COMP337 - Chapter 2 Socket Assignments
For chapter two you’ll be doing some programming assignments in lieu of an exam. Each of the assignments involves the use of the python socket module . Spending some time with that documentation would be wise. I suggest you begin by finding documentation on the methods used in the book and work from there as needed.

Lab 1 - Web Server
DUE FRIDAY 2/14
The first set of programs consists of a bare bones server along with two simple clients. The first uses basic sockets where the second uses a modern HTTP client library. Your goal is to get your feet wet sending and receiving messages using HTTP. First you write a server and test it with the client, a browser. Then write your own client using a modern library. Finally, write a client using sockets to get a taste for what goes on behind the scenes of the library. Be sure to checkout the TCP client template discussed in section 2.8 and use that as a starter for your client.
- Complete the web server as described in the handout.
- Do exercise 2 but use the requests library rather than low level sockets.
- Do exercise 2 but drop down to low level sockets. No request module.
Lab 2 - UDP Pinger
DUE MONDAY 2/17
The second set of programs is about building your own protocol for a well known application: ping. We’ll tackle the real ping protocol later, but for now you’ll try to get at the same information via a this roll-your-own protocol. The lack of an established protocol means you cannot find or use some preexisting library and must stay within sockets. Be sure to checkout the UDP client template discussed in section 2.7 and use that as a starter for your client.
- Complete the lab as written
- Do both optional exercises
You’ll want to run your servers for this lab on the departmental server.
DUE WEDNESDAY 2/19
Now that you’ve cut your teeth on some client-server applications lets build a RESTful API . These kinds of APIs are at the heart of a lot of the modern web. They let developers layer on top of other web-applications in much the same way that they do with libraries and modules for native applications. You’re API is a straight forward dice rolling API .
Programming Assignment: UDP Ping Lab
You are given the complete code for the Ping server in Java below. Your job is to write the Ping client in C .
Server Code
import java.io.*; import java.net.*; import java.util.*; /* * Server to process ping requests over UDP. */ public class PingServer { private static final double LOSS_RATE = 0.3; private static final int AVERAGE_DELAY = 100; // milliseconds public static void main(String[] args) throws Exception { // Get command line argument. if (args.length != 1) { System.out.println("Required arguments: port"); return; } int port = Integer.parseInt(args[0]); // Create random number generator for use in simulating // packet loss and network delay. Random random = new Random(); // Create a datagram socket for receiving and sending UDP packets // through the port specified on the command line. DatagramSocket socket = new DatagramSocket(port); // Processing loop. while (true) { // Create a datagram packet to hold incomming UDP packet. DatagramPacket request = new DatagramPacket(new byte[1024], 1024); // Block until the host receives a UDP packet. socket.receive(request); // Print the recieved data. printData(request); // Decide whether to reply, or simulate packet loss. if (random.nextDouble() < LOSS_RATE) { System.out.println(" Reply not sent."); continue; } // Simulate network delay. Thread.sleep((int) (random.nextDouble() * 2 * AVERAGE_DELAY)); // Send reply. InetAddress clientHost = request.getAddress(); int clientPort = request.getPort(); byte[] buf = request.getData(); DatagramPacket reply = new DatagramPacket(buf, buf.length, clientHost, clientPort); socket.send(reply); System.out.println(" Reply sent."); } } /* * Print ping data to the standard output stream. */ private static void printData(DatagramPacket request) throws Exception { // Obtain references to the packet's array of bytes. byte[] buf = request.getData(); // Wrap the bytes in a byte array input stream, // so that you can read the data as a stream of bytes. ByteArrayInputStream bais = new ByteArrayInputStream(buf); // Wrap the byte array output stream in an input stream reader, // so you can read the data as a stream of characters. InputStreamReader isr = new InputStreamReader(bais); // Wrap the input stream reader in a buffered reader, // so you can read the character data a line at a time. // (A line is a sequence of chars terminated by any combination of \r and \n.) BufferedReader br = new BufferedReader(isr); // The message data is contained in a single line, so read this line. String line = br.readLine(); // Print host address and data received from it. System.out.println( "Received from " + request.getAddress().getHostAddress() + ": " + new String(line) ); } }
Packet Loss
UDP provides applications with an unreliable transport service, because messages may get lost in the network due to router queue overflows or other reasons. In contrast, TCP provides applications with a reliable transport service and takes care of any lost packets by retransmitting them until they are successfully received. Applications using UDP for communication must therefore implement any reliability they need separately in the application level (each application can implement a different policy, according to its specific needs). Because packet loss is rare or even non-existent in typical campus networks, the server in this lab injects artificial loss to simulate the effects of network packet loss. The server has a parameter LOSS_RATE that determines which percentage of packets should be lost. The server also has another parameter AVERAGE_DELAY that is used to simulate transmission delay from sending a packet across the Internet. You should set AVERAGE_DELAY to a positive value when testing your client and server on the same machine, or when machines are close by on the network. You can set AVERAGE_DELAY to 0 to find out the true round trip times of your packets.
Compiling and Running Server To compile the server, do the following:
You should write the client so that it sends 10 ping requests to the server, separated by approximately one second. Each message contains a payload of data that includes the keyword PING, a sequence number, and a timestamp. After sending each packet, the client waits up to one second to receive a reply. If one seconds goes by without a reply from the server, then the client assumes that its packet or the server's reply packet has been lost in the network.
You should write the client so that it starts with the following command:
Message Format
The ping messages in this lab are formatted in a simple way. Each message contains a sequence of characters terminated by a carriage return character ( r ) and a line feed character ( n ). The message contains the following string:
Client Output

Programming Assignments
As teachers, we've found that programming assignments are often a great way for students to cement their understanding of the principles and practice of networking. Students can "get their hands dirty," "learn by doing" and derive great satisfaction (and sometimes relief!) by building something that works.
This page contains programming assignment descriptions, and supplementary code fragments (when needed) do the programming assignments in our book. Most assignments are available in multiple languages, we recommend Python (which is the mostly used/taught) and C (which lets a student program closest to the underlying socket abstraction). Solutions to these assignments are available to instructors (only) here . If you're a faculty member who would like to access the solutions, contact one of the authors at [email protected] .
- A simple client/server simple socket program ( Python,Java,C )
- Webserver ( Python )
- UDP Pinger Lab ( Python , Java )
- SMTP Lab ( Python,Java )
- HTTP Web Proxy Server Lab ( Python , Java )
- ICMP Pinger Lab ( Python )
- Traceroute Lab ( Python )
- Video Streaming with RTSP and RTP Lab ( Python , Java )
- Reliable data transfer protocol (RDT) Lab ( Python,C )
- Distance Vector Algorithm ( C,Java )
We gratefully acknowledge the programming and problem design work of John Broderick (UMass '21), which has really helped to substantially improve this site.
Copyright © 2010-2024 J.F. Kurose, K.W. Ross Comments welcome and appreciated: [email protected]
All About Programming Languages
- 0.00 $ 0 items

SOLVED:Programming Assignment 3: UDP Pinger Lab
30.00 $
You'll get a download link with a: . zip solution files instantly, after Payment
If Helpful Share:

Description
In this lab, you will study a simple Internet ping server written in the Java language, and implement a corresponding client. The functionality provided by these programs are similar to the standard ping programs available in modern operating systems, except that they use UDP rather than Internet Control Message Protocol (ICMP) to communicate with each other. (Java does not provide a straightforward means to interact with ICMP.) The ping protocol allows a client machine to send a packet of data to a remote machine, and have the remote machine return the data back to the client unchanged (an action referred to as echoing). Among other uses, the ping protocol allows hosts to determine round-trip times to other machines. You are given the complete code for the Ping server below. Your job is to write the Ping client. Server Code The following code fully implements a ping server. You need to compile and run this code. You should study this code carefully, as it will help you write your Ping client. import java.io.*; import java.net.*; import java.util.*; /* * Server to process ping requests over UDP. */ public class PingServer { private static final double LOSS_RATE = 0.3; private static final int AVERAGE_DELAY = 100; // milliseconds public static void main(String[] args) throws Exception { // Get command line argument. if (args.length != 1) { System.out.println(“Required arguments: port”); return; } int port = Integer.parseInt(args[0]); // Create random number generator for use in simulating // packet loss and network delay. Random random = new Random(); // Create a datagram socket for receiving and sending UDP packets // through the port specified on the command line. DatagramSocket socket = new DatagramSocket(port); // Processing loop. while (true) { // Create a datagram packet to hold incomming UDP packet. DatagramPacket request = new DatagramPacket(new byte[1024], 1024); // Block until the host receives a UDP packet. socket.receive(request);
// Print the recieved data. printData(request); // Decide whether to reply, or simulate packet loss. if (random.nextDouble() < LOSS_RATE) { System.out.println(” Reply not sent.”); continue; } // Simulate network delay. Thread.sleep((int) (random.nextDouble() * 2 * AVERAGE_DELAY)); // Send reply. InetAddress clientHost = request.getAddress(); int clientPort = request.getPort(); byte[] buf = request.getData(); DatagramPacket reply = new DatagramPacket(buf, buf.length, clientHost, clientPort); socket.send(reply); System.out.println(” Reply sent.”); } } /* * Print ping data to the standard output stream. */ private static void printData(DatagramPacket request) throws Exception { // Obtain references to the packet’s array of bytes. byte[] buf = request.getData(); // Wrap the bytes in a byte array input stream, // so that you can read the data as a stream of bytes. ByteArrayInputStream bais = new ByteArrayInputStream(buf); // Wrap the byte array output stream in an input stream reader, // so you can read the data as a stream of characters. InputStreamReader isr = new InputStreamReader(bais); // Wrap the input stream reader in a bufferred reader, // so you can read the character data a line at a time. // (A line is a sequence of chars terminated by any combination of \r and \n.) BufferedReader br = new BufferedReader(isr); // The message data is contained in a single line, so read this line. String line = br.readLine(); // Print host address and data received from it. System.out.println( “Received from ” + request.getAddress().getHostAddress() + “: ” + new String(line) ); } } The server sits in an infinite loop listening for incoming UDP packets. When a packet comes in, the server simply sends the encapsulated data back to the client. Packet Loss UDP provides applications with an unreliable transport service, because messages may get lost in the network due to router queue overflows or other reasons. In contrast, TCP provides applications with a reliable transport service and takes care of any lost packets by retransmitting them until they are successfully received. Applications using UDP for communication must therefore implement any reliability they need separately in the application level (each application can implement a different policy, according to its specific needs). Because packet loss is rare or even non-existent in typical campus networks, the server in this lab injects artificial loss to simulate the effects of network packet loss. The server has a parameter LOSS_RATE that determines which percentage of packets should be lost. The server also has another parameter AVERAGE_DELAY that is used to simulate transmission delay from sending a packet across the Internet. You should set AVERAGE_DELAY to a positive value when testing your client and server on the same machine, or when machines are close by on the network. You can set AVERAGE_DELAY to 0 to find out the true round trip times of your packets. Compiling and Running Server To compile the server, do the following: javac PingServer.java To run the server, do the following: java PingServer port where port is the port number the server listens on. Remember that you have to pick a port number greater than 1024, because only processes running with root (administrator) privilege can bind to ports less than 1024. Note: if you get a class not found error when running the above command, then you may need to tell Java to look in the current directory in order to resolve class references. In this case, the commands will be as follows: java -classpath . PingServer port Your Job: The Client You should write the client so that it sends 10 ping requests to the server, separated by approximately one second. Each message contains a payload of data that includes the keyword PING, a sequence number, and a timestamp. After sending each packet, the client waits up to one second to receive a reply. If one seconds goes by without a reply from the server, then the client assumes that its packet or the server’s reply packet has been lost in the network. Hint: Cut and paste PingServer, rename the code PingClient, and then modify the code. You should write the client so that it starts with the following command: java PingClient host port where host is the name of the computer the server is running on and port is the port number it is listening to. Note that you can run the client and server either on different machines or on the same machine. The client should send 10 pings to the server. Because UDP is an unreliable protocol, some of the packets sent to the server may be lost, or some of the packets sent from server to client may be lost. For this reason, the client can not wait indefinitely for a reply to a ping message. You should have the client wait up to one second for a reply; if no reply is received, then the client should assume that the packet was lost during transmission across the network. You will need to research the API for DatagramSocket to find out how to set the timeout value on a datagram socket. When developing your code, you should run the ping server on your machine, and test your client by sending packets to localhost (or, 127.0.0.1). After you have fully debugged your code, you should see how your application communicates across the network with a ping server run by another member of the class. Message Format The ping messages in this lab are formatted in a simple way. Each message contains a sequence of characters terminated by a carriage return character (r) and a line feed character (n). The message contains the following string: PING sequence_number time CRLF where sequence_number starts at 0 and progresses to 9 for each successive ping message sent by the client, time is the time when the client sent the message, and CRLF represent the carriage return and line feed characters that terminate the line. Optional Exercises When you are finished writing the client, you may wish to try one of the following exercises. 1) Currently the program calculates the round-trip time for each packet and prints them out individually. Modify this to correspond to the way the standard ping program works. You will need to report the minimum, maximum, and average RTTs. (easy) 2) The basic program sends a new ping immediately when it receives a reply. Modify the program so that it sends exactly 1 ping per second, similar to how the standard ping program works. Hint: Use the Timer and TimerTask classes in java.util. (difficult) 3) Develop two new classes ReliableUdpSender and ReliableUdpReceiver, which are used to send and receive data reliably over UDP. To do this, you will need to devise a protocol (such as TCP) in which the recipient of data sends acknowledgements back to the sender to indicate that the data has arrived. You can simplify the problem by only providing one-way transport of application data from sender to recipient. Because your experiments may be in a network environment with little or no loss of IP packets, you should simulate packet loss. (difficult)
- Assignment_3-1.zip
Related products

Solution to Program #6

Programming Problem Chapter 10-3 Pg. 494 Solution

Raptor: Sales Report Brewster’s Used Cars, Inc

[email protected]
Whatsapp +1 -419-877-7882
[email protected] /Whatsapp +1 -419-877-7882
- REFUND POLICY
- PRODUCT CATEGORIES
- GET HOMEWORK HELP
- C++ Programming Assignment
- Java Assignment Help
- MATLAB Assignment Help
- MySQL Assignment Help

Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Team Programming Assignment 2
InnovaTree/CST311_PA2_UDP_Pinger
Folders and files, repository files navigation, programming assignment 2.
CST 311-30, Introduction to Computer Networks, Online
READ INSTRUCTIONS CAREFULLY BEFORE YOU START THE ASSIGNMENT.
Assignment must be submitted electronically to Canvas by 11:59 p.m. on the due date. Late assignments will not be accepted.
This assignment requires you to work with teams assigned to you on the Team Assignment Document in Week 1. You must also adhere to the instructions on the Programming Process document.
Follow the steps below to develop and write the client part of a client server application. The naming convention of the file should be PA2_your_last_names_in_alphabetic_order.py. Put your names in the program as well. Your client program must work with the server program given to you below. Your program must have sufficient comments to clearly explain how your code works.
This assignment is worth 150 points. The grading objectives for the assignment are given below.
In this assignment, you will learn the basics of socket programming for UDP in Python. You will learn how to send and receive datagram packets using UDP sockets and also, how to set a proper socket timeout. Throughout the assignment, you will gain familiarity with a Ping application and its usefulness in computing statistics such as packet loss rate.
You will first study a simple Internet ping server written in Python, and implement a corresponding client. The functionality provided by these programs is similar to the functionality provided by standard ping programs available in modern operating systems. However, these programs use a simpler protocol, UDP, rather than the standard Internet Control Message Protocol (ICMP) to communicate with each other. The ping protocol allows a client machine to send a packet of data to a remote machine, and have the remote machine return the data back to the client unchanged (an action referred to as echoing). Among other uses, the ping protocol allows hosts to determine round-trip times to other machines.
You are given the complete code for the Ping server below. Your task is to write the Ping client.
Server Starter Code
The following code fully implements a ping server. You can use the server code given below as starter code for the assignment. You need to compile and run this code before running your client program.
In this server code, 30% of the client's packets are simulated to be lost. You should study this code carefully, as it will help you write your ping client.
The server sits in an infinite loop listening for incoming UDP packets. When the first packet, or a succeeding packet when a randomized integer is greater than or equal to 4, comes in, the server simply capitalizes the encapsulated data and sends it back to the client.
Packet Loss
UDP provides applications with an unreliable transport service. Messages may get lost in the network due to router queue overflows, faulty hardware or some other reasons. Because packet loss is rare or even non-existent in typical campus networks, the server in this assignment injects artificial loss to simulate the effects of network packet loss. The server creates a variable randomized integer which determines whether a particular incoming packet is lost or not.
Client Code
The client should send 10 pings to the server. See the Message Format below for each ping to be sent to the Server. Also print the message that is sent to the server.
Because UDP is an unreliable protocol, a packet sent from the client to the server may be lost in the network, or vice versa. For this reason, the client cannot wait indefinitely for a reply to a ping message. You should get the client to wait up to one second for a reply; if no reply is received within one second, your client program should assume that the packet was lost during transmission across the network. You will need to look up the Python documentation to find out how to set the timeout value on a datagram socket. If the packet is lost, print "Request timed out".
The program must calculate the round-trip time for each packet and print it out individually. Have the client print out the information similar to the output from doing a normal ping command – see sample output below. Your client software will need to determine and print out the minimum, maximum, and average RTTs at the end of all pings from the client along with printing out the number of packets lost and the packet loss rate (in percentage). Then compute and print the estimated RTT, the DevRTT and the Timeout interval based on the RTT results.
The initial estimated RTT is just the first Sample RTT – the results of your first ping. The initial DevRTT is the first Sample TTT divided by 2. The initial Timeout interval is set to 1 minute. The formulas for calculating the estimated RTT, DevRTT, and Timeout interval for each succeeding ping are contained in the slides on the last page of this document and on pages 242-243 of the textbook.1
Execution and Testing
You are to run and test your program in a two host, one switch mininet emulation, using delay parameters that match the real world. The client program should be run on one host (h1) and the server program should be run on the second host (h2). To set up the test environment, start mininet with the basic topology, and set speed of links to 100 Mbps and delay to 25 ms per link. (By setting delay to 25 ms, the RTT will be about 100 ms – this is the approximate RTT between San Francisco and Boston.) Here is the command to set up the test environment:
mininet@mininet-vm:~$ sudo mn --link tc,bw=100,delay=25ms
Sample output of ping to Google.com
Here is a sample output from a ping of Google 4 times:
Expected output of your program
Server Output:

Client Output :

(Values in sample below are smaller than your expected output)
Grading Objectives
- (30 points) You must complete this program in the Mininet VM. The screenshots for the running code and the results in the Output checker file must come from executing your code on the mininet VM.
- (5 points) Ping messages must be sent using UDP. Server must change the received message to uppercase before sending the message back to the client.
- (5 + 5 points) Print the request from client and response from server messages on both the client and server machines.
- Round trip time (RTT) - If the server doesn't respond, print "Request timed out".
- Minimum RTT
- Maximum RTT
- Average RTT (Leave out lost packets from average calculation)
- Estimated RTT. Consider alpha = 0.125. (Look at slides at the end for formulae.)
- Deviation RTT. Consider beta = 0.25. (Look at slides at the end for formulae.)
- Timeout Interval (Look at slides at the end for formulae.)
- Packet loss percentage
- (5 points) You must write the client code to do the assignment with the calculations in 4 above without the use of a list (or array). Find an efficient and effective use of storage and program speed.
- (5 points) Programs must be well documented.
- (5 points) Submission files are in order. (Look at the "What to hand in" section.)
- (50 points) Teamwork grade: (50 points) Each team member will grade each other teammate out of 10 points during peer evaluation. I will average all team members' grades and scale it to get your teamwork grade out of 50 points. Note that 30% of your grade will come from your teamwork and team member evaluations.
What to Hand in
- You will hand in the complete client and server code to iLearn. (Server starter is provided. You must modify the server code to print messages as shown in the Expected output section.)
- Minutes of the 3 meetings.
- Screenshots of server and client-side output. Please provide screenshots in one pdf file.
- Fill in columns B and C with RTTs and lost packets as indicated in the file - Output Checker. Your outputs in your screenshots must match the outputs calculated in the Output Checker.
- One submission per Team.
- The estimated RTT, DevRTT, and Timeout intervals are actually only used with TCP. These calculations are included in this programming assignment to give you experience working with these parameters.
- RTT Slides :

Contributors 2
- Python 100.0%
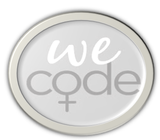
Programming Assignment 3: UDP Pinger Lab solution
In this lab, you will study a simple Internet ping server written in the Java language, and implement a corresponding client. The functionality provided by these programs are similar to the standard ping programs available in modern operating systems, except that they use UDP rather than Internet Control Message Protocol (ICMP) to communicate with each other. (Java does not provide a straightforward means to interact with ICMP.) The ping protocol allows a client machine to send a packet of data to a remote machine, and have the remote machine return the data back to the client unchanged (an action referred to as echoing). Among other uses, the ping protocol allows hosts to determine round-trip times to other machines. You are given the complete code for the Ping server below. Your job is to write the Ping client. Server Code The following code fully implements a ping server. You need to compile and run this code. You should study this code carefully, as it will help you write your Ping client. import java.io.*; import java.net.*; import java.util.*; /* * Server to process ping requests over UDP. */ public class PingServer { private static final double LOSS_RATE = 0.3; private static final int AVERAGE_DELAY = 100; // milliseconds public static void main(String[] args) throws Exception { // Get command line argument. if (args.length != 1) { System.out.println("Required arguments: port"); return; } int port = Integer.parseInt(args[0]); // Create random number generator for use in simulating // packet loss and network delay. Random random = new Random(); // Create a datagram socket for receiving and sending UDP packets // through the port specified on the command line. DatagramSocket socket = new DatagramSocket(port); // Processing loop. while (true) { // Create a datagram packet to hold incomming UDP packet. DatagramPacket request = new DatagramPacket(new byte[1024], 1024); // Block until the host receives a UDP packet. socket.receive(request); // Print the recieved data. printData(request); // Decide whether to reply, or simulate packet loss. if (random.nextDouble() < LOSS_RATE) { System.out.println(" Reply not sent."); continue; } // Simulate network delay. Thread.sleep((int) (random.nextDouble() * 2 * AVERAGE_DELAY)); // Send reply. InetAddress clientHost = request.getAddress(); int clientPort = request.getPort(); byte[] buf = request.getData(); DatagramPacket reply = new DatagramPacket(buf, buf.length, clientHost, clientPort); socket.send(reply); System.out.println(" Reply sent."); } } /* * Print ping data to the standard output stream. */ private static void printData(DatagramPacket request) throws Exception { // Obtain references to the packet's array of bytes. byte[] buf = request.getData(); // Wrap the bytes in a byte array input stream, // so that you can read the data as a stream of bytes. ByteArrayInputStream bais = new ByteArrayInputStream(buf); // Wrap the byte array output stream in an input stream reader, // so you can read the data as a stream of characters. InputStreamReader isr = new InputStreamReader(bais); // Wrap the input stream reader in a bufferred reader, // so you can read the character data a line at a time. // (A line is a sequence of chars terminated by any combination of \r and \n.) BufferedReader br = new BufferedReader(isr); // The message data is contained in a single line, so read this line. String line = br.readLine(); // Print host address and data received from it. System.out.println( "Received from " + request.getAddress().getHostAddress() + ": " + new String(line) ); } } The server sits in an infinite loop listening for incoming UDP packets. When a packet comes in, the server simply sends the encapsulated data back to the client. Packet Loss UDP provides applications with an unreliable transport service, because messages may get lost in the network due to router queue overflows or other reasons. In contrast, TCP provides applications with a reliable transport service and takes care of any lost packets by retransmitting them until they are successfully received. Applications using UDP for communication must therefore implement any reliability they need separately in the application level (each application can implement a different policy, according to its specific needs). Because packet loss is rare or even non-existent in typical campus networks, the server in this lab injects artificial loss to simulate the effects of network packet loss. The server has a parameter LOSS_RATE that determines which percentage of packets should be lost. The server also has another parameter AVERAGE_DELAY that is used to simulate transmission delay from sending a packet across the Internet. You should set AVERAGE_DELAY to a positive value when testing your client and server on the same machine, or when machines are close by on the network. You can set AVERAGE_DELAY to 0 to find out the true round trip times of your packets. Compiling and Running Server To compile the server, do the following: javac PingServer.java To run the server, do the following: java PingServer port where port is the port number the server listens on. Remember that you have to pick a port number greater than 1024, because only processes running with root (administrator) privilege can bind to ports less than 1024. Note: if you get a class not found error when running the above command, then you may need to tell Java to look in the current directory in order to resolve class references. In this case, the commands will be as follows: java -classpath . PingServer port Your Job: The Client You should write the client so that it sends 10 ping requests to the server, separated by approximately one second. Each message contains a payload of data that includes the keyword PING, a sequence number, and a timestamp. After sending each packet, the client waits up to one second to receive a reply. If one seconds goes by without a reply from the server, then the client assumes that its packet or the server's reply packet has been lost in the network. Hint: Cut and paste PingServer, rename the code PingClient, and then modify the code. You should write the client so that it starts with the following command: java PingClient host port where host is the name of the computer the server is running on and port is the port number it is listening to. Note that you can run the client and server either on different machines or on the same machine. The client should send 10 pings to the server. Because UDP is an unreliable protocol, some of the packets sent to the server may be lost, or some of the packets sent from server to client may be lost. For this reason, the client can not wait indefinitely for a reply to a ping message. You should have the client wait up to one second for a reply; if no reply is received, then the client should assume that the packet was lost during transmission across the network. You will need to research the API for DatagramSocket to find out how to set the timeout value on a datagram socket. When developing your code, you should run the ping server on your machine, and test your client by sending packets to localhost (or, 127.0.0.1). After you have fully debugged your code, you should see how your application communicates across the network with a ping server run by another member of the class. Message Format The ping messages in this lab are formatted in a simple way. Each message contains a sequence of characters terminated by a carriage return character (r) and a line feed character (n). The message contains the following string: PING sequence_number time CRLF where sequence_number starts at 0 and progresses to 9 for each successive ping message sent by the client, time is the time when the client sent the message, and CRLF represent the carriage return and line feed characters that terminate the line. Optional Exercises When you are finished writing the client, you may wish to try one of the following exercises. 1) Currently the program calculates the round-trip time for each packet and prints them out individually. Modify this to correspond to the way the standard ping program works. You will need to report the minimum, maximum, and average RTTs. (easy) 2) The basic program sends a new ping immediately when it receives a reply. Modify the program so that it sends exactly 1 ping per second, similar to how the standard ping program works. Hint: Use the Timer and TimerTask classes in java.util. (difficult) 3) Develop two new classes ReliableUdpSender and ReliableUdpReceiver, which are used to send and receive data reliably over UDP. To do this, you will need to devise a protocol (such as TCP) in which the recipient of data sends acknowledgements back to the sender to indicate that the data has arrived. You can simplify the problem by only providing one-way transport of application data from sender to recipient. Because your experiments may be in a network environment with little or no loss of IP packets, you should simulate packet loss. (difficult)
Starting from: $20
More products

IMAGES
VIDEO
COMMENTS
Programming Assignment 3: UDP Pinger Lab In this lab, you will study a simple Internet ping server written in the Java language, and implement a corresponding client. The functionality provided by these programs are similar to the standard ping programs available in modern operating systems, except that they use UDP rather than Internet Control ...
In this lab, you will learn the basics of socket programming for UDP in Python. You will learn how to send and receive datagram packets using UDP sockets and also, how to set a proper socket timeout. Throughout the lab, you will gain familiarity with a Ping application and its usefulness in computing statistics such as packet loss rate.
Programming Assignment 3: UDP Pinger Lab In this lab, you will study a simple Internet ping server written in the Java language, and implement a corresponding client. The functionality provided by these programs are similar to the standard ping programs available in modern operating systems, except that they use UDP rather than Internet Control.
Ping Lab. Programming Assignment 3: UDP Pinger Lab. In this lab, you will study a simple Internet ping server written in the Java language, and implement a corresponding client. The functionality provided by these programs are similar to the standard ping programs available in modern operating systems, except that they use UDP rather than ...
Lab 2: UDP Pinger Lab. In this lab, you will learn the basics of socket programming for UDP in Python. You will learn how to send and receive datagram packets using UDP sockets and also, how to set a proper socket timeout. Throughout the lab, you will gain familiarity with a Ping application and its usefulness in computing statistics such as ...
COMP337 - Chapter 2 Socket Assignments. For chapter two you'll be doing some programming assignments in lieu of an exam. ... Lab 2 - UDP Pinger. DUE MONDAY 2/17. The second set of programs is about building your own protocol for a well known application: ping. We'll tackle the real ping protocol later, but for now you'll try to get at the ...
Question: Programming Assignment 3: UDP Pinger Lab In this lab, you will study a simple Internet ping server written in the Java language, and implement a corresponding client. The functionality provided by these programs are similar to the standard ping programs available in modern operating systems, except that they use UDP rather than ...
Programming Assignment 3: UDP Pinger Lab In this lab, you will study a simple Internet ping server written in the Java language, and implement a corresponding client. The functionality provided by these programs are similar to the standard ping programs available in modern operating systems, except that they use UDP rather than Internet Control Message Protocol (ICMP) to communicate with each ...
During development, you should run the UDPPingerServer.py on your machine, and test your client by sending packets to localhost (or, 127.0.0.1). After you have fully debugged your code, you should see how your application communicates across the network with the ping server and ping client running on different machines.
I have been trying to code a UDP client/server in python (as seen at bottom). The code works and runs. But, I believe there is something wrong with the line: try: data, server = ... UDP Pinger Assignment. Ask Question Asked 5 years, 10 months ago. Modified 5 years, ... Raw Socket Programming UDP Python. 71 Python send UDP packet. 2 ...
Programming Assignment 3: UDP Pinger Lab In this lab, you will study a simple Internet ping server written in the Java language, and implement a corresponding client. The functionality provided by these programs are similar to the standard ping programs available in modern operating systems, except that they use UDP rather than Internet Control Message Protocol (ICMP) to communicate with each ...
Here we will create a nonstandard (but simple!) UDP-based ping program. Your ping program is to send 10 ping messages to the target server over UDP. For each message, your client is to determine and print the RTT when the corresponding pong message is returned. Because UDP is an unreliable protocol, a packet sent by the client or server may be ...
Programming Assignment: UDP Ping Lab In this lab, you will study a simple Internet ping server written in the Java language, and implement a corresponding client in C.The functionality provided by these programs are similar to the standard ping programs available in modern operating systems, except that they use UDP rather than Internet Control Message Protocol (ICMP) to communicate with each ...
Solutions to these assignments are available to instructors (only) here. If you're a faculty member who would like to access the solutions, contact one of the authors at [email protected]. A simple client/server simple socket program (Python,Java,C) Webserver ; UDP Pinger Lab (Python,Java) SMTP Lab (Python,Java)
Question: Programming Assignment 3: UDP Pinger Lab In this lab, you will study a simple Internet ping server written in the Java language and implement a corresponding client. The functionality provided by these programs are similar to the standard ping programs available in modern operating systems, cncept that they use UDP rather than ...
In this lab, you will study a simple Internet ping server written in the Java language, and implement a corresponding client. The functionality provided by these programs are similar to the standard ping programs available in modern operating systems, except that they use UDP rather than Internet Control Message Protocol (ICMP) to communicate with each other.
This assignment requires you to work with teams assigned to you on the Team Assignment Document in Week 1. You must also adhere to the instructions on the Programming Process document. Follow the steps below to develop and write the client part of a client server application. The naming convention of the file should be PA2_your_last_names_in ...
Programming Assignment 3: UDP Pinger Lab In this lab, you will study a simple Internet ping server written in the Java language, and implement a corresponding client. The functionality provided by these programs are similar to the standard ping programs available in modern operating systems, except that they use UDP rather than Internet Control Message Protocol (ICMP) to communicate with each ...
Programming Assignment 3: UDP Pinger Lab. In this lab, you will study a simple Internet ping server written in the Java language, and. implement a corresponding client. The functionality provided by these programs are similar to. the standard ping programs available in modern operating systems, except that they use UDP
Programming Assignment 3: UDP Pinger Lab solution. In this lab, you will study a simple Internet ping server written in the Java language, and implement a corresponding client. The functionality provided by these programs are similar to the standard ping programs available in modern operating systems, except that they use UDP rather than ...