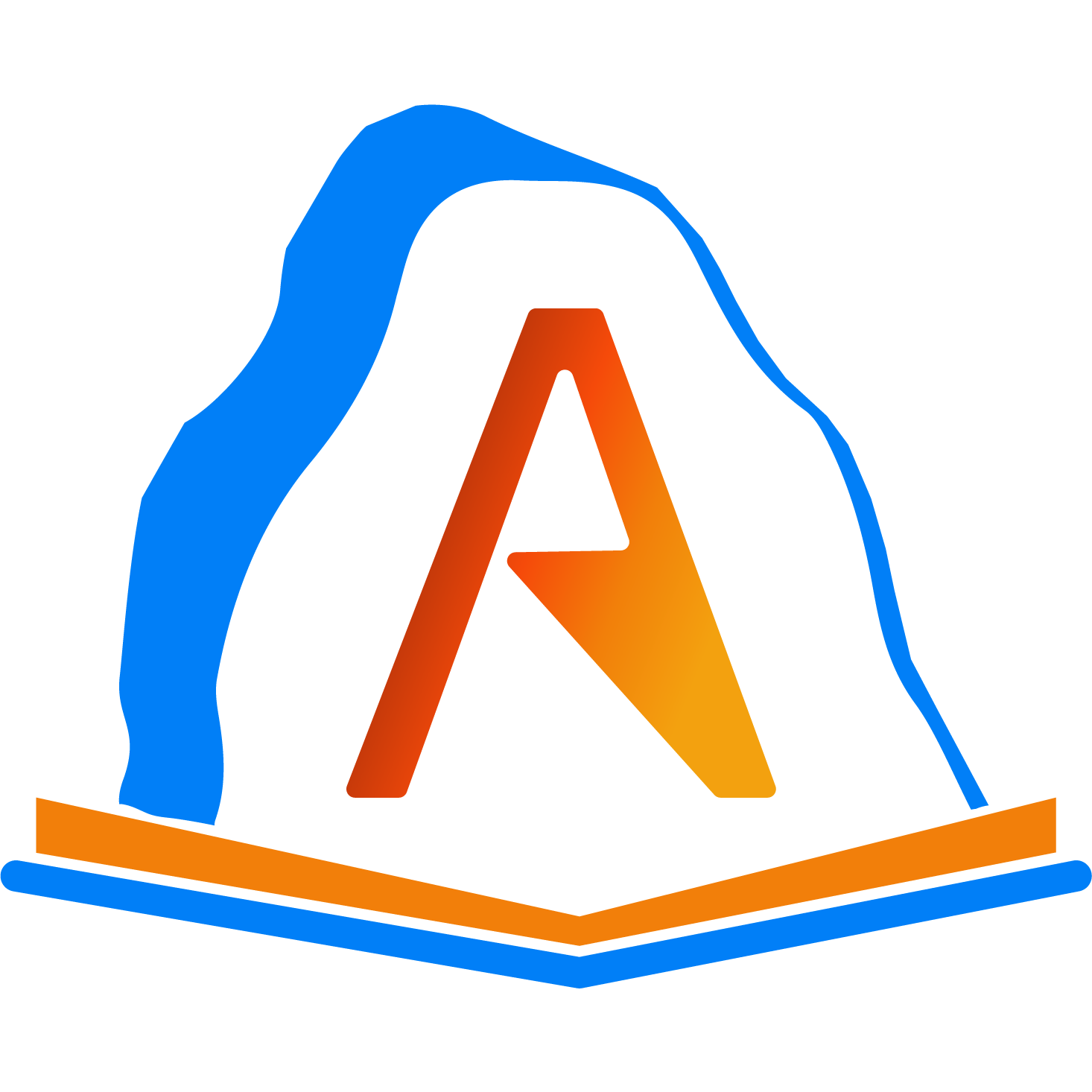
- Table of Contents
- Course Home
- Assignments
- Peer Instruction (Instructor)
- Peer Instruction (Student)
- Change Course
- Instructor's Page
- Progress Page
- Edit Profile
- Change Password
- Scratch ActiveCode
- Scratch Activecode
- Instructors Guide
- About Runestone
- Report A Problem
- Coding Practice
- Activecode Exercises
- Mixed Up Code Practice
- 6.1 Multiple assignment
- 6.2 Iteration
- 6.3 The while statement
- 6.5 Two-dimensional tables
- 6.6 Encapsulation and generalization
- 6.7 Functions
- 6.8 More encapsulation
- 6.9 Local variables
- 6.10 More generalization
- 6.11 Glossary
- 6.12 Multiple Choice Exercises
- 6.13 Mixed-Up Code Exercises
- 6.14 Coding Practice
- 6. Iteration" data-toggle="tooltip">
- 6.2. Iteration' data-toggle="tooltip" >

6.1. Multiple assignment ¶
I haven’t said much about it, but it is legal in C++ to make more than one assignment to the same variable. The effect of the second assignment is to replace the old value of the variable with a new value.
The active code below reassigns fred from 5 to 7 and prints both values out.
The output of this program is 57 , because the first time we print fred his value is 5, and the second time his value is 7.
The active code below reassigns fred from 5 to 7 without printing out the initial value.
However, if we do not print fred the first time, the output is only 7 because the value of fred is just 7 when it is printed.
This kind of multiple assignment is the reason I described variables as a container for values. When you assign a value to a variable, you change the contents of the container, as shown in the figure:
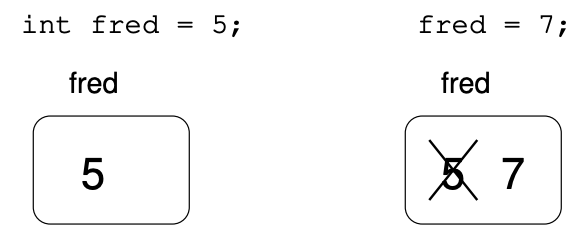
When there are multiple assignments to a variable, it is especially important to distinguish between an assignment statement and a statement of equality. Because C++ uses the = symbol for assignment, it is tempting to interpret a statement like a = b as a statement of equality. It is not!
An assignment statement uses a single = symbol. For example, x = 3 assigns the value of 3 to the variable x . On the other hand, an equality statement uses two = symbols. For example, x == 3 is a boolean that evaluates to true if x is equal to 3 and evaluates to false otherwise.
First of all, equality is commutative, and assignment is not. For example, in mathematics if \(a = 7\) then \(7 = a\) . But in C++ the statement a = 7; is legal, and 7 = a; is not.
Furthermore, in mathematics, a statement of equality is true for all time. If \(a = b\) now, then \(a\) will always equal \(b\) . In C++, an assignment statement can make two variables equal, but they don’t have to stay that way!
The third line changes the value of a but it does not change the value of b , and so they are no longer equal. In many programming languages an alternate symbol is used for assignment, such as <- or := , in order to avoid confusion.
Although multiple assignment is frequently useful, you should use it with caution. If the values of variables are changing constantly in different parts of the program, it can make the code difficult to read and debug.
- Checking if a is equal to b
- Assigning a to the value of b
- Setting the value of a to 4
Q-4: What will print?
- There are no spaces between the numbers.
- Remember, in C++ spaces must be printed.
- Carefully look at the values being assigned.
Q-5: What is the correct output?
- Remember that printing a boolean results in either 0 or 1.
- Is x equal to y?
- x is equal to y, so the output is 1.
C++ Tutorial
C++ functions, c++ classes, c++ examples, c++ declare multiple variables, declare many variables.
To declare more than one variable of the same type , use a comma-separated list:
One Value to Multiple Variables
You can also assign the same value to multiple variables in one line:

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.

When there are multiple assignments to a variable, it is especially important to distinguish between an assignment statement and a statement of equality. Because C++ uses the = symbol for assignment, it is tempting to interpret a statement like a = b as a statement of equality. It is not!
First of all, equality is commutative, and assignment is not. For example, in mathematics if a = 7 then 7 = a . But in C++ the statement a = 7; is legal, and 7 = a; is not.
Furthermore, in mathematics, a statement of equality is true for all time. If a = b now, then a will always equal b . In C++, an assignment statement can make two variables equal, but they don't have to stay that way!
The third line changes the value of a but it does not change the value of b , and so they are no longer equal. In many programming languages an alternate symbol is used for assignment, such as <- or := , in order to avoid confusion.
Although multiple assignment is frequently useful, you should use it with caution. If the values of variables are changing constantly in different parts of the program, it can make the code difficult to read and debug.

- C++ Language
- Ascii Codes
- Boolean Operations
- Numerical Bases
Introduction
Basics of c++.
- Structure of a program
- Variables and types
- Basic Input/Output
Program structure
- Statements and flow control
- Overloads and templates
- Name visibility
Compound data types
- Character sequences
- Dynamic memory
- Data structures
- Other data types
- Classes (I)
- Classes (II)
- Special members
- Friendship and inheritance
- Polymorphism
Other language features
- Type conversions
- Preprocessor directives
Standard library
- Input/output with files
Assignment operator (=)
Arithmetic operators ( +, -, *, /, % ), compound assignment (+=, -=, *=, /=, %=, >>=, <<=, &=, ^=, |=), increment and decrement (++, --), relational and comparison operators ( ==, =, >, <, >=, <= ), logical operators ( , &&, || ), conditional ternary operator ( ), comma operator ( , ), bitwise operators ( &, |, ^, ~, <<, >> ), explicit type casting operator, other operators, precedence of operators.

21.12 — Overloading the assignment operator
The copy assignment operator (operator=) is used to copy values from one object to another already existing object .
Related content
As of C++11, C++ also supports “Move assignment”. We discuss move assignment in lesson 22.3 -- Move constructors and move assignment .
Copy assignment vs Copy constructor
The purpose of the copy constructor and the copy assignment operator are almost equivalent -- both copy one object to another. However, the copy constructor initializes new objects, whereas the assignment operator replaces the contents of existing objects.
The difference between the copy constructor and the copy assignment operator causes a lot of confusion for new programmers, but it’s really not all that difficult. Summarizing:
- If a new object has to be created before the copying can occur, the copy constructor is used (note: this includes passing or returning objects by value).
- If a new object does not have to be created before the copying can occur, the assignment operator is used.
Overloading the assignment operator
Overloading the copy assignment operator (operator=) is fairly straightforward, with one specific caveat that we’ll get to. The copy assignment operator must be overloaded as a member function.
This prints:
This should all be pretty straightforward by now. Our overloaded operator= returns *this, so that we can chain multiple assignments together:
Issues due to self-assignment
Here’s where things start to get a little more interesting. C++ allows self-assignment:
This will call f1.operator=(f1), and under the simplistic implementation above, all of the members will be assigned to themselves. In this particular example, the self-assignment causes each member to be assigned to itself, which has no overall impact, other than wasting time. In most cases, a self-assignment doesn’t need to do anything at all!
However, in cases where an assignment operator needs to dynamically assign memory, self-assignment can actually be dangerous:
First, run the program as it is. You’ll see that the program prints “Alex” as it should.
Now run the following program:
You’ll probably get garbage output. What happened?
Consider what happens in the overloaded operator= when the implicit object AND the passed in parameter (str) are both variable alex. In this case, m_data is the same as str.m_data. The first thing that happens is that the function checks to see if the implicit object already has a string. If so, it needs to delete it, so we don’t end up with a memory leak. In this case, m_data is allocated, so the function deletes m_data. But because str is the same as *this, the string that we wanted to copy has been deleted and m_data (and str.m_data) are dangling.
Later on, we allocate new memory to m_data (and str.m_data). So when we subsequently copy the data from str.m_data into m_data, we’re copying garbage, because str.m_data was never initialized.
Detecting and handling self-assignment
Fortunately, we can detect when self-assignment occurs. Here’s an updated implementation of our overloaded operator= for the MyString class:
By checking if the address of our implicit object is the same as the address of the object being passed in as a parameter, we can have our assignment operator just return immediately without doing any other work.
Because this is just a pointer comparison, it should be fast, and does not require operator== to be overloaded.
When not to handle self-assignment
Typically the self-assignment check is skipped for copy constructors. Because the object being copy constructed is newly created, the only case where the newly created object can be equal to the object being copied is when you try to initialize a newly defined object with itself:
In such cases, your compiler should warn you that c is an uninitialized variable.
Second, the self-assignment check may be omitted in classes that can naturally handle self-assignment. Consider this Fraction class assignment operator that has a self-assignment guard:
If the self-assignment guard did not exist, this function would still operate correctly during a self-assignment (because all of the operations done by the function can handle self-assignment properly).
Because self-assignment is a rare event, some prominent C++ gurus recommend omitting the self-assignment guard even in classes that would benefit from it. We do not recommend this, as we believe it’s a better practice to code defensively and then selectively optimize later.
The copy and swap idiom
A better way to handle self-assignment issues is via what’s called the copy and swap idiom. There’s a great writeup of how this idiom works on Stack Overflow .
The implicit copy assignment operator
Unlike other operators, the compiler will provide an implicit public copy assignment operator for your class if you do not provide a user-defined one. This assignment operator does memberwise assignment (which is essentially the same as the memberwise initialization that default copy constructors do).
Just like other constructors and operators, you can prevent assignments from being made by making your copy assignment operator private or using the delete keyword:
Note that if your class has const members, the compiler will instead define the implicit operator= as deleted. This is because const members can’t be assigned, so the compiler will assume your class should not be assignable.
If you want a class with const members to be assignable (for all members that aren’t const), you will need to explicitly overload operator= and manually assign each non-const member.
cppreference.com
Assignment operators.
Assignment and compound assignment operators are binary operators that modify the variable to their left using the value to their right.
[ edit ] Simple assignment
The simple assignment operator expressions have the form
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs .
Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non-lvalue (so that expressions such as ( a = b ) = c are invalid).
rhs and lhs must satisfy one of the following:
- both lhs and rhs have compatible struct or union type, or..
- rhs must be implicitly convertible to lhs , which implies
- both lhs and rhs have arithmetic types , in which case lhs may be volatile -qualified or atomic (since C11)
- both lhs and rhs have pointer to compatible (ignoring qualifiers) types, or one of the pointers is a pointer to void, and the conversion would not add qualifiers to the pointed-to type. lhs may be volatile or restrict (since C99) -qualified or atomic (since C11) .
- lhs is a (possibly qualified or atomic (since C11) ) pointer and rhs is a null pointer constant such as NULL or a nullptr_t value (since C23)
[ edit ] Notes
If rhs and lhs overlap in memory (e.g. they are members of the same union), the behavior is undefined unless the overlap is exact and the types are compatible .
Although arrays are not assignable, an array wrapped in a struct is assignable to another object of the same (or compatible) struct type.
The side effect of updating lhs is sequenced after the value computations, but not the side effects of lhs and rhs themselves and the evaluations of the operands are, as usual, unsequenced relative to each other (so the expressions such as i = ++ i ; are undefined)
Assignment strips extra range and precision from floating-point expressions (see FLT_EVAL_METHOD ).
In C++, assignment operators are lvalue expressions, not so in C.
[ edit ] Compound assignment
The compound assignment operator expressions have the form
The expression lhs @= rhs is exactly the same as lhs = lhs @ ( rhs ) , except that lhs is evaluated only once.
[ edit ] References
- C17 standard (ISO/IEC 9899:2018):
- 6.5.16 Assignment operators (p: 72-73)
- C11 standard (ISO/IEC 9899:2011):
- 6.5.16 Assignment operators (p: 101-104)
- C99 standard (ISO/IEC 9899:1999):
- 6.5.16 Assignment operators (p: 91-93)
- C89/C90 standard (ISO/IEC 9899:1990):
- 3.3.16 Assignment operators
[ edit ] See Also
Operator precedence
[ edit ] See also
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 19 August 2022, at 08:36.
- This page has been accessed 54,567 times.
- Privacy policy
- About cppreference.com
- Disclaimers

C++ Operators Introduction
C++ multiple assignments, c++ arithmetic operators, c++ relational operators, c++ logical operators, c++ relational logical precedence, c++ increment and decrement, c++ assignment operator, c++ compound assignment, c++ conditional operator, c++ logical operators in if, c++ bitwise operators introduction, c++ bitwise shift operators, c++ bitwise operators usage, c++ comma operator, c++ operator precedence, c++ sizeof operator, c++ operators new and delete, c++ allocating arrays, c++ memory initializing, c++ malloc() and free(), c++ operator exercise 1.
C++ allows a very convenient method of assigning many variables the same value: using multiple assignments in a single statement.
For example, this fragment assigns count, incr, and index the value 10:
In professionally written programs, you will often see variables assigned a common value using this format.
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
- Solve Coding Problems
- Bitmask in C++
- C++ Variable Templates
- vTable And vPtr in C++
- Address Operator & in C
- Macros In C++
- Variable Shadowing in C++
- Unique_ptr in C++
- Pass By Reference In C
- C++ Program For Sentinel Linear Search
- Partial Template Specialization in C++
- Difference Between Constant and Literals
- Concurrency in C++
- String Tokenization in C
- Decision Making in C++
- auto_ptr in C++
- shared_ptr in C++
- Mutex in C++
- C++ 20 - <semaphore> Header
- Compound Statements in C++
Assignment Operators In C++
In C++, the assignment operator forms the backbone of many algorithms and computational processes by performing a simple operation like assigning a value to a variable. It is denoted by equal sign ( = ) and provides one of the most basic operations in any programming language that is used to assign some value to the variables in C++ or in other words, it is used to store some kind of information.
The right-hand side value will be assigned to the variable on the left-hand side. The variable and the value should be of the same data type.
The value can be a literal or another variable of the same data type.
Compound Assignment Operators
In C++, the assignment operator can be combined into a single operator with some other operators to perform a combination of two operations in one single statement. These operators are called Compound Assignment Operators. There are 10 compound assignment operators in C++:
- Addition Assignment Operator ( += )
- Subtraction Assignment Operator ( -= )
- Multiplication Assignment Operator ( *= )
- Division Assignment Operator ( /= )
- Modulus Assignment Operator ( %= )
- Bitwise AND Assignment Operator ( &= )
- Bitwise OR Assignment Operator ( |= )
- Bitwise XOR Assignment Operator ( ^= )
- Left Shift Assignment Operator ( <<= )
- Right Shift Assignment Operator ( >>= )
Lets see each of them in detail.
1. Addition Assignment Operator (+=)
In C++, the addition assignment operator (+=) combines the addition operation with the variable assignment allowing you to increment the value of variable by a specified expression in a concise and efficient way.
This above expression is equivalent to the expression:
2. Subtraction Assignment Operator (-=)
The subtraction assignment operator (-=) in C++ enables you to update the value of the variable by subtracting another value from it. This operator is especially useful when you need to perform subtraction and store the result back in the same variable.
3. Multiplication Assignment Operator (*=)
In C++, the multiplication assignment operator (*=) is used to update the value of the variable by multiplying it with another value.
4. Division Assignment Operator (/=)
The division assignment operator divides the variable on the left by the value on the right and assigns the result to the variable on the left.
5. Modulus Assignment Operator (%=)
The modulus assignment operator calculates the remainder when the variable on the left is divided by the value or variable on the right and assigns the result to the variable on the left.
6. Bitwise AND Assignment Operator (&=)
This operator performs a bitwise AND between the variable on the left and the value on the right and assigns the result to the variable on the left.
7. Bitwise OR Assignment Operator (|=)
The bitwise OR assignment operator performs a bitwise OR between the variable on the left and the value or variable on the right and assigns the result to the variable on the left.
8. Bitwise XOR Assignment Operator (^=)
The bitwise XOR assignment operator performs a bitwise XOR between the variable on the left and the value or variable on the right and assigns the result to the variable on the left.
9. Left Shift Assignment Operator (<<=)
The left shift assignment operator shifts the bits of the variable on the left to left by the number of positions specified on the right and assigns the result to the variable on the left.
10. Right Shift Assignment Operator (>>=)
The right shift assignment operator shifts the bits of the variable on the left to the right by a number of positions specified on the right and assigns the result to the variable on the left.
Also, it is important to note that all of the above operators can be overloaded for custom operations with user-defined data types to perform the operations we want.

Please Login to comment...
- Geeks Premier League 2023
- Geeks Premier League
- WhatsApp To Launch New App Lock Feature
- Node.js 21 is here: What’s new
- Zoom: World’s Most Innovative Companies of 2024
- 10 Best Skillshare Alternatives in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Learn C++ practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c++ interactively, c++ introduction.
- C++ Variables and Literals
- C++ Data Types
- C++ Basic I/O
- C++ Type Conversion
- C++ Operators
- C++ Comments
C++ Flow Control
- C++ if...else
- C++ for Loop
- C++ do...while Loop
- C++ continue
- C++ switch Statement
- C++ goto Statement
- C++ Functions
- C++ Function Types
- C++ Function Overloading
- C++ Default Argument
- C++ Storage Class
- C++ Recursion
- C++ Return Reference
C++ Arrays & String
- Multidimensional Arrays
- C++ Function and Array
- C++ Structures
- Structure and Function
- C++ Pointers to Structure
- C++ Enumeration
C++ Object & Class
- C++ Objects and Class
- C++ Constructors
- C++ Objects & Function
- C++ Operator Overloading
- C++ Pointers
- C++ Pointers and Arrays
- C++ Pointers and Functions
- C++ Memory Management
C++ Inheritance
- Inheritance Access Control
C++ Function Overriding
- Inheritance Types
- C++ Friend Function
- C++ Virtual Function
- C++ Templates
C++ Tutorials
C++ Public, Protected and Private Inheritance
- C++ Abstract Class and Pure Virtual Function
C++ Virtual Functions
- C++ Polymorphism
C++ Multiple, Multilevel and Hierarchical Inheritance
Inheritance is one of the core feature of an object-oriented programming language. It allows software developers to derive a new class from the existing class. The derived class inherits the features of the base class (existing class).
There are various models of inheritance in C++ programming.
- C++ Multilevel Inheritance
In C++ programming, not only you can derive a class from the base class but you can also derive a class from the derived class. This form of inheritance is known as multilevel inheritance.
Here, class B is derived from the base class A and the class C is derived from the derived class B .
Example 1: C++ Multilevel Inheritance
In this program, class C is derived from class B (which is derived from base class A ).
The obj object of class C is defined in the main() function.
When the display() function is called, display() in class A is executed. It's because there is no display() function in class C and class B .
The compiler first looks for the display() function in class C . Since the function doesn't exist there, it looks for the function in class B (as C is derived from B ).
The function also doesn't exist in class B , so the compiler looks for it in class A (as B is derived from A ).
If display() function exists in C , the compiler overrides display() of class A (because of member function overriding ).
- C++ Multiple Inheritance
In C++ programming, a class can be derived from more than one parent. For example, A class Bat is derived from base classes Mammal and WingedAnimal . It makes sense because bat is a mammal as well as a winged animal.

Example 2: Multiple Inheritance in C++ Programming
- Ambiguity in Multiple Inheritance
The most obvious problem with multiple inheritance occurs during function overriding.
Suppose, two base classes have a same function which is not overridden in derived class.
If you try to call the function using the object of the derived class, compiler shows error. It's because compiler doesn't know which function to call. For example,
This problem can be solved using the scope resolution function to specify which function to class either base1 or base2
- C++ Hierarchical Inheritance
If more than one class is inherited from the base class, it's known as hierarchical inheritance . In hierarchical inheritance, all features that are common in child classes are included in the base class.
For example, Physics, Chemistry, Biology are derived from Science class. Similarly, Dog, Cat, Horse are derived from Animal class.
Syntax of Hierarchical Inheritance
Example 3: hierarchical inheritance in c++ programming.
Here, both the Dog and Cat classes are derived from the Animal class. As such, both the derived classes can access the info() function belonging to the Animal class.
Table of Contents
- Introduction
- Example: C++ Multilevel Inheritance
- Example: Multiple Inheritance in C++ Programming
Sorry about that.
Related Tutorials
C++ Tutorial
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Assignment operators
- 8 contributors
expression assignment-operator expression
assignment-operator : one of = *= /= %= += -= <<= >>= &= ^= |=
Assignment operators store a value in the object specified by the left operand. There are two kinds of assignment operations:
simple assignment , in which the value of the second operand is stored in the object specified by the first operand.
compound assignment , in which an arithmetic, shift, or bitwise operation is performed before storing the result.
All assignment operators in the following table except the = operator are compound assignment operators.
Assignment operators table
Operator keywords.
Three of the compound assignment operators have keyword equivalents. They are:
C++ specifies these operator keywords as alternative spellings for the compound assignment operators. In C, the alternative spellings are provided as macros in the <iso646.h> header. In C++, the alternative spellings are keywords; use of <iso646.h> or the C++ equivalent <ciso646> is deprecated. In Microsoft C++, the /permissive- or /Za compiler option is required to enable the alternative spelling.
Simple assignment
The simple assignment operator ( = ) causes the value of the second operand to be stored in the object specified by the first operand. If both objects are of arithmetic types, the right operand is converted to the type of the left, before storing the value.
Objects of const and volatile types can be assigned to l-values of types that are only volatile , or that aren't const or volatile .
Assignment to objects of class type ( struct , union , and class types) is performed by a function named operator= . The default behavior of this operator function is to perform a member-wise copy assignment of the object's non-static data members and direct base classes; however, this behavior can be modified using overloaded operators. For more information, see Operator overloading . Class types can also have copy assignment and move assignment operators. For more information, see Copy constructors and copy assignment operators and Move constructors and move assignment operators .
An object of any unambiguously derived class from a given base class can be assigned to an object of the base class. The reverse isn't true because there's an implicit conversion from derived class to base class, but not from base class to derived class. For example:
Assignments to reference types behave as if the assignment were being made to the object to which the reference points.
For class-type objects, assignment is different from initialization. To illustrate how different assignment and initialization can be, consider the code
The preceding code shows an initializer; it calls the constructor for UserType2 that takes an argument of type UserType1 . Given the code
the assignment statement
can have one of the following effects:
Call the function operator= for UserType2 , provided operator= is provided with a UserType1 argument.
Call the explicit conversion function UserType1::operator UserType2 , if such a function exists.
Call a constructor UserType2::UserType2 , provided such a constructor exists, that takes a UserType1 argument and copies the result.
Compound assignment
The compound assignment operators are shown in the Assignment operators table . These operators have the form e1 op = e2 , where e1 is a non- const modifiable l-value and e2 is:
an arithmetic type
a pointer, if op is + or -
a type for which there exists a matching operator *op*= overload for the type of e1
The built-in e1 op = e2 form behaves as e1 = e1 op e2 , but e1 is evaluated only once.
Compound assignment to an enumerated type generates an error message. If the left operand is of a pointer type, the right operand must be of a pointer type, or it must be a constant expression that evaluates to 0. When the left operand is of an integral type, the right operand must not be of a pointer type.
Result of built-in assignment operators
The built-in assignment operators return the value of the object specified by the left operand after the assignment (and the arithmetic/logical operation in the case of compound assignment operators). The resultant type is the type of the left operand. The result of an assignment expression is always an l-value. These operators have right-to-left associativity. The left operand must be a modifiable l-value.
In ANSI C, the result of an assignment expression isn't an l-value. That means the legal C++ expression (a += b) += c isn't allowed in C.
Expressions with binary operators C++ built-in operators, precedence, and associativity C assignment operators
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Error: multiple assignment operators specified. #245
AIVASteph commented May 26, 2021 • edited
wjwwood commented Jun 10, 2021
Sorry, something went wrong.
No branches or pull requests
- FanNation FanNation FanNation
- SI.COM SI.COM SI.COM
- SI Swimsuit SI Swimsuit SI Swimsuit
- SI Sportsbook SI Sportsbook SI Sportsbook
- SI Tickets SI Tickets SI Tickets
- SI Showcase SI Showcase SI Showcase
- SI Resorts SI Resorts SI Resorts
Lakers News: LA Players Have Confidence in Role Player's Ability to Guard Tough Assignments
- Author: Matt Levine
In this story:
The Los Angeles Lakers season has been one of the stranger in recent years. Some nights they look like a juggernaut team, while on others, they feel like they could lose to the worst team in the NBA.
It has been a frustrating experience all season long, but the team has seemingly started to play much better of late. With the postseason coming up in a few weeks, Los Angeles is looking to continue their winning ways to propel them forward.
Within this run has been strong play from the star players on the team. But they haven't done it alone and multiple role players have stepped up to help. One of which has been forward Rui Hachimura, who has helped take this team to a new level.
Both on the offensive and defensive end, Hachimura gives the Lakers more length and flexibility to work with . Star Anthony Davis reiterated that Hachimura has the confidence of the rest of the team to take on tough assignments on defense, such as the opposing teams' star players.
“Sometimes, he guards (Nikola) Jokić. He guarded Giannis (Antetokounmpo) some when I wasn’t on him. (Devin Booker). He guarded (Kevin Durant) before,” Davis said. “So we have a lot of confidence in Rui on the defensive end. He’s shown that he can handle those matchups.”
The ability to switch the physical Hachimura onto some of the better players in the league such as Nikola Jokic or Kevin Durant gives the Lakers rotation versatility. He allows someone like Davis to focus more on protecting the rim, rather than having to step out to guard some of these players on the perimeter.
Hachimura isn't the perfect player, but he does vastly help this club. He can stretch the floor on offense as well, giving them a dual-threat each time he is on the court.
If the Lakers are going to continue to push forward, they will need contributions from players like Hachimura each night. They have a long road ahead of them, but the goal remains the same for this team.
It's championship or bust for Los Angeles, no matter where they end up in the standings or who they face off against.
Latest Lakers News
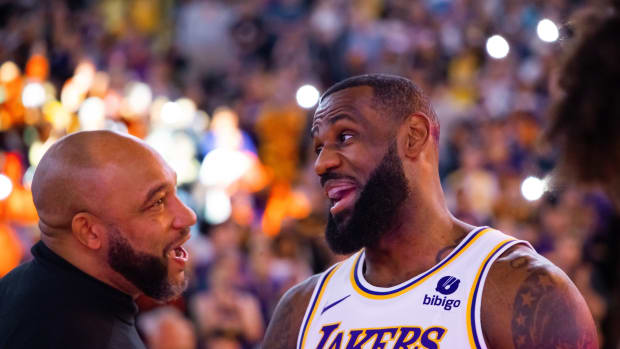
Lakers News: Darvin Ham Credits LA Star For Setting Tone Entering Crucial Stretch
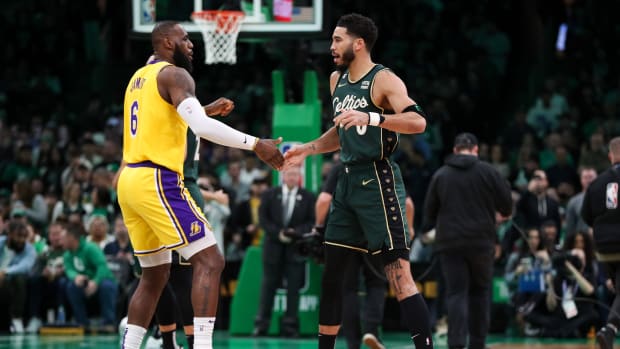
LeBron James' Strong Jayson Tatum-Nikola Jokic Statement
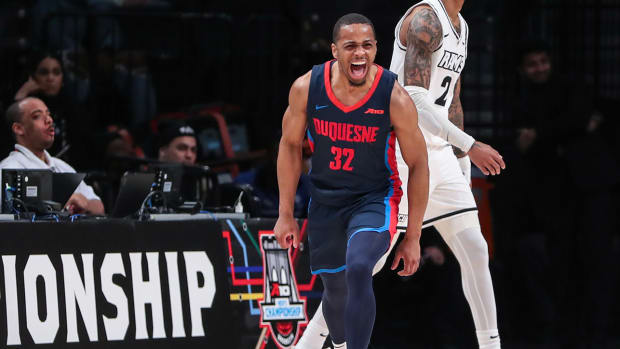
LeBron James Had Epic Gift for Duquesne Basketball Team Ahead of NCAA Tournament
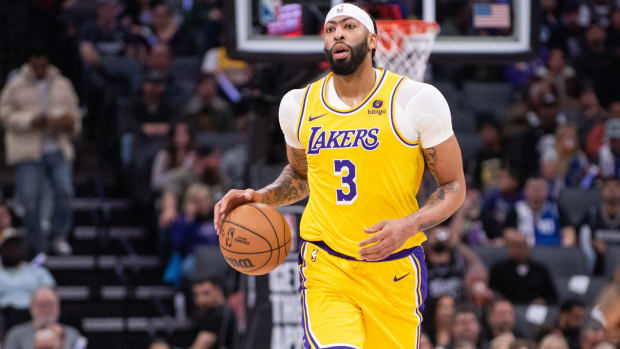
Lakers News: Anthony Davis Decided Not to Wear Protection Over Eyes Despite Risks
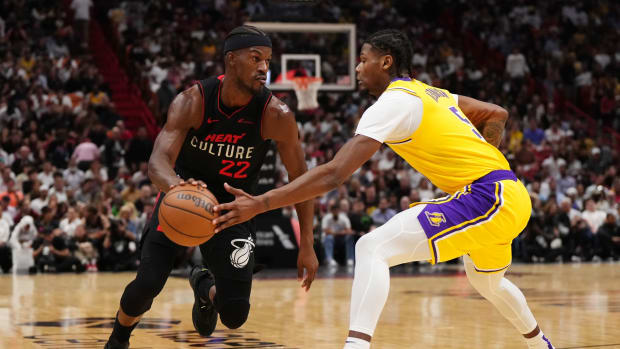
Lakers Injury Report: LA Given Huge Update on Crucial Role Player's Status For Matchup vs 76ers

IMAGES
VIDEO
COMMENTS
sample1 = sample2 = 0; some compilers will produce an assembly slightly faster in comparison to 2 assignments: sample1 = 0; sample2 = 0; specially if you are initializing to a non-zero value. Because, the multiple assignment translates to: sample2 = 0; sample1 = sample2; So instead of 2 initializations you do only one and one copy.
6.1. Multiple assignment ¶. I haven't said much about it, but it is legal in C++ to make more than one assignment to the same variable. The effect of the second assignment is to replace the old value of the variable with a new value. The active code below reassigns fred from 5 to 7 and prints both values out. The output of this program is 57 ...
for assignments to class type objects, the right operand could be an initializer list only when the assignment is defined by a user-defined assignment operator. removed user-defined assignment constraint. CWG 1538. C++11. E1 ={E2} was equivalent to E1 = T(E2) ( T is the type of E1 ), this introduced a C-style cast. it is equivalent to E1 = T{E2}
In this C++ tutorial video, you will learn about combined assignment operators and multiple assignment techniques. Discover how to efficiently perform operat...
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
Let's talk about multiple and combined assignment. Multiple assignment lets you squish more than one assignment statements into a single statement.The combin...
This kind of multiple assignment is the reason I described variables as a container for values. When you assign a value to a variable, you change the contents of the container, as shown in the figure: When there are multiple assignments to a variable, it is especially important to distinguish between an assignment statement and a statement of equality.
In the previous lesson (1.3 -- Introduction to objects and variables), we covered how to define a variable that we can use to store values.In this lesson, we'll explore how to actually put values into variables and use those values. As a reminder, here's a short snippet that first allocates a single integer variable named x, then allocates two more integer variables named y and z:
Packing multiple operations and/or statements into the same line is straightforward - both you and Xerzi have come up with ways. I think what the OP is looking for is something subtly different - a syntax construct to apply the same operation to multiple variables, concisely in one statement. I don't know of any such syntax in standard C++.
Dec 31, 2012 at 21:39. 1. It may be considered bad style because it's a bit more taxing to read (at 2am when you're debugging to a deadline) than three simple assignments. On the other hand, one line is less than three, so it can be argued both ways. - Omri Barel. Dec 31, 2012 at 21:40.
Assignment operator (=) The assignment operator assigns a value to a variable. 1: ... A single expression may have multiple operators. For example: 1: x = 5 + 7 % 2; In C++, the above expression always assigns 6 to variable x, because the % operator has a higher precedence than the + operator, and is always evaluated before. Parts of the ...
Overloading the assignment operator. Overloading the copy assignment operator (operator=) is fairly straightforward, with one specific caveat that we'll get to. The copy assignment operator must be overloaded as a member function. #include <cassert> #include <iostream> class Fraction { private: int m_numerator { 0 }; int m_denominator { 1 ...
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs . Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non ...
C++ Multiple Assignments. C++ allows a very convenient method of assigning many variables the same value: using multiple assignments in a single statement. For example, this fragment assigns count, incr, and index the value 10: In professionally written programs, you will often see variables assigned a common value using this format.
In C++, the assignment operator can be combined into a single operator with some other operators to perform a combination of two operations in one single statement. These operators are called Compound Assignment Operators. There are 10 compound assignment operators in C++: Addition Assignment Operator ( += ) Subtraction Assignment Operator ...
It does nothing. The comma operator makes it return the value of a (the right most operand). Because assignment binds tighter, b = b is in parens. The proper way doing this is just. std::swap(a, b); Boost includes a tuple class with which you can do. tie(a, b) = make_tuple(b, a);
C++ Multiple, Multilevel and Hierarchical Inheritance. Inheritance is one of the core feature of an object-oriented programming language. It allows software developers to derive a new class from the existing class. The derived class inherits the features of the base class (existing class). There are various models of inheritance in C++ programming.
The built-in assignment operators return the value of the object specified by the left operand after the assignment (and the arithmetic/logical operation in the case of compound assignment operators). The resultant type is the type of the left operand. The result of an assignment expression is always an l-value.
Forgive me if this is a noob question, but I haven't used C++ much in the last 9 or so years so I've forgotten a lot of it's nuances. I'm trying to include a built version of this library into UE4. I've successfully downloaded and built ...
so when you initialize an array, you can assign multiple values to it in one spot: int array [] = {1,3,34,5,6} but what if the array is already initialized and I want to completely replace the values of the elements in that array in one line. so .
Star Anthony Davis reiterated that Hachimura has the confidence of the rest of the team to take on tough assignments on defense, such as the opposing teams' star players. "Sometimes, he guards ...
You can simply place a forward declaration of your second () function in your main.cpp above main (). If your second.cpp has more than one function and you want all of it in main (), put all the forward declarations of your functions in second.cpp into a header file and #include it in main.cpp. Like this-. Second.h: