'b' both having the value 10. It then performs a series of operations: addition, subtraction, multiplication, and a left shift operation on 'b'.
Here's an example: +"200" // 20 - number +false // 0 - number representation +"hello" // NaN. As you can see here again, only one operand is required, which comes after the operator. I'll stop with these three examples. But know that there are more unary operators such as the increment ++, decrement ++, and Logical NOT ! operators, to name a few.
"I'm trying to re-write existing code into the ternary operator way." — Don't. As you have noticed, it is hard to understand. Don't make more work for whomever has to maintain your code. They'll hate you for it. That person is likely to be you but 6 months older. -