
Home > Bash Scripting Tutorial > Bash Variables > Variable Declaration and Assignment > How to Assign Variable in Bash Script? [8 Practical Cases]

How to Assign Variable in Bash Script? [8 Practical Cases]

Variables allow you to store and manipulate data within your script, making it easier to organize and access information. In Bash scripts , variable assignment follows a straightforward syntax, but it offers a range of options and features that can enhance the flexibility and functionality of your scripts. In this article, I will discuss modes to assign variable in the Bash script . As the Bash script offers a range of methods for assigning variables, I will thoroughly delve into each one.
Key Takeaways
- Getting Familiar With Different Types Of Variables.
- Learning how to assign single or multiple bash variables.
- Understanding the arithmetic operation in Bash Scripting.
Free Downloads
Local vs global variable assignment.
In programming, variables are used to store and manipulate data. There are two main types of variable assignments: local and global .
A. Local Variable Assignment
In programming, a local variable assignment refers to the process of declaring and assigning a variable within a specific scope, such as a function or a block of code. Local variables are temporary and have limited visibility, meaning they can only be accessed within the scope in which they are defined.
Here are some key characteristics of local variable assignment:
- Local variables in bash are created within a function or a block of code.
- By default, variables declared within a function are local to that function.
- They are not accessible outside the function or block in which they are defined.
- Local variables typically store temporary or intermediate values within a specific context.
Here is an example in Bash script.
In this example, the variable x is a local variable within the scope of the my_function function. It can be accessed and used within the function, but accessing it outside the function will result in an error because the variable is not defined in the outer scope.
B. Global Variable Assignment
In Bash scripting, global variables are accessible throughout the entire script, regardless of the scope in which they are declared. Global variables can be accessed and modified from any script part, including within functions.
Here are some key characteristics of global variable assignment:
- Global variables in bash are declared outside of any function or block.
- They are accessible throughout the entire script.
- Any variable declared outside of a function or block is considered global by default.
- Global variables can be accessed and modified from any script part, including within functions.
Here is an example in Bash script given in the context of a global variable .
It’s important to note that in bash, variable assignment without the local keyword within a function will create a global variable even if there is a global variable with the same name. To ensure local scope within a function , using the local keyword explicitly is recommended.
Additionally, it’s worth mentioning that subprocesses spawned by a bash script, such as commands executed with $(…) or backticks , create their own separate environments, and variables assigned within those subprocesses are not accessible in the parent script .
8 Different Cases to Assign Variables in Bash Script
In Bash scripting , there are various cases or scenarios in which you may need to assign variables. Here are some common cases I have described below. These examples cover various scenarios, such as assigning single variables , multiple variable assignments in a single line , extracting values from command-line arguments , obtaining input from the user , utilizing environmental variables, etc . So let’s start.
Case 01: Single Variable Assignment
To assign a value to a single variable in Bash script , you can use the following syntax:
However, replace the variable with the name of the variable you want to assign, and the value with the desired value you want to assign to that variable.
To assign a single value to a variable in Bash , you can go in the following manner:
Steps to Follow >
❶ At first, launch an Ubuntu Terminal .
❷ Write the following command to open a file in Nano :
- nano : Opens a file in the Nano text editor.
- single_variable.sh : Name of the file.
❸ Copy the script mentioned below:
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. Next, variable var_int contains an integer value of 23 and displays with the echo command .
❹ Press CTRL+O and ENTER to save the file; CTRL+X to exit.
❺ Use the following command to make the file executable :
- chmod : changes the permissions of files and directories.
- u+x : Here, u refers to the “ user ” or the owner of the file and +x specifies the permission being added, in this case, the “ execute ” permission. When u+x is added to the file permissions, it grants the user ( owner ) permission to execute ( run ) the file.
- single_variable.sh : File name to which the permissions are being applied.
❻ Run the script by using the following command:

Case 02: Multi-Variable Assignment in a Single Line of a Bash Script
Multi-variable assignment in a single line is a concise and efficient way of assigning values to multiple variables simultaneously in Bash scripts . This method helps reduce the number of lines of code and can enhance readability in certain scenarios. Here’s an example of a multi-variable assignment in a single line.
You can follow the steps of Case 01 , to save & make the script executable.
Script (multi_variable.sh) >
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. Then, three variables x , y , and z are assigned values 1 , 2 , and 3 , respectively. The echo statements are used to print the values of each variable. Following that, two variables var1 and var2 are assigned values “ Hello ” and “ World “, respectively. The semicolon (;) separates the assignment statements within a single line. The echo statement prints the values of both variables with a space in between. Lastly, the read command is used to assign values to var3 and var4. The <<< syntax is known as a here-string , which allows the string “ Hello LinuxSimply ” to be passed as input to the read command . The input string is split into words, and the first word is assigned to var3 , while the remaining words are assigned to var4 . Finally, the echo statement displays the values of both variables.
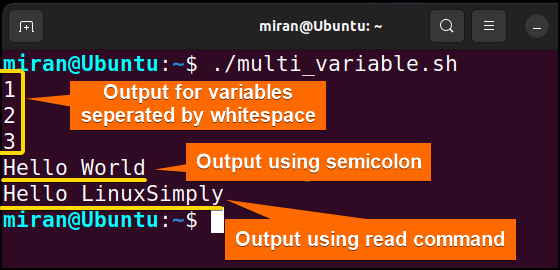
Case 03: Assigning Variables From Command-Line Arguments
In Bash , you can assign variables from command-line arguments using special variables known as positional parameters . Here is a sample code demonstrated below.
Script (var_as_argument.sh) >
The provided Bash script starts with the shebang ( #!/bin/bash ) to use Bash shell. The script assigns the first command-line argument to the variable name , the second argument to age , and the third argument to city . The positional parameters $1 , $2 , and $3 , which represent the values passed as command-line arguments when executing the script. Then, the script uses echo statements to display the values of the assigned variables.
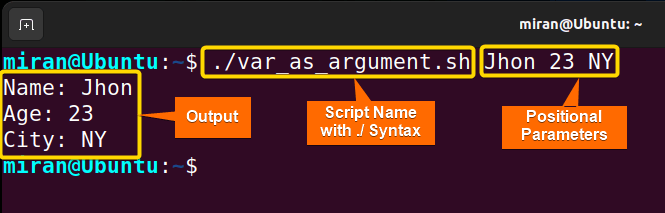
Case 04: Assign Value From Environmental Bash Variable
In Bash , you can also assign the value of an Environmental Variable to a variable. To accomplish the task you can use the following syntax :
However, make sure to replace ENV_VARIABLE_NAME with the actual name of the environment variable you want to assign. Here is a sample code that has been provided for your perusal.
Script (env_variable.sh) >
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. The value of the USER environment variable, which represents the current username, is assigned to the Bash variable username. Then the output is displayed using the echo command.
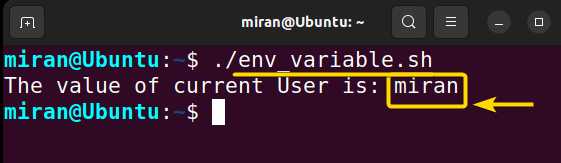
Case 05: Default Value Assignment
In Bash , you can assign default values to variables using the ${variable:-default} syntax . Note that this default value assignment does not change the original value of the variable; it only assigns a default value if the variable is empty or unset . Here’s a script to learn how it works.
Script (default_variable.sh) >
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. The next line stores a null string to the variable . The ${ variable:-Softeko } expression checks if the variable is unset or empty. As the variable is empty, it assigns the default value ( Softeko in this case) to the variable . In the second portion of the code, the LinuxSimply string is stored as a variable. Then the assigned variable is printed using the echo command .
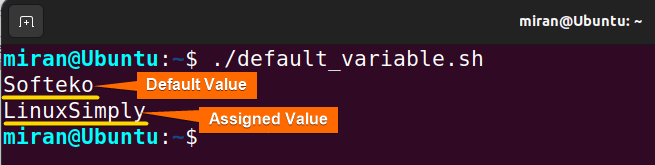
Case 06: Assigning Value by Taking Input From the User
In Bash , you can assign a value from the user by using the read command. Remember we have used this command in Case 2 . Apart from assigning value in a single line, the read command allows you to prompt the user for input and assign it to a variable. Here’s an example given below.
Script (user_variable.sh) >
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. The read command is used to read the input from the user and assign it to the name variable . The user is prompted with the message “ Enter your name: “, and the value they enter is stored in the name variable. Finally, the script displays a message using the entered value.
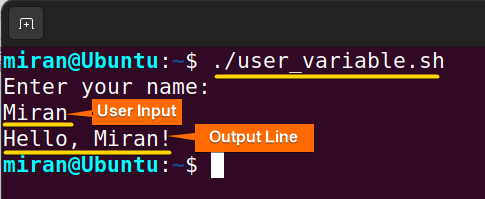
Case 07: Using the “let” Command for Variable Assignment
In Bash , the let command can be used for arithmetic operations and variable assignment. When using let for variable assignment, it allows you to perform arithmetic operations and assign the result to a variable .
Script (let_var_assign.sh) >
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. then the let command performs arithmetic operations and assigns the results to variables num. Later, the echo command has been used to display the value stored in the num variable.
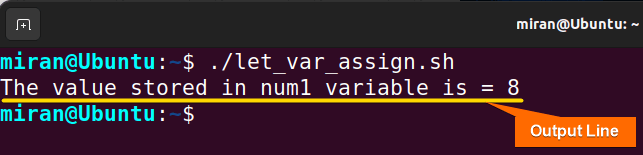
Case 08: Assigning Shell Command Output to a Variable
Lastly, you can assign the output of a shell command to a variable using command substitution . There are two common ways to achieve this: using backticks ( “) or using the $() syntax. Note that $() syntax is generally preferable over backticks as it provides better readability and nesting capability, and it avoids some issues with quoting. Here’s an example that I have provided using both cases.
Script (shell_command_var.sh) >
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. The output of the ls -l command (which lists the contents of the current directory in long format) allocates to the variable output1 using backticks . Similarly, the output of the date command (which displays the current date and time) is assigned to the variable output2 using the $() syntax . The echo command displays both output1 and output2 .
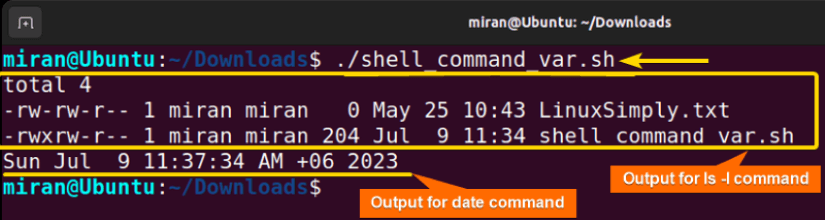
Assignment on Assigning Variables in Bash Scripts
Finally, I have provided two assignments based on today’s discussion. Don’t forget to check this out.
- Difference: ?
- Quotient: ?
- Remainder: ?
- Write a Bash script to find and display the name of the largest file using variables in a specified directory.
In conclusion, assigning variable Bash is a crucial aspect of scripting, allowing developers to store and manipulate data efficiently. This article explored several cases to assign variables in Bash, including single-variable assignments , multi-variable assignments in a single line , assigning values from environmental variables, and so on. Each case has its advantages and limitations, and the choice depends on the specific needs of the script or program. However, if you have any questions regarding this article, feel free to comment below. I will get back to you soon. Thank You!
People Also Ask
Related Articles
- How to Declare Variable in Bash Scripts? [5 Practical Cases]
- Bash Variable Naming Conventions in Shell Script [6 Rules]
- How to Check Variable Value Using Bash Scripts? [5 Cases]
- How to Use Default Value in Bash Scripts? [2 Methods]
- How to Use Set – $Variable in Bash Scripts? [2 Examples]
- How to Read Environment Variables in Bash Script? [2 Methods]
- How to Export Environment Variables with Bash? [4 Examples]
<< Go Back to Variable Declaration and Assignment | Bash Variables | Bash Scripting Tutorial

Mohammad Shah Miran
Hey, I'm Mohammad Shah Miran, previously worked as a VBA and Excel Content Developer at SOFTEKO, and for now working as a Linux Content Developer Executive in LinuxSimply Project. I completed my graduation from Bangladesh University of Engineering and Technology (BUET). As a part of my job, i communicate with Linux operating system, without letting the GUI to intervene and try to pass it to our audience.
Leave a Comment Cancel reply
Save my name, email, and website in this browser for the next time I comment.

Get In Touch!
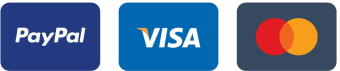
Legal Corner
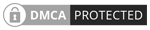
Copyright © 2024 LinuxSimply | All Rights Reserved.
Next: Grouping Commands , Previous: Looping Constructs , Up: Compound Commands [ Contents ][ Index ]
3.2.5.2 Conditional Constructs
The syntax of the if command is:
The test-commands list is executed, and if its return status is zero, the consequent-commands list is executed. If test-commands returns a non-zero status, each elif list is executed in turn, and if its exit status is zero, the corresponding more-consequents is executed and the command completes. If ‘ else alternate-consequents ’ is present, and the final command in the final if or elif clause has a non-zero exit status, then alternate-consequents is executed. The return status is the exit status of the last command executed, or zero if no condition tested true.
The syntax of the case command is:
case will selectively execute the command-list corresponding to the first pattern that matches word . The match is performed according to the rules described below in Pattern Matching . If the nocasematch shell option (see the description of shopt in The Shopt Builtin ) is enabled, the match is performed without regard to the case of alphabetic characters. The ‘ | ’ is used to separate multiple patterns, and the ‘ ) ’ operator terminates a pattern list. A list of patterns and an associated command-list is known as a clause .
Each clause must be terminated with ‘ ;; ’, ‘ ;& ’, or ‘ ;;& ’. The word undergoes tilde expansion, parameter expansion, command substitution, arithmetic expansion, and quote removal (see Shell Parameter Expansion ) before matching is attempted. Each pattern undergoes tilde expansion, parameter expansion, command substitution, arithmetic expansion, process substitution, and quote removal.
There may be an arbitrary number of case clauses, each terminated by a ‘ ;; ’, ‘ ;& ’, or ‘ ;;& ’. The first pattern that matches determines the command-list that is executed. It’s a common idiom to use ‘ * ’ as the final pattern to define the default case, since that pattern will always match.
Here is an example using case in a script that could be used to describe one interesting feature of an animal:
If the ‘ ;; ’ operator is used, no subsequent matches are attempted after the first pattern match. Using ‘ ;& ’ in place of ‘ ;; ’ causes execution to continue with the command-list associated with the next clause, if any. Using ‘ ;;& ’ in place of ‘ ;; ’ causes the shell to test the patterns in the next clause, if any, and execute any associated command-list on a successful match, continuing the case statement execution as if the pattern list had not matched.
The return status is zero if no pattern is matched. Otherwise, the return status is the exit status of the command-list executed.
The select construct allows the easy generation of menus. It has almost the same syntax as the for command:
The list of words following in is expanded, generating a list of items, and the set of expanded words is printed on the standard error output stream, each preceded by a number. If the ‘ in words ’ is omitted, the positional parameters are printed, as if ‘ in "$@" ’ had been specified. select then displays the PS3 prompt and reads a line from the standard input. If the line consists of a number corresponding to one of the displayed words, then the value of name is set to that word. If the line is empty, the words and prompt are displayed again. If EOF is read, the select command completes and returns 1. Any other value read causes name to be set to null. The line read is saved in the variable REPLY .
The commands are executed after each selection until a break command is executed, at which point the select command completes.
Here is an example that allows the user to pick a filename from the current directory, and displays the name and index of the file selected.
The arithmetic expression is evaluated according to the rules described below (see Shell Arithmetic ). The expression undergoes the same expansions as if it were within double quotes, but double quote characters in expression are not treated specially are removed. If the value of the expression is non-zero, the return status is 0; otherwise the return status is 1.
Return a status of 0 or 1 depending on the evaluation of the conditional expression expression . Expressions are composed of the primaries described below in Bash Conditional Expressions . The words between the [[ and ]] do not undergo word splitting and filename expansion. The shell performs tilde expansion, parameter and variable expansion, arithmetic expansion, command substitution, process substitution, and quote removal on those words (the expansions that would occur if the words were enclosed in double quotes). Conditional operators such as ‘ -f ’ must be unquoted to be recognized as primaries.
When used with [[ , the ‘ < ’ and ‘ > ’ operators sort lexicographically using the current locale.
When the ‘ == ’ and ‘ != ’ operators are used, the string to the right of the operator is considered a pattern and matched according to the rules described below in Pattern Matching , as if the extglob shell option were enabled. The ‘ = ’ operator is identical to ‘ == ’. If the nocasematch shell option (see the description of shopt in The Shopt Builtin ) is enabled, the match is performed without regard to the case of alphabetic characters. The return value is 0 if the string matches (‘ == ’) or does not match (‘ != ’) the pattern, and 1 otherwise.
If you quote any part of the pattern, using any of the shell’s quoting mechanisms, the quoted portion is matched literally. This means every character in the quoted portion matches itself, instead of having any special pattern matching meaning.
An additional binary operator, ‘ =~ ’, is available, with the same precedence as ‘ == ’ and ‘ != ’. When you use ‘ =~ ’, the string to the right of the operator is considered a POSIX extended regular expression pattern and matched accordingly (using the POSIX regcomp and regexec interfaces usually described in regex (3)). The return value is 0 if the string matches the pattern, and 1 if it does not. If the regular expression is syntactically incorrect, the conditional expression returns 2. If the nocasematch shell option (see the description of shopt in The Shopt Builtin ) is enabled, the match is performed without regard to the case of alphabetic characters.
You can quote any part of the pattern to force the quoted portion to be matched literally instead of as a regular expression (see above). If the pattern is stored in a shell variable, quoting the variable expansion forces the entire pattern to be matched literally.
The pattern will match if it matches any part of the string. If you want to force the pattern to match the entire string, anchor the pattern using the ‘ ^ ’ and ‘ $ ’ regular expression operators.
For example, the following will match a line (stored in the shell variable line ) if there is a sequence of characters anywhere in the value consisting of any number, including zero, of characters in the space character class, immediately followed by zero or one instances of ‘ a ’, then a ‘ b ’:
That means values for line like ‘ aab ’, ‘ aaaaaab ’, ‘ xaby ’, and ‘ ab ’ will all match, as will a line containing a ‘ b ’ anywhere in its value.
If you want to match a character that’s special to the regular expression grammar (‘ ^$|[]()\.*+? ’), it has to be quoted to remove its special meaning. This means that in the pattern ‘ xxx.txt ’, the ‘ . ’ matches any character in the string (its usual regular expression meaning), but in the pattern ‘ "xxx.txt" ’, it can only match a literal ‘ . ’.
Likewise, if you want to include a character in your pattern that has a special meaning to the regular expression grammar, you must make sure it’s not quoted. If you want to anchor a pattern at the beginning or end of the string, for instance, you cannot quote the ‘ ^ ’ or ‘ $ ’ characters using any form of shell quoting.
If you want to match ‘ initial string ’ at the start of a line, the following will work:
but this will not:
because in the second example the ‘ ^ ’ is quoted and doesn’t have its usual special meaning.
It is sometimes difficult to specify a regular expression properly without using quotes, or to keep track of the quoting used by regular expressions while paying attention to shell quoting and the shell’s quote removal. Storing the regular expression in a shell variable is often a useful way to avoid problems with quoting characters that are special to the shell. For example, the following is equivalent to the pattern used above:
Shell programmers should take special care with backslashes, since backslashes are used by both the shell and regular expressions to remove the special meaning from the following character. This means that after the shell’s word expansions complete (see Shell Expansions ), any backslashes remaining in parts of the pattern that were originally not quoted can remove the special meaning of pattern characters. If any part of the pattern is quoted, the shell does its best to ensure that the regular expression treats those remaining backslashes as literal, if they appeared in a quoted portion.
The following two sets of commands are not equivalent:
The first two matches will succeed, but the second two will not, because in the second two the backslash will be part of the pattern to be matched. In the first two examples, the pattern passed to the regular expression parser is ‘ \. ’. The backslash removes the special meaning from ‘ . ’, so the literal ‘ . ’ matches. In the second two examples, the pattern passed to the regular expression parser has the backslash quoted (e.g., ‘ \\\. ’), which will not match the string, since it does not contain a backslash. If the string in the first examples were anything other than ‘ . ’, say ‘ a ’, the pattern would not match, because the quoted ‘ . ’ in the pattern loses its special meaning of matching any single character.
Bracket expressions in regular expressions can be sources of errors as well, since characters that are normally special in regular expressions lose their special meanings between brackets. However, you can use bracket expressions to match special pattern characters without quoting them, so they are sometimes useful for this purpose.
Though it might seem like a strange way to write it, the following pattern will match a ‘ . ’ in the string:
The shell performs any word expansions before passing the pattern to the regular expression functions, so you can assume that the shell’s quoting takes precedence. As noted above, the regular expression parser will interpret any unquoted backslashes remaining in the pattern after shell expansion according to its own rules. The intention is to avoid making shell programmers quote things twice as much as possible, so shell quoting should be sufficient to quote special pattern characters where that’s necessary.
The array variable BASH_REMATCH records which parts of the string matched the pattern. The element of BASH_REMATCH with index 0 contains the portion of the string matching the entire regular expression. Substrings matched by parenthesized subexpressions within the regular expression are saved in the remaining BASH_REMATCH indices. The element of BASH_REMATCH with index n is the portion of the string matching the n th parenthesized subexpression.
Bash sets BASH_REMATCH in the global scope; declaring it as a local variable will lead to unexpected results.
Expressions may be combined using the following operators, listed in decreasing order of precedence:
Returns the value of expression . This may be used to override the normal precedence of operators.
True if expression is false.
True if both expression1 and expression2 are true.
True if either expression1 or expression2 is true.
The && and || operators do not evaluate expression2 if the value of expression1 is sufficient to determine the return value of the entire conditional expression.
How-To Geek
How to use bash if statements (with 4 examples).
The if statement is the easiest way to explore conditional execution in Bash scripts.
Quick Links
What is conditional execution, a simple if statement example, the elif clause, different forms of conditional test, nested if statements, the case for if, key takeaways.
Use the Linux Bash if statement to build conditional expressions with an if then fi structure. Add elif keywords for additional conditional expressions, or the else keyword to define a catch-all section of code that's executed if no previous conditional clause was executed.
All non-trivial Bash scripts need to make decisions. The Bash if statement lets your Linux script ask questions and, depending on the answer, run different sections of code. Here's how they work.
In all but the most trivial of Bash scripts , there's usually a need for the flow of execution to take a different path through the script, according to the outcome of a decision. This is called conditional execution.
One way to decide which branch of execution to take is to use an if statement. You might hear
statements called
statements, or
statements. They're different names for the same thing.
Related: 9 Examples of for Loops in Linux Bash Scripts
The if statement says that if something is true, then do this. But if the something is false, do that instead. The "something" can be many things, such as the value of a variable, the presence of a file , or whether two strings match.
Conditional execution is vital for any meaningful script. Without it, you're very limited in what you can get your script to do. Unless it can make meaningful decisions you won't be able to take real-world problems and produce workable solutions.
The if statement is probably the most frequently used means of obtaining conditional execution. Here's how to use it in Bash scripting.
Related: How to Check If a File Exists in Linux Bash Scripts
This is the canonical form of the simplest if statement:
if [ this-condition-is-true ]
execute-these-statements
If the condition within the text resolves to true, the lines of script in the then clause are executed. If you're looking through scripts written by others, you may see the if statement written like this:
if [ this-condition-is-true ]; then
Some points to note:
- The if statement is concluded by writing fi .
- There must be a space after the first bracket " [ " and before the second bracket " ] " of the conditional test.
- If you're going to put the then keyword on the same line as the conditional test, make sure you use a semi-colon " ; " after the test.
We can add an optional else clause to have some code executed if the condition test proves to be false. The else clause doesn't need a then keyword.
execute-these-statements-instead
This script shows a simple example of an if statement that uses an else clause. The conditional test checks whether the customer's age is greater or equal to 21. If it is, the customer can enter the premises, and the then clause is executed. If they're not old enough, the else clause is executed, and they're not allowed in.
#!/bin/bash
customer_age=25
if [ $customer_age -ge 21 ]
echo "Come on in."
echo "You can't come in."
Copy the script from above into an editor, save it as a file called "if-age.sh", and use the chmod command to make it executable. You'll need to do that with each of the scripts we discuss.
chmod +x if-age.sh
Let's run our script.
./if-age.sh
Now we'll edit the file and use an age less than 21.
customer_age=18
Make that change to your script, and save your changes. If we run it now the condition returns false, and the else clause is executed.
The elif clause adds additional conditional tests. You can have as many elif clauses as you like. They're evaluated in turn until one of them is found to be true. If none of the elif conditional tests prove to be true, the else clause, if present, is executed.
This script asks for a number then tells you if it is odd or even. Zero is an even number , so we don't need to test anything.
All other numbers are tested by finding the modulo of a division by two. In our case, the modulo is the fractional part of the result of a division by two. If there is no fractional part, the number is divisible by two, exactly. Therefore it is an even number.
echo -n "Enter a number: "
read number
if [ $number -eq 0 ]
echo "You entered zero. Zero is an even number."
elif [ $(($number % 2)) -eq 0 ]
echo "You entered $number. It is an even number."
echo "You entered $number. It is an odd number."
To run this script, copy it to an editor and save it as "if-even.sh", then use chmod to make it executable.
Let's run the script a few times and check its output.
./if-even.sh
That's all working fine.
The brackets " [] " we've used for our conditional tests are a shorthand way of calling the test program. Because of that, all the comparisons and tests that test supports are available to your if statement.
This is just a few of them :
- ! expression : True if the expression is false.
- -n string : True if the length of the string is greater than zero.
- -z string : True if the length of the string is zero. That is, it's an empty string.
- string1 = string2 : True if string1 is the same as string2.
- string1 != string2 : True if string1 is not the same as string2.
- integer1 -eq integer2 : True if integer1 is numerically equal to integer2
- integer1 -qt integer2 : True if integer1 is numerically greater than integer2
- integer1 -lt integer2 : True if integer1 is numerically less than integer2
- -d directory : True if the directory exists.
- -e file : True if the file exists.
- -s file : True if the file exists with a size of more than zero.
- -r file : True if the file exists and the read permission is set.
- -w file : True if the file exists and the write permission is set.
- -x file : True if the file exists and the execute permission is set.
In the table, "file" and "directory" can include directory paths, either relative or absolute.
The equals sign " = " and the equality test -eq are not the same. The equals sign performs a character by character text comparison. The equality test performs a numerical comparison.
We can see this by using the test program on the command line.
test "this string" = "this string"
test "this string" = "that string"
test 1 = 001
test 1 -eq 001
In each case, we use the echo command to print the return code of the last command. Zero means true, one means false.
Using the equals sign " = " gives us a false response comparing 1 to 001. That's correct, because they're two different strings of characters. Numerically they're the same value---one---so the -eq operator returns a true response.
If you want to use wildcard matching in your conditional test, use the double bracket " [[ ]] " syntax.
if [[ $USER == *ve ]]
echo "Hello $USER"
echo "$USER does not end in 've'"
This script checks the current user's account name. If it ends in " ve ", it prints the user name. If it doesn't end in " ve ", the script tells you so, and ends.
./if-wild.sh
You can put an if statement inside another if statement.
This is perfectly acceptable, but nesting if statements makes for code that is less easy to read, and more difficult to maintain. If you find yourself nesting more than two or three levels of if statements, you probably need to reorganize the logic of your script.
Here's a script that gets the day as a number, from one to seven. One is Monday, seven is Sunday.
It tells us a shop's opening hours. If it is a weekday or Saturday, it reports the shop is open. If it is a Sunday, it reports that the shop is closed.
If the shop is open, the nested if statement makes a second test. If the day is Wednesday, it tells us it is open in the morning only.
# get the day as a number 1..7
day=$(date +"%u")
if [ $day -le 6 ]
## the shop is open
if [ $day -eq 3 ]
# Wednesday is half-day
echo "On Wednesdays we open in the morning only."
# regular week days and Saturday
echo "We're open all day."
# not open on Sundays
echo "It's Sunday, we're closed."
Copy this script into an editor, save it as a file called "if-shop.sh", and make it executable using the chmod command.
We ran the script once and then changed the computer's clock to be a Wednesday, and re-ran the script. We then changed the day to a Sunday and ran it once more.
./if-shop.sh
Related: How to Use Double Bracket Conditional Tests in Linux
Conditional execution is what brings power to programming and scripting, and the humble if statement might well be the most commonly used way of switching the path of execution within code. But that doesn't mean it's always the answer.
Writing good code means knowing what options you have and which are the best ones to use in order to solve a particular requirement. The if statement is great, but don't let it be the only tool in your bag. In particular, check out the case statement which can be a solution in some scenarios.
Related: How to Use Case Statements in Bash Scripts

CNN Communications Press Releases
Cnn to host 2024 election presidential debate between president joe biden and former president donald j. trump on june 27.
Debate To Air Live on CNN, CNN International, CNN en Español, CNN Max and CNN.com
Washington, DC – (May 15, 2024) – CNN will host an election debate between President Joe Biden and former President Donald J. Trump on June 27, 2024 at 9pm ET from the crucial battleground state of Georgia.
The debate will be held in CNN’s Atlanta studios. CNN Anchors Jake Tapper and Dana Bash will serve as moderators. To ensure candidates may maximize the time allotted in the debate, no audience will be present. Additional details will be announced at a later date.
To qualify for participation, candidates must fulfill the requirements outlined in Article II, Section 1 of the Constitution of the United States; file a Statement of Candidacy with the Federal Election Commission; a candidate’s name must appear on a sufficient number of state ballots to reach the 270 electoral vote threshold to win the presidency prior to the eligibility deadline; agree to accept the rules and format of the debate; and receive at least 15% in four separate national polls of registered or likely voters that meet CNN’s standards for reporting.
Polls that meet CNN editorial standards and will be considered qualifying polls include those sponsored by: CNN, ABC News, CBS News, Fox News, Marquette University Law School, Monmouth University, NBC News, the New York Times/Siena College, NPR/PBS NewsHour/Marist College, Quinnipiac University, the Wall Street Journal, and the Washington Post.
The polling window to determine eligibility for the debate opened March 13, 2024, and closes seven days before the date of the debate.
PRESS CONTACTS: [email protected] [email protected]
MEDIA CREDENTIALING INQUIRIES: [email protected]
About CNN Worldwide CNN Worldwide is the most honored brand in cable news, reaching more individuals through television, streamingand online than any other cable news organization in the United States. Globally, people across the world can watch CNN International, which is widely distributed in over 200 countries and territories. CNN Digital is the #1 online news destination, with more unique visitors than any other news source. Max, Warner Bros. Discovery’s streaming platform, features CNN Max, a 24/7 streaming news offering available to subscribers alongside expanded access to News content and CNN Originals. CNN’s award-winning portfolio includes non-scripted programming from CNN Original Series and CNN Films for broadcast, streaming and distribution across multiple platforms. CNN programming can be found on CNN, CNN International and CNN en Español channels, via CNN Max and the CNN Originals hub on discovery+ and via pay TV subscription on CNN.com, CNN apps and cable operator platforms. Additionally, CNN Newsource is the world’s most extensively utilized news service partnering with over 1,000 local and international news organizations around the world. CNN is a division of Warner Bros. Discovery.
About Warner Bros. Discovery Warner Bros. Discovery is a leading global media and entertainment company that creates and distributes the world’s most differentiated and complete portfolio of branded content across television, film, streaming and gaming. Available in more than 220 countries and territories and 50 languages, Warner Bros. Discovery inspires, informs and entertains audiences worldwide through its iconic brands and products including: Discovery Channel, Max, discovery+, CNN, DC, TNT Sports, Eurosport, HBO, HGTV, Food Network, OWN, Investigation Discovery, TLC, Magnolia Network, TNT, TBS, truTV, Travel Channel, MotorTrend, Animal Planet, Science Channel, Warner Bros. Motion Picture Group, Warner Bros. Television Group, Warner Bros. Pictures Animation, Warner Bros. Games, New Line Cinema, Cartoon Network, Adult Swim, Turner Classic Movies, Discovery en Español, Hogar de HGTV and others. For more information, please visit www.wbd.com .
Sandy Springs school issues statement after Hitler assignment controversy
ATLANTA, Ga. (Atlanta News First) - A metro Atlanta school is responding to backlash to a controversial assignment.
Parents are fuming and questioning the Mount Vernon School in Sandy Springs after their kids were asked about Hitler’s leadership.
The assignment asked students to rate the attributes of the former Nazi dictator. The school now acknowledges this caused distress and the assignment has now been removed from the curriculum.
Head of School Kristy Lundstrom sent the following statement to Mount Vernon parents:
Dear Mount Vernon Family, I am aware that a screenshot is being circulated on social media and has caused concern and distress. The screenshot is a snapshot taken out of context from a classroom assignment from March 2024. We do not condone positive labels for Adolf Hitler. The intent of the assignment was an exploration of World War II designed to boost student knowledge of factual events and understand the manipulation of fear leveraged by Adolf Hitler in connection to the Treaty of Versailles. When leadership was made aware of how the assignment was written it was removed from the curriculum. Immediately following this incident, I met with the School’s Chief of Inclusion, Diversity, Equity, and Action, Head of Middle School, and a concerned Rabbi and friend of the School who shared the perspective of some of our families and supported us in a thorough review of the assignment and community impact. We worked to understand the balance of holding a safe environment for students while doing the important work of sharing history to prevent deniers and to be clear about the atrocities committed. Adolf Hitler and the events of this time period are difficult and traumatic to discuss. In this very heavy time, when our Jewish students and families have the added stress of world events, we want to be clear that The Mount Vernon School wholeheartedly denounces antisemitism. Our commitment is to embrace a diverse community where every individual is seen, valued, and heard. Please know that we are here to care for and support your children. We have counselors, faith leaders, and school leaders who have been and will continue to be here to talk to, listen to, and lift up your children. Kristy Lundstrom
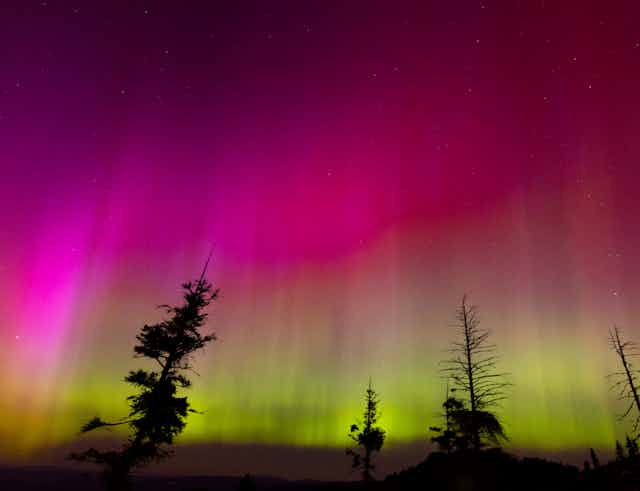
What causes the different colours of the aurora? An expert explains the electric rainbow
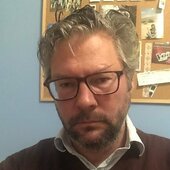
Professor of Chemistry, UNSW Sydney
Disclosure statement
Timothy Schmidt receives funding from the Australian Research Council and the Australian Renewable Energy Agency.
UNSW Sydney provides funding as a member of The Conversation AU.
View all partners
Last week, a huge solar flare sent a wave of energetic particles from the Sun surging out through space. Over the weekend, the wave reached Earth, and people around the world enjoyed the sight of unusually vivid aurora in both hemispheres.
While the aurora is normally only visible close to the poles, this weekend it was spotted as far south as Hawaii in the northern hemisphere, and as far north as Mackay in the south.
This spectacular spike in auroral activity appears to have ended, but don’t worry if you missed out. The Sun is approaching the peak of its 11-year sunspot cycle , and periods of intense aurora are likely to return over the next year or so.
If you saw the aurora, or any of the photos, you might be wondering what exactly was going on. What makes the glow, and the different colours? The answer is all about atoms, how they get excited – and how they relax.
When electrons meet the atmosphere
The auroras are caused by charged subatomic particles (mostly electrons) smashing into Earth’s atmosphere. These are emitted from the Sun all the time, but there are more during times of greater solar activity.
Most of our atmosphere is protected from the influx of charged particles by Earth’s magnetic field. But near the poles, they can sneak in and wreak havoc.
Earth’s atmosphere is about 20% oxygen and 80% nitrogen, with some trace amounts of other things like water, carbon dioxide (0.04%) and argon.
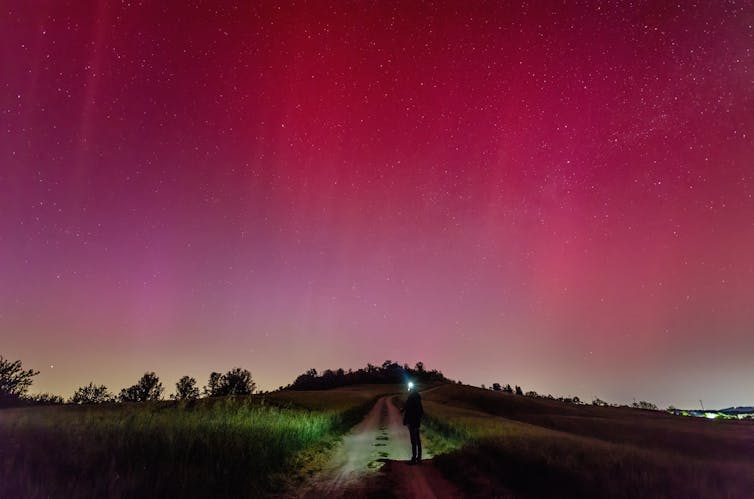
When high-speed electrons smash into oxygen molecules in the upper atmosphere, they split the oxygen molecules (O₂) into individual atoms. Ultraviolet light from the Sun does this too, and the oxygen atoms generated can react with O₂ molecules to produce ozone (O₃), the molecule that protects us from harmful UV radiation.
But, in the case of the aurora, the oxygen atoms generated are in an excited state. This means the atoms’ electrons are arranged in an unstable way that can “relax” by giving off energy in the form of light.
What makes the green light?
As you see in fireworks, atoms of different elements produce different colours of light when they are energised.
Copper atoms give a blue light, barium is green, and sodium atoms produce a yellow–orange colour that you may also have seen in older street lamps. These emissions are “allowed” by the rules of quantum mechanics, which means they happen very quickly.
When a sodium atom is in an excited state it only stays there for around 17 billionths of a second before firing out a yellow–orange photon.
But, in the aurora, many of the oxygen atoms are created in excited states with no “allowed” ways to relax by emitting light. Nevertheless, nature finds a way.
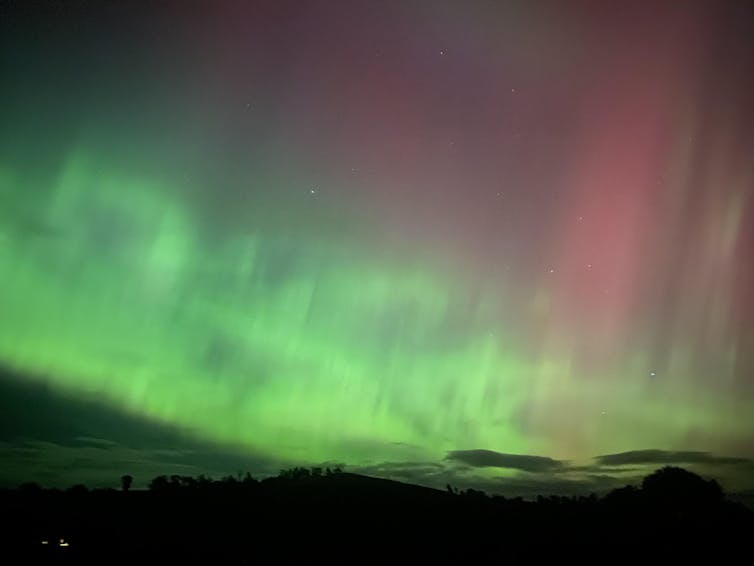
The green light that dominates the aurora is emitted by oxygen atoms relaxing from a state called “¹S” to a state called “¹D”. This is a relatively slow process, which on average takes almost a whole second.
In fact, this transition is so slow it won’t usually happen at the kind of air pressure we see at ground level, because the excited atom will have lost energy by bumping into another atom before it has a chance to send out a lovely green photon. But in the atmosphere’s upper reaches, where there is lower air pressure and therefore fewer oxygen molecules, they have more time before bumping into one another and therefore have a chance to release a photon.
For this reason, it took scientists a long time to figure out that the green light of the aurora was coming from oxygen atoms. The yellow–orange glow of sodium was known in the 1860s, but it wasn’t until the 1920s that Canadian scientists figured out the auroral green was due to oxygen.
What makes the red light?
The green light comes from a so-called “forbidden” transition, which happens when an electron in the oxygen atom executes an unlikely leap from one orbital pattern to another. (Forbidden transitions are much less probable than allowed ones, which means they take longer to occur.)
However, even after emitting that green photon, the oxygen atom finds itself in yet another excited state with no allowed relaxation. The only escape is via another forbidden transition, from the ¹D to the ³P state – which emits red light.
This transition is even more forbidden, so to speak, and the ¹D state has to survive for about about two minutes before it can finally break the rules and give off red light. Because it takes so long, the red light only appears at high altitudes, where the collisions with other atoms and molecules are scarce.
Also, because there is such a small amount of oxygen up there, the red light tends to appear only in intense auroras – like the ones we have just had.
This is why the red light appears above the green. While they both originate in forbidden relaxations of oxygen atoms, the red light is emitted much more slowly and has a higher chance of being extinguished by collisions with other atoms at lower altitudes.

Other colours, and why cameras see them better
While green is the most common colour to see in the aurora, and red the second most common, there are also other colours. In particular, ionised nitrogen molecules (N₂⁺, which are missing one electron and have a positive electrical charge), can emit blue and red light. This can produce a magenta hue at low altitudes.
All these colours are visible to the naked eye if the aurora is bright enough. However, they show up with more intensity in the camera lens.
There are two reasons for this. First, cameras have the benefit of a long exposure, which means they can spend more time collecting light to produce an image than our eyes can. As a result, they can make a picture in dimmer conditions.
The second is that the colour sensors in our eyes don’t work very well in the dark – so we tend to see in black and white in low light conditions. Cameras don’t have this limitation.
Not to worry, though. When the aurora is bright enough, the colours are clearly visible to the naked eye.
Read more: What are auroras, and why do they come in different shapes and colours? Two experts explain
- Quantum mechanics
- Space weather
- Aurora borealis
- Aurora Australis

Case Management Specialist

Lecturer / Senior Lecturer - Marketing

Assistant Editor - 1 year cadetship

Executive Dean, Faculty of Health

Lecturer/Senior Lecturer, Earth System Science (School of Science)
The Best Nails From the 2024 Met Gala Red Carpet
Diamonds (and 3D flowers) are a girl’s best friend.
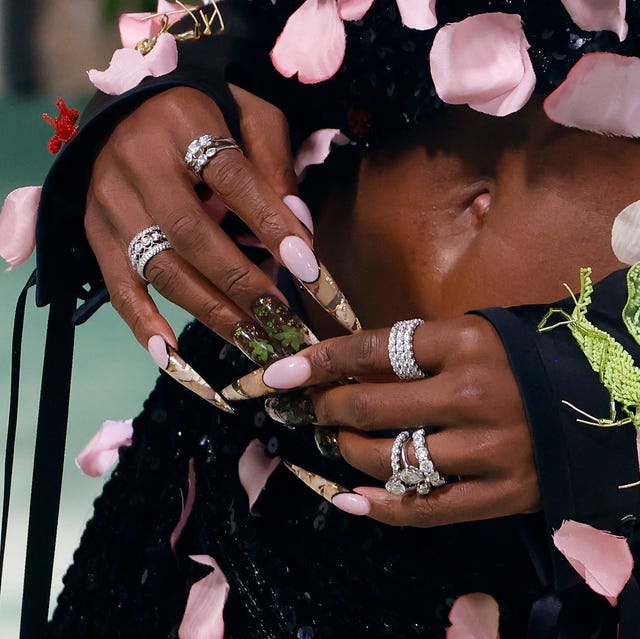
Every item on this page was chosen by an ELLE editor. We may earn commission on some of the items you choose to buy.
As usual, there were plenty of classic nudes and French tips that made an appearance at the bash, but there was also a wealth of creativity that inspired us to redo our nail Pinterest boards for the rest of spring. One particular trend that caught our eye was 3D floral nails , like the red and pink flowers adorning Dove Cameron’s mani. Plenty of glitz and glam was present, too: Da’Vine Joy Randolph and Taraji P. Henson’s nail moments featured gemstone-like charms that sparkled under the lights and camera flashes. Meanwhile, Cardi stuck to her roots with towering stiletto claws.
Ahead, click through the best nails we saw at the 2024 Met Gala—they’ll take your breath away and have you scheduling your next nail appointment ASAP.
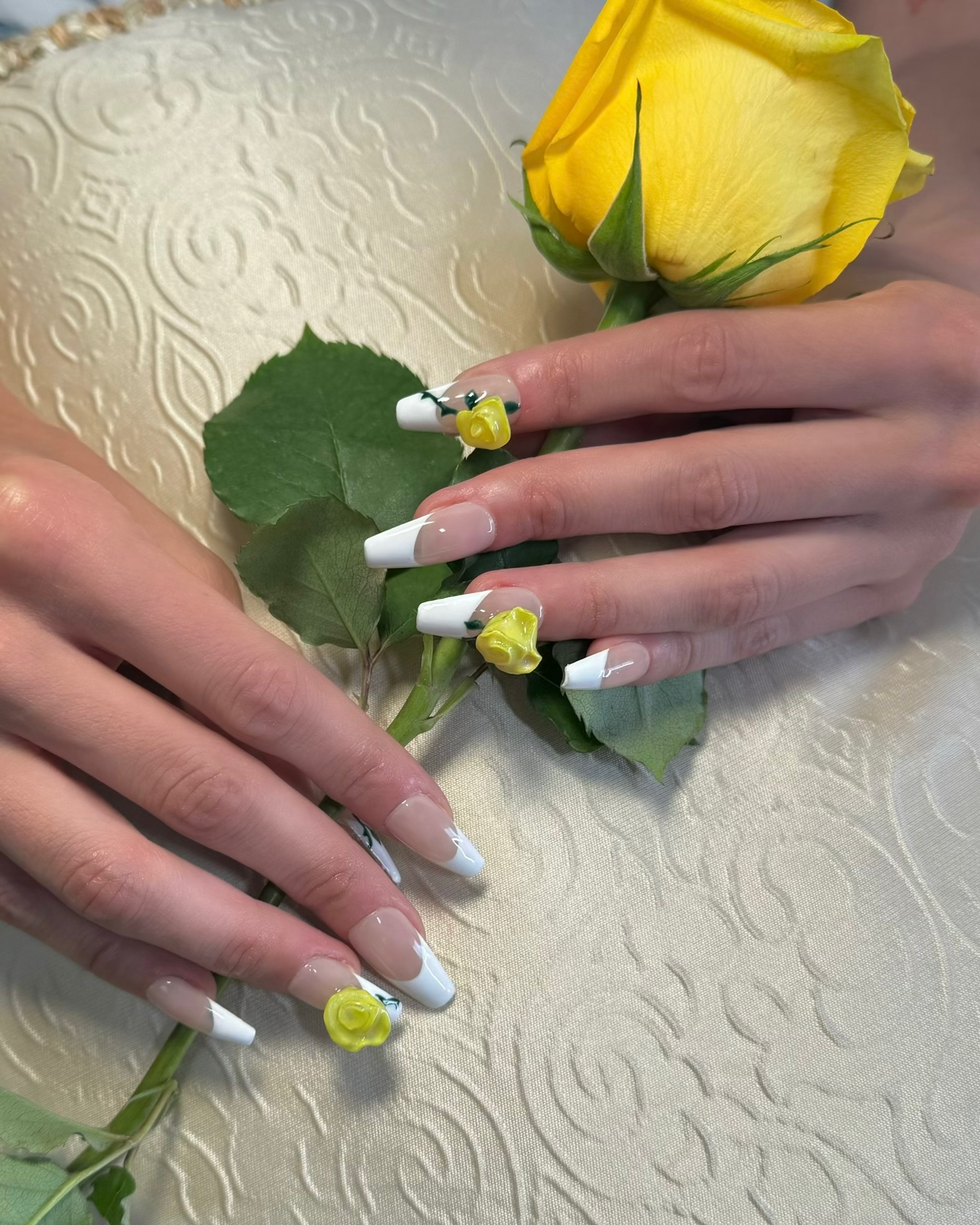
Belle from Beauty and the Beast would’ve loved Gigi's garden nails. Manicurist Mei Kawajiri composed a classic, spring-fresh nail with Nailboo’s Nail-Flex & Chill and Here’s A Tip before hand-sculpting the mesmerizing yellow roses on top. “This mix of playful and classic is just like Gigi. We wanted the nail looks to be a continuation of her Met Gala look designed by Thom Browne,” Kawajiri said in a press release. Truly a manicure fit for a princess.
Taraji P. Henson
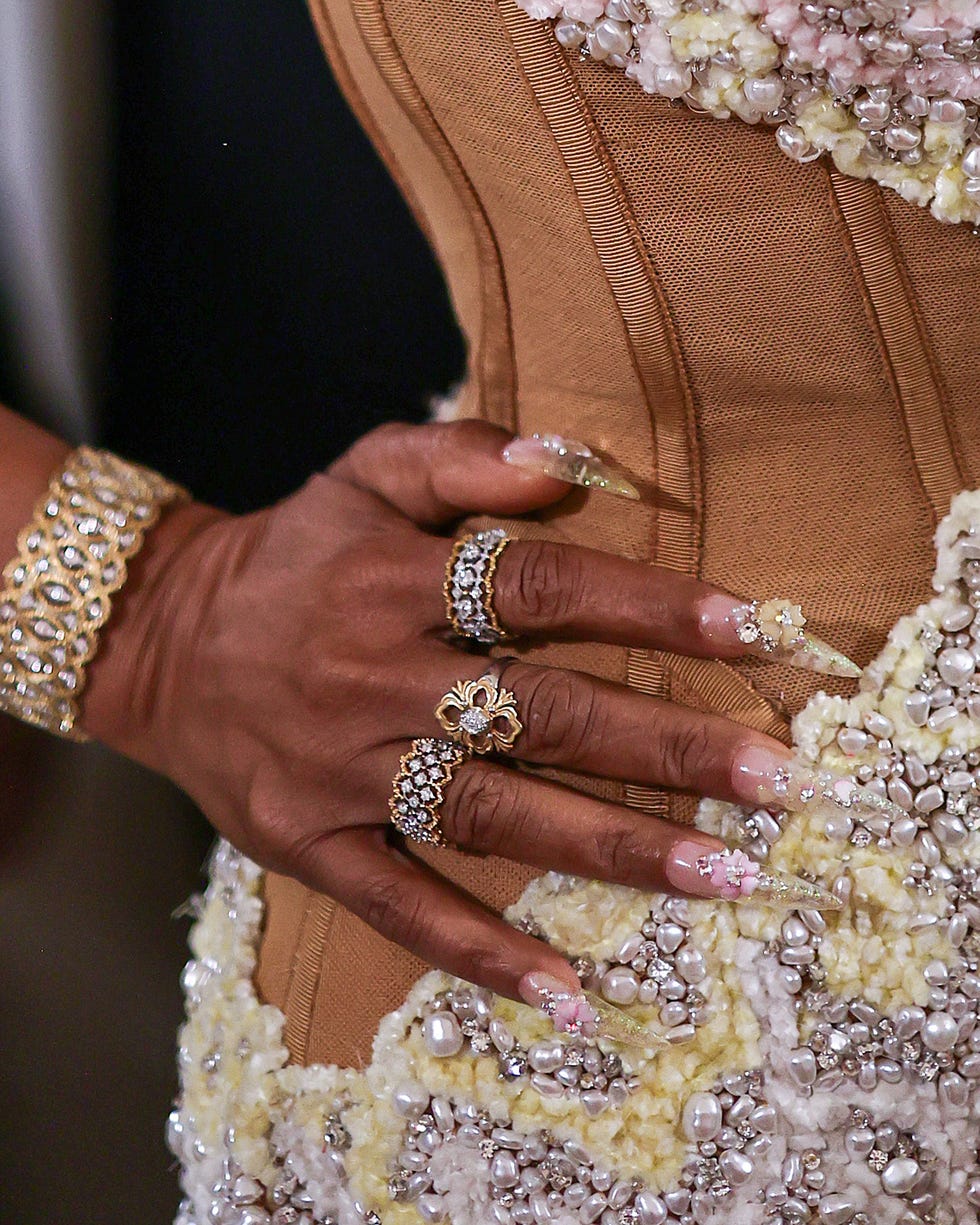
Taraji P. Henson knows how to accessorize and make a statement. Her nails, encrusted with flowers and gemstones, seemed more like an extension of her jewelry than a manicure.
Cynthia Erivo
Natural elements like dried beetles, rose petals, and succulents, along with flakes of gold, adorned Cynthia Erivo’s nails. Layers of OPI GelColor Stay Strong Base Coat , GelColor in Midnight Snacc , and GelColor in Bubble Bath held the decorations in place, like amber encasing fossilized bugs.
Da’Vine Joy Randolph
Da’Vine Joy Randolph’s sweeping denim gown stole the show, but her bejeweled blue nails deserved a closer look, too.
Allison Williams
Press-on nails have come a long way since the bulky, ill-fitting offerings of our youth, and believe it or not, Allison Williams was sporting a set of imPRESS No Glue Manicures in Vanilla Glazed on the red carpet. “After seeing a swatch of Allison’s gown, I knew I wanted to create a design that would flow across her hand. Following the floral pattern in her gown, I created a cascading rosette design with vines that connected from nail to nail. Allison fell in love with them the moment she saw them!” said celebrity manicurist Pattie Yankee in a press release.
Ayo Edebiri
Loewe dressed Ayo Edebiri, and Après Nails’ First Glance , Aquarius Rising , and Be-Leaf In Me dressed her nails. Her manicurist, Eri Ishizu, then built the 3D flowers with Top Gelcoat X and supplemented them with pearls, studs, and actual dried blooms.
Sabrina Carpenter
Sabrina Carpenter was feeling blue on the 2024 Met Gala red carpet. “The black and blue fairytale gown she wore is so elegant and classic. To complement the look, I used OPI It’s a Boy! with Chrome Effects in Tin Man Can and adorned the nails with rhinestones,” celebrity nail artist Zola Ganzorigt shared via press release.
Dove Cameron
Manicurist Melanie Shengaris made sure that each of the floral charms on Dove Cameron’s nails matched the actress and singer’s gown. Aprés Nails Dear Diary provided the milky pink base.
All that glitters is not gold, but Mona Patel’s nails sure were. The gilded look matched the gold details on the rest of her ethereal ensemble.
Quannah Chasinghorse
Hailey Bieber kicked off the glazed donut nail trend at the 2022 Met Gala, and Quannah Chasinghorse put her own twist on it this year by adding tiny hand-painted blooms on her fourth fingers.
Cardi B’s nail artist, Coca Michelle, took structured manicures to a whole other level. Fun fact: The three-dimensional nails took more than 20 hours to construct. “I went with a simple design to give the color and structure of the nail a moment and an elevated look of a thorn on a rose stem,” Michelle said in a press release. While OPI shades Spice Up Your Life and Samoan Sand provided the neutral base, a combination of Is That a Spear in Your Pocket? And AmazON…AmazOFF was responsible for the deep green tips.
Keke Palmer
Bling was the name of the game for Keke Palmer on the 2024 Met Gala red carpet. Tiny gems studded the actress’s nude stiletto manicure.
Best Nail Colors, Designs, and Trends
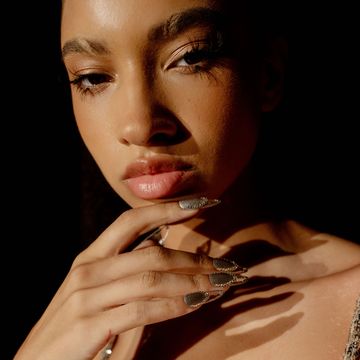
2024 Nail Trends According to Experts
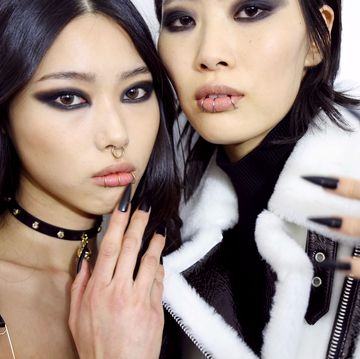
The 17 Best UV Nail Lamps for At-Home Manicures
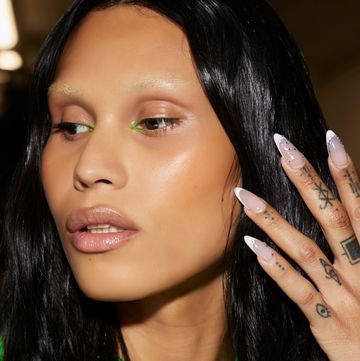
36 New Ways to Wear a French Manicure
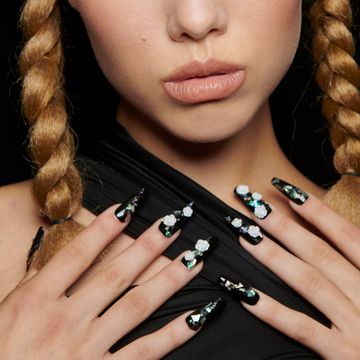
Fall Into Florals With These 3D Nail Designs
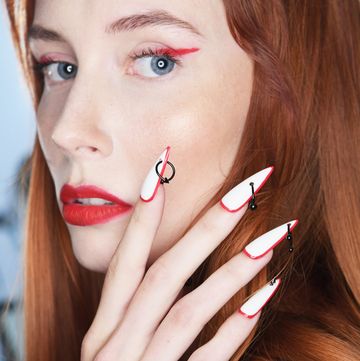
45 Spooky Halloween Nail Art Ideas
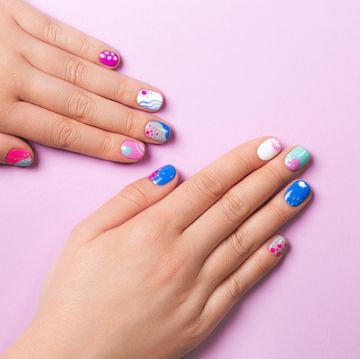
Dip Nail Design Ideas to Inspire Your Next Mani
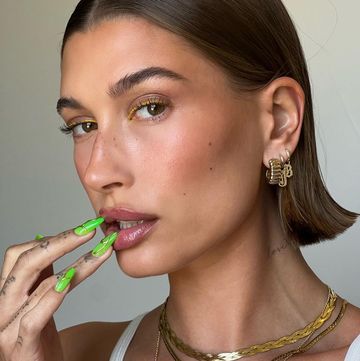
How to Get Hailey Bieber's Glow-in-the-Dark Nails
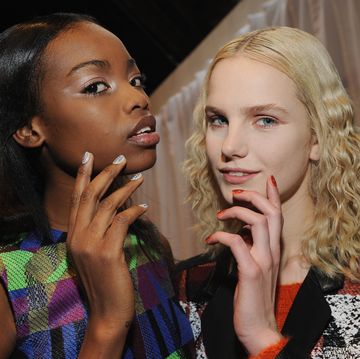
4 Nails Colors to Cure Your Winter Blues
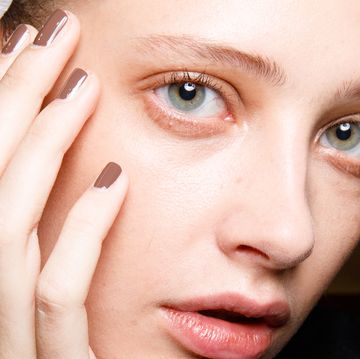
9 Winter Nail Colors to Match an Espresso Martini
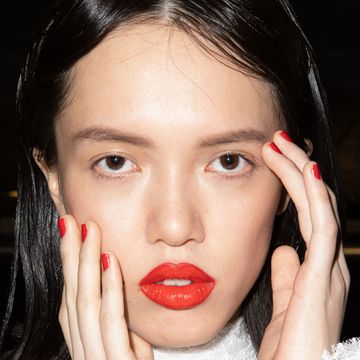
13 Nail Colors ELLE Editors Are Wearing This Fall
25 Non-Literal Thanksgiving Nail Designs
Ga. school retracts controversial class assignment on Hitler
ATLANTA, Ga. (Atlanta News First) - A metro Atlanta school is responding to backlash to a controversial assignment.
Parents are fuming and questioning the Mount Vernon School in Sandy Springs after their kids were asked about Hitler’s leadership.
The assignment asked students to rate the attributes of the former Nazi dictator. The school now acknowledges this caused distress and the assignment has now been removed from the curriculum.
MORE | Biden’s speech roils Morehouse College, a center of Black politics and culture
When he gives the commencement address at Morehouse College, President Joe Biden will have his most direct engagement with college students since the start of the Israel-Hamas war at a center of Black politics and culture.

Head of School Kristy Lundstrom sent the following statement to Mount Vernon parents:
Dear Mount Vernon Family,
I am aware that a screenshot is being circulated on social media and has caused concern and distress. The screenshot is a snapshot taken out of context from a classroom assignment from March 2024. We do not condone positive labels for Adolf Hitler. The intent of the assignment was an exploration of World War II designed to boost student knowledge of factual events and understand the manipulation of fear leveraged by Adolf Hitler in connection to the Treaty of Versailles. When leadership was made aware of how the assignment was written it was removed from the curriculum.
Immediately following this incident, I met with the School’s Chief of Inclusion, Diversity, Equity, and Action, Head of Middle School, and a concerned Rabbi and friend of the School who shared the perspective of some of our families and supported us in a thorough review of the assignment and community impact. We worked to understand the balance of holding a safe environment for students while doing the important work of sharing history to prevent deniers and to be clear about the atrocities committed. Adolf Hitler and the events of this time period are difficult and traumatic to discuss.
In this very heavy time, when our Jewish students and families have the added stress of world events, we want to be clear that The Mount Vernon School wholeheartedly denounces antisemitism.
Our commitment is to embrace a diverse community where every individual is seen, valued, and heard. Please know that we are here to care for and support your children. We have counselors, faith leaders, and school leaders who have been and will continue to be here to talk to, listen to, and lift up your children.
Copyright 2024 WRDW/WAGT. All rights reserved.

DHEC forces S.C. family to remove beach memorial to lost loved one

How inmates ran wild in uprising at Augusta youth detention center

3-year-old girl ‘crushed’ by birdbath in Warrenville

Shooting victim shows up to North Augusta emergency room

3rd suspect arrested in November murder near Paine College
Latest news.

Add these dates to your calendar as S.C. June primary approaches

‘This is what matters’: Ossoff to mark hospital, airport improvements in Augusta

News 12 FastCast: Quick headlines May 17

Wednesday night's MN House debate could impact end of session negotiations

Masters champion detained by police before start of PGA Championship

IMAGES
VIDEO
COMMENTS
Assignment statements may also appear as arguments to the alias, declare, typeset, export, readonly, and local builtin commands (declaration commands). When in POSIX mode (see Bash POSIX Mode), these builtins may appear in a command after one or more instances of the command builtin and retain these assignment statement properties.
Bash: let statement vs assignment. Ask Question Asked 10 years, 8 months ago. Modified 8 years ago. Viewed 79k times ... Making statements based on opinion; back them up with references or personal experience. To learn more, see our tips on writing great answers. Sign ...
Bash: let Statement vs. Assignment. 1. Overview. The built-in let command is part of the Bash and Korn shell fundamental software. Its main usage focuses on building variable assignments with basic arithmetic operations. In this article, we describe the typical usage syntax of the command. Thereupon, we show all meaningful properties that ...
The first line #!/bin/bash specifies the interpreter to use (/bin/bash) for executing the script.Then, three variables x, y, and z are assigned values 1, 2, and 3, respectively.The echo statements are used to print the values of each variable.Following that, two variables var1 and var2 are assigned values "Hello" and "World", respectively.The semicolon (;) separates the assignment ...
Here, we'll create five variables. The format is to type the name, the equals sign =, and the value. Note there isn't a space before or after the equals sign. Giving a variable a value is often referred to as assigning a value to the variable. We'll create four string variables and one numeric variable, my_name=Dave.
Variable Assignment. the assignment operator ( no space before and after) Do not confuse this with = and -eq, which test , rather than assign! Note that = can be either an assignment or a test operator, depending on context. Example 4-2. Plain Variable Assignment. #!/bin/bash. # Naked variables. echo.
Using the multiple variable assignment technique in Bash scripts can make our code look compact and give us the benefit of better performance, particularly when we want to assign multiple variables by the output of expensive command execution. For example, let's say we want to assign seven variables - the calendar week number, year, month ...
The importance of this type-checking lies in the operator's most common use—in conditional assignment statements. In this usage it appears as an expression on the right side of an assignment statement, as follows: ... a general ternary assignment doesn't exist in Bash. - zakmck. Apr 10, 2023 at 18:49. @zakmck i think you should check again ...
6.4 Bash Conditional Expressions. Conditional expressions are used by the [[ compound command (see Conditional Constructs ) and the test and [ builtin commands (see Bourne Shell Builtins ). The test and [ commands determine their behavior based on the number of arguments; see the descriptions of those commands for any other command-specific ...
3.2.5.2 Conditional Constructs. The syntax of the if command is: consequent-commands ; more-consequents ;] The test-commands list is executed, and if its return status is zero, the consequent-commands list is executed. If test-commands returns a non-zero status, each elif list is executed in turn, and if its exit status is zero, the ...
1st Value is. 2nd Value is. varName is varBar. varCmd is echo bar. Command is varBar=echo bar. ./testvars.sh: line 8: varBar=echo bar: command not found. 1st Value is. 2nd Value is. It looks like Bash is interpreting the whole thing as one command name (string) and not interpreting the = as an operator.
A case statement is far more readable than jamming it all into one line (which can end in catastrophe if the second command can fail, in this case, it is fine, but getting into that habit can be costly). ... If your assignment is numeric, you can use bash ternary operation: (( assign_condition ? value_when_true : value_when_false )) Share ...
3 min read. The bash case statement is generally used to simplify complex conditionals when there are multiple different choices. Using the case statement instead of nested if statements will help make your bash scripts more readable and easier to maintain. The Bash case statement has a concept similar to the Javascript or C switch statement.
Copy the script from above into an editor, save it as a file called "if-age.sh", and use the chmod command to make it executable. You'll need to do that with each of the scripts we discuss. chmod +x if-age.sh. Let's run our script. ./if-age.sh. Now we'll edit the file and use an age less than 21. customer_age=18.
fi. You can use all the if else statements in a single line like this: if [ $(whoami) = 'root' ]; then echo "root"; else echo "not root"; fi. You can copy and paste the above in terminal and see the result for yourself. Basically, you just add semicolons after the commands and then add the next if-else statement.
The if statement starts with the if keyword followed by the conditional expression and the then keyword. The statement ends with the fi keyword.. If the TEST-COMMAND evaluates to True, the STATEMENTS are executed. If the TEST-COMMAND returns False, nothing happens; the STATEMENTS are ignored.. Generally, it is always good practice to indent your code and separate code blocks with blank lines.
$ a=1; b=2; c=3 $ echo $(( max = a > b ? ( a > c ? a : c) : (b > c ? b : c) )) 3. In this case, the second and third operands or the main ternary expression are themselves ternary expressions.If a is greater than b, then a is compared to c and the larger of the two is returned. Otherwise, b is compared to c and the larger is returned. Numerically, since the first operand evaluates to zero ...
Washington, DC - (May 15, 2024) - CNN will host an election debate between President Joe Biden and former President Donald J. Trump on June 27, 2024 at 9pm ET from the crucial battleground ...
Chapter 5 of the Bash Cookbook by O'Reilly, discusses (at some length) the reasons for the requirement in a variable assignment that there be no spaces around the '=' sign. MYVAR="something" The explanation has something to do with distinguishing between the name of a command and a variable (where '=' may be a valid argument).
The behavior is documented in man bash in the "ENVIRONMENT" section: The environment for any simple command or function may be augmented temporarily by prefixing it with parameter assignments, as described above in PARAMETERS. These assignment statements affect only the envi‐ ronment seen by that command.
A screenshot posted on social media from a classroom assignment about Adolf Hitler and World War II at Mount Vernon School was taken out of context and caused distress for some families, a school ...
The assignment asked students to rate the attributes of the former Nazi dictator. The school now acknowledges this caused distress and the assignment has now been removed from the curriculum.
Georgia's parliament is set to pass a highly controversial so-called "foreign agents" bill that has triggered widespread protests across the former Soviet republic nestled in the Caucasus ...
Other colours, and why cameras see them better. Read more: What are auroras, and why do they come in different shapes and colours? Two experts explain. The electric rainbow of the aurora happens ...
bash integer variables. In addition to, dutCh, Vladimir and ghostdog74's corrects answers and because this question is regarding integer and tagged bash: Is there a way to do something like this. int a = (b == 5) ? c : d; There is a nice and proper way to work with integers under bash:
ksh, zsh, bash and yash also support some forms of array / list variables with variation in syntax for both assignment and expansion. ksh93, zsh and bash also have support for associative arrays with again variation in syntax between the 3. ksh93 also has compound variables and types, reminiscent of the objects and classes of object programming ...
Manicures are the cherry on top of any well-crafted look, and the stars at the 2024 Met Gala understood the assignment. "The Garden of Time," a short story by J.G. Ballard, served as the theme ...
Published: May. 16, 2024 at 5:13 AM PDT | Updated: moments ago. ATLANTA, Ga. (Atlanta News First) - A metro Atlanta school is responding to backlash to a controversial assignment. Parents are ...